How to Solve Cloudflare with Puppeteer
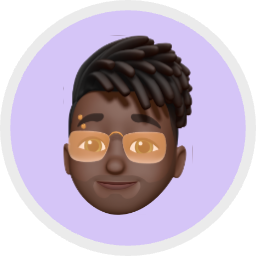
Lucas Mitchell
Automation Engineer
26-Aug-2024
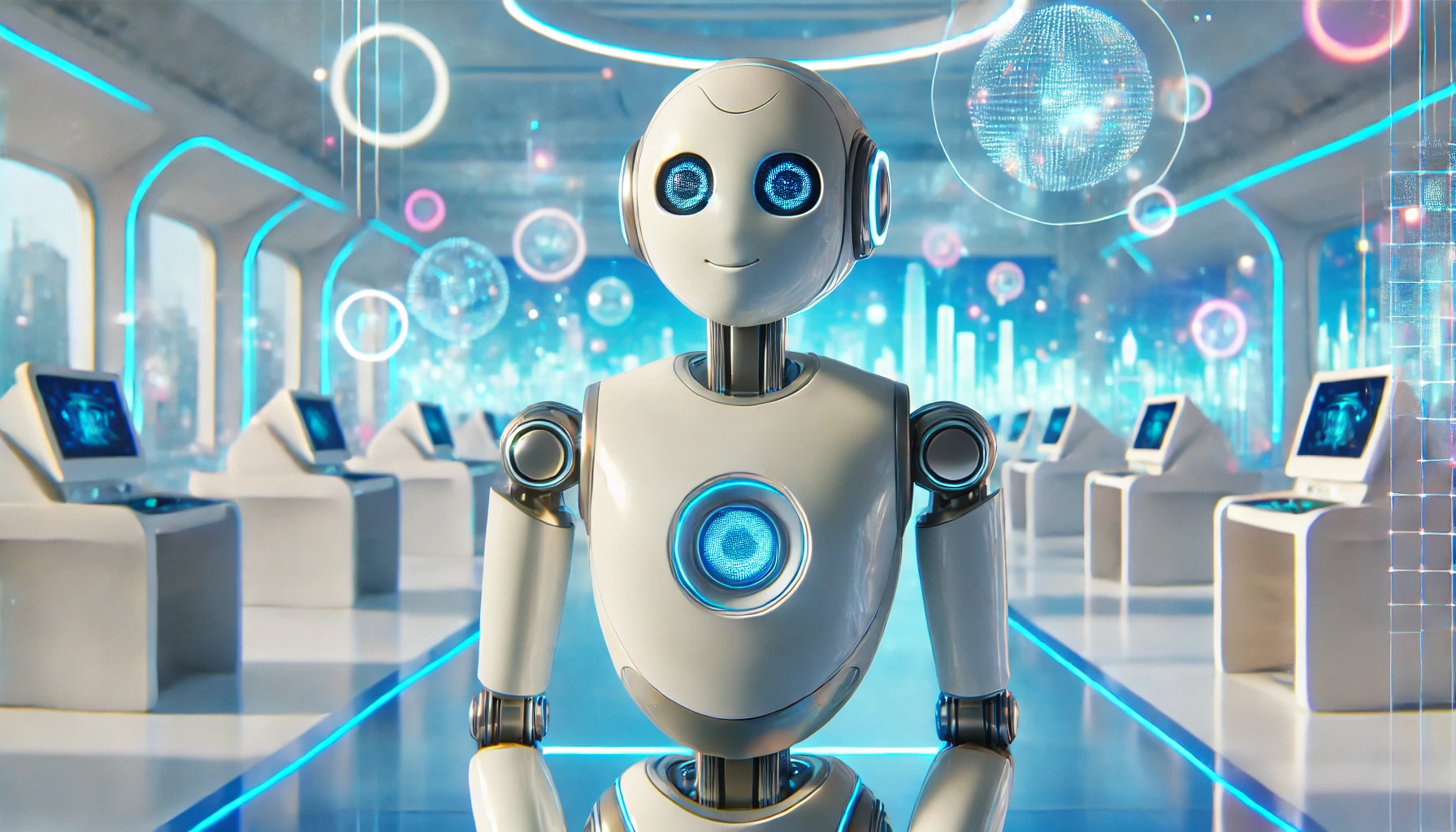
How to Solve Cloudflare with Puppeteer
Introduction
Cloudflare is a powerful service that provides security and performance enhancements for websites. It protects sites from a range of threats, including DDoS attacks and malicious bots, by implementing various security mechanisms. While these protections are beneficial for website owners, they can pose significant challenges for developers involved in web scraping and automation. Cloudflare’s defenses often include CAPTCHAs, JavaScript challenges, and browser checks, all designed to block automated scripts. For those using tools like Puppeteer to automate tasks, these barriers can be a significant obstacle. In this guide, we’ll walk through how to use Puppeteer to effectively navigate and solve Cloudflare's protections, enabling you to continue your automation projects without disruption.
Step-by-Step Guide to Using Puppeteer to Solve Cloudflare
Step 1: Setting Up Puppeteer
To begin, you'll need to set up Puppeteer, a Node.js library that offers a high-level API to control Chrome or Chromium. This tool is widely used for automating tasks, testing, and scraping websites.
Start by installing Puppeteer using npm:
bash
npm install puppeteer
Once installed, you can write a simple script to launch a browser instance and navigate to a Cloudflare-protected website:
javascript
const puppeteer = require('puppeteer');
(async () => {
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
await page.goto('https://example.com'); // Replace with your target URL
await page.screenshot({ path: 'before-cf.png' });
// Additional steps to handle Cloudflare's protections will follow
await browser.close();
})();
This script launches a browser, navigates to the specified URL, and takes a screenshot. However, simply visiting the site might trigger Cloudflare's security checks, so additional steps are necessary to handle them.
Step 2: Handling Cloudflare’s JavaScript Challenges
Cloudflare often uses JavaScript challenges to verify that the request is coming from a legitimate browser. These challenges typically involve running JavaScript that takes a few seconds to complete. Puppeteer can easily handle these checks by waiting for the necessary scripts to execute:
javascript
await page.waitForTimeout(10000); // Wait 10 seconds for Cloudflare's verification
await page.screenshot({ path: 'after-cf.png' });
This approach works for basic checks, but if Cloudflare deploys more sophisticated challenges, such as CAPTCHAs, you'll need a more advanced solution. This is where CapSolver comes into play.
CapSolver Integration: Enhancing Puppeteer to Bypass Cloudflare
CapSolver is a service designed to solve CAPTCHAs and other similar challenges automatically, which is particularly useful when dealing with Cloudflare’s advanced protections. By integrating CapSolver into your Puppeteer script, you can automate the resolution of these challenges, allowing your script to continue running without interruption.
Here’s how you can integrate CapSolver with Puppeteer:
javascript
const puppeteer = require('puppeteer');
const axios = require('axios');
const clientKey = 'your-client-key-here'; // Replace with your CapSolver client key
const websiteURL = 'https://example.com'; // Replace with your target website URL
const websiteKey = 'your-website-key-here'; // Replace with the website key provided by CapSolver
async function createTask() {
const response = await axios.post('https://api.capsolver.com/createTask', {
clientKey: clientKey,
task: {
type: "AntiTurnstileTaskProxyLess",
websiteURL: websiteURL,
websiteKey: websiteKey
}
}, {
headers: {
'Content-Type': 'application/json',
'Pragma': 'no-cache'
}
});
return response.data.taskId;
}
async function getTaskResult(taskId) {
console.log(taskId);
let response;
while (true) {
response = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: clientKey,
taskId: taskId
}, {
headers: {
'Content-Type': 'application/json'
}
});
if (response.data.status === 'ready') {
return response.data.solution;
}
console.log('Status not ready, checking again in 5 seconds...');
await new Promise(resolve => setTimeout(resolve, 5000));
}
}
(async () => {
const taskId = await createTask();
const result = await getTaskResult(taskId);
console.log(result);
let solution = result.token;
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
await page.goto(websiteURL);
await page.waitForSelector('input[name="cf-turnstile-response"]');
await page.evaluate(solution => {
document.querySelector('input[name="cf-turnstile-response"]').value = solution;
}, solution);
await page.screenshot({ path: 'example.png' });
})();
In this script:
- createTask(): Sends a request to CapSolver to solve the CAPTCHA for the specified website.
- getTaskResult(): Continuously checks the status of the CAPTCHA-solving task until CapSolver provides a solution.
- The Puppeteer script then uses this solution to bypass the CAPTCHA and continue interacting with the website.
By integrating CapSolver, you enhance Puppeteer's ability to bypass Cloudflare's protections, ensuring your automation tasks proceed without any manual intervention.
Conclusion
Navigating Cloudflare's security measures can be a significant challenge for developers and data engineers working on automation and web scraping tasks. While Puppeteer provides the tools needed to handle basic challenges, integrating CapSolver allows you to overcome more complex obstacles like CAPTCHAs seamlessly. This combination ensures that your scripts run smoothly, even on sites protected by Cloudflare.
To get started with CapSolver and improve the efficiency of your automation tasks, make sure to use our bonus code WEBS for added value. With the right tools and strategies, you can navigate Cloudflare's defenses and keep your projects on track.
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
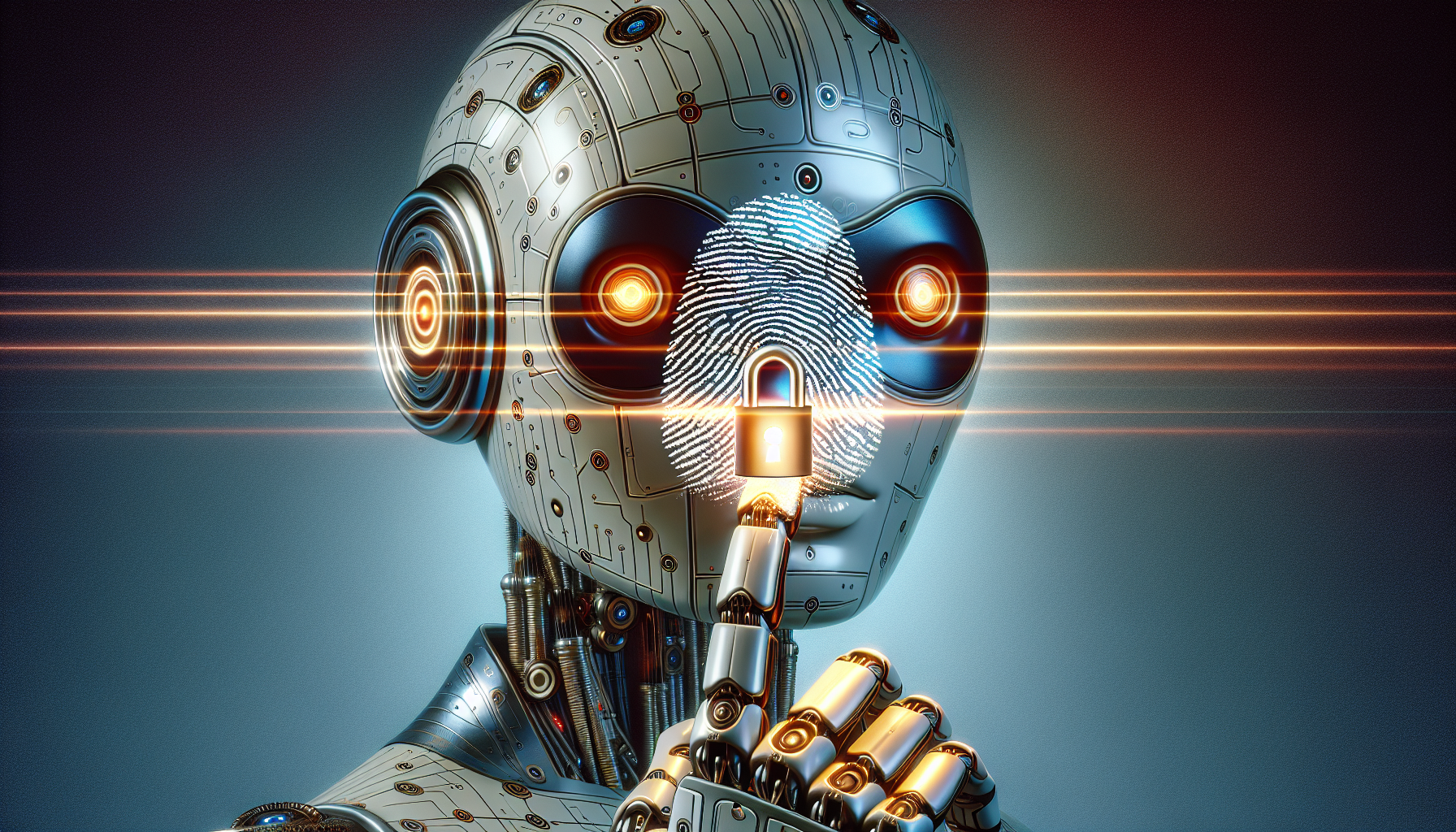
Cloudflare TLS Fingerprinting: What It Is and How to Solve It
Learn about Cloudflare's use of TLS fingerprinting for security, how it detects and blocks bots, and explore effective methods to solve it for web scraping and automated browsing tasks.
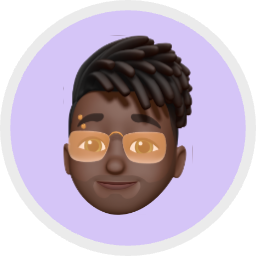
Lucas Mitchell
28-Feb-2025
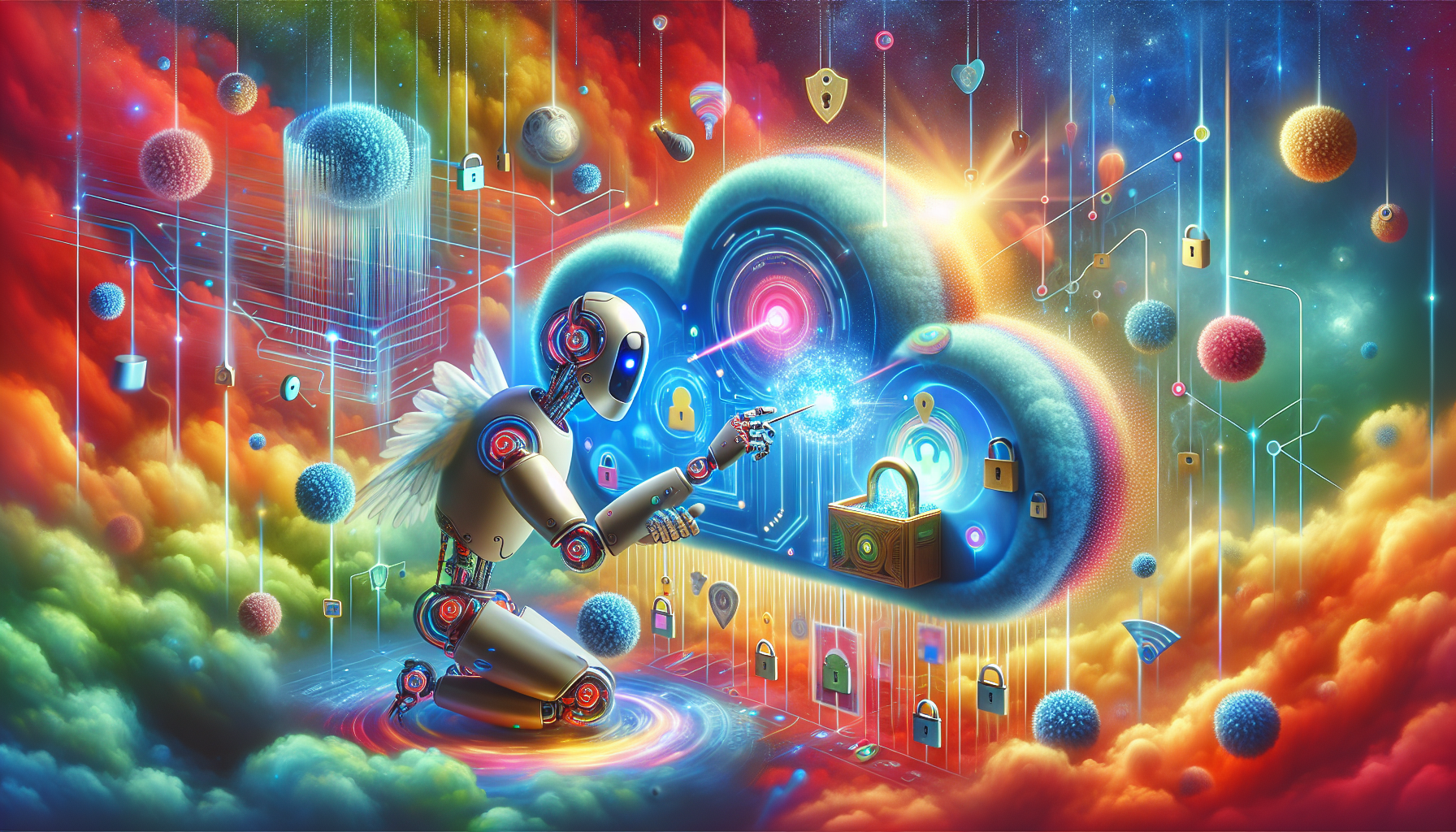
How to Extract Data from a Cloudflare-Protected Website
In this guide, we'll explore ethical and effective techniques to extract data from Cloudflare-protected websites.
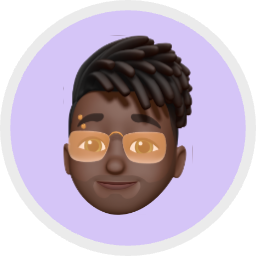
Lucas Mitchell
20-Feb-2025
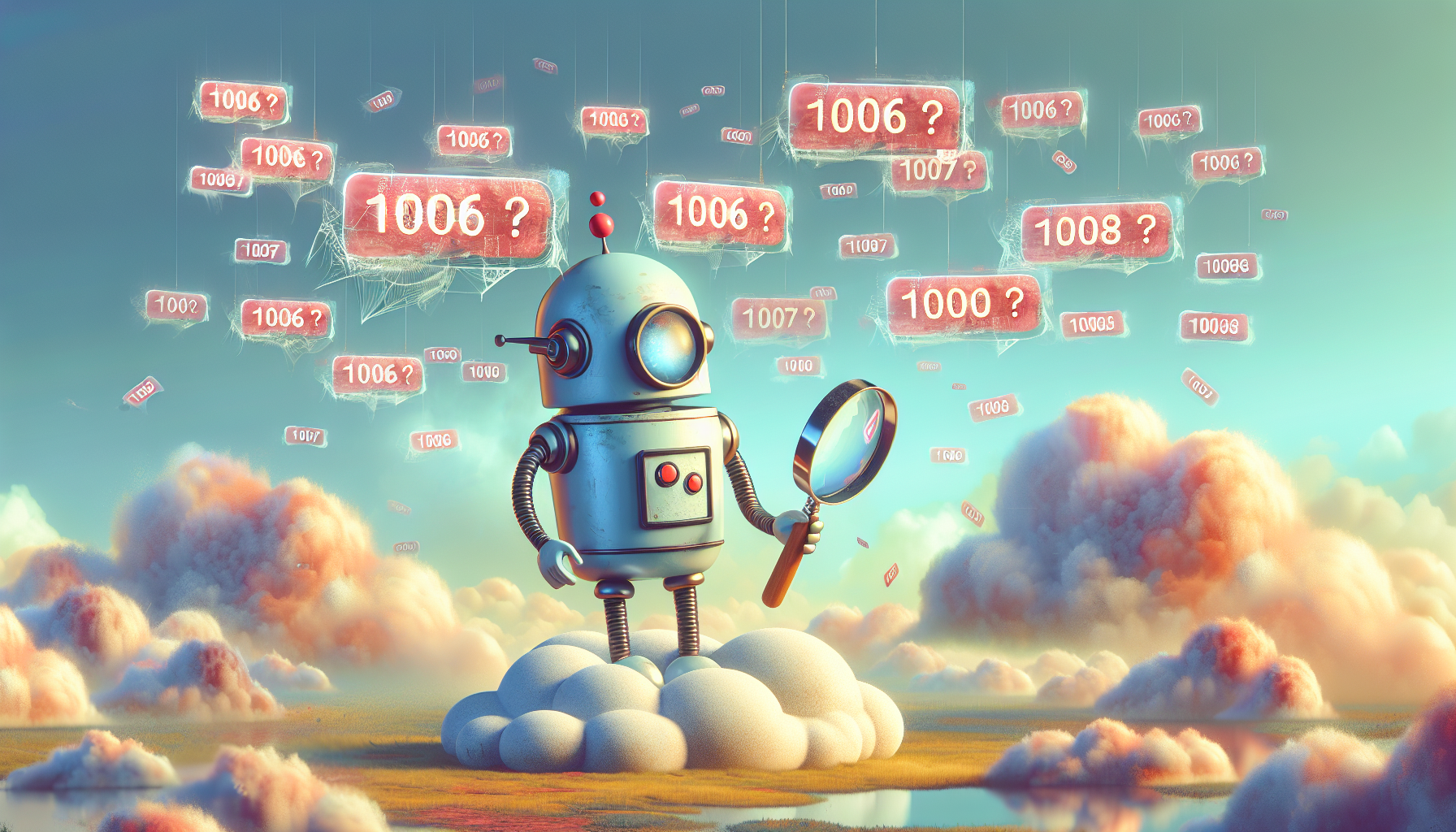
How to Fix Cloudflare Errors 1006, 1007, and 1008 Quickly
Cloudflare errors 1006, 1007, and 1008 can block your access due to suspicious or automated traffic. Learn quick fixes using premium proxies, user agent rotation, human behavior simulation, and IP address changes to overcome these roadblocks for smooth web scraping.
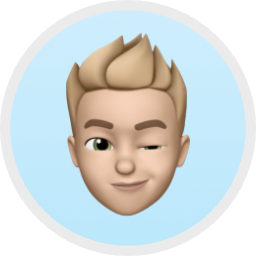
Ethan Collins
05-Feb-2025
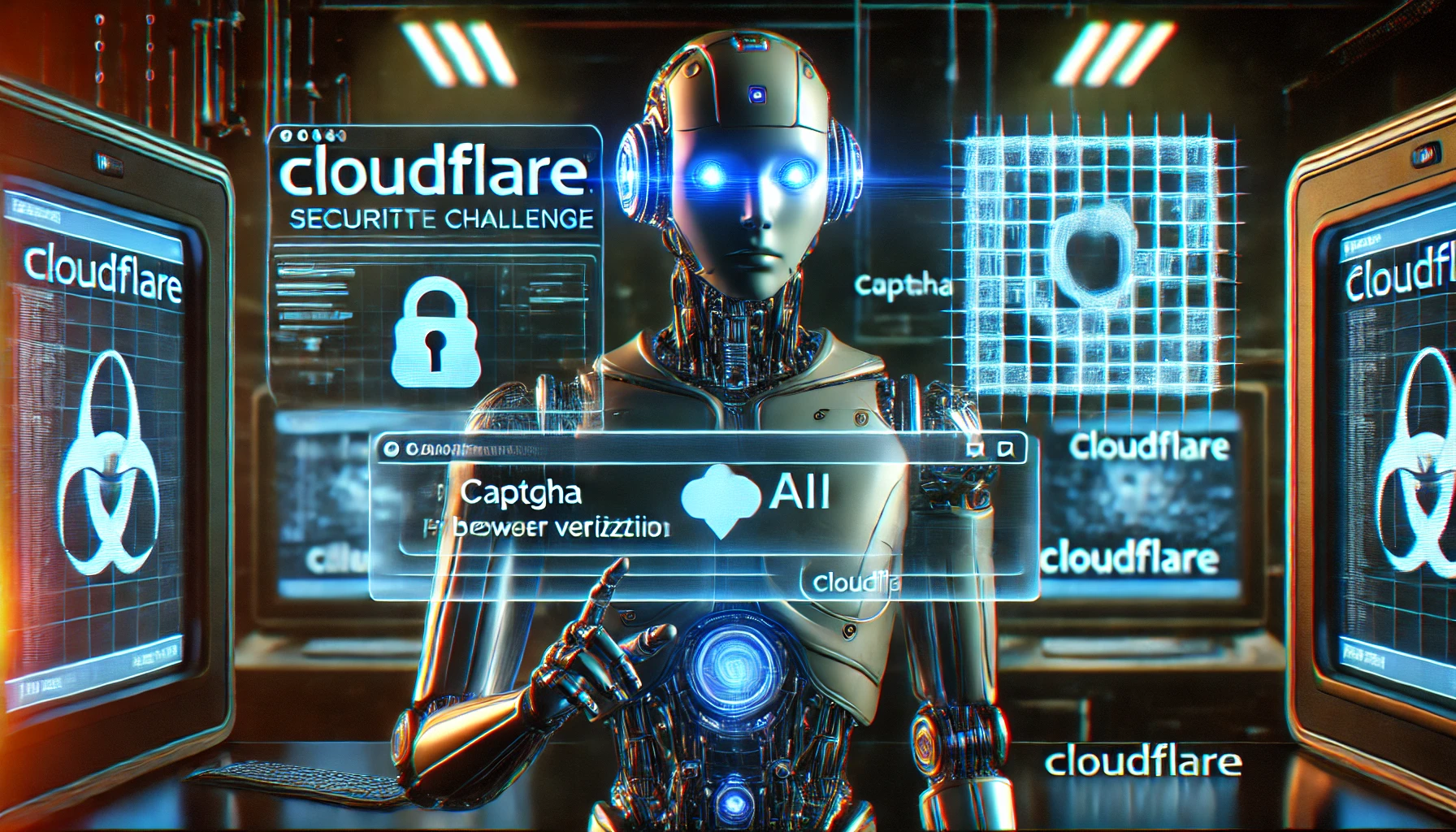
How to Bypass Cloudflare Challenge While Web Scraping in 2025
Learn how to bypass Cloudflare Challenge and Turnstile in 2025 for seamless web scraping. Discover Capsolver integration, TLS fingerprinting tips, and fixes for common errors to avoid CAPTCHA hell. Save time and scale your data extraction.
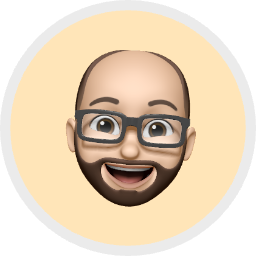
Aloísio Vítor
23-Jan-2025
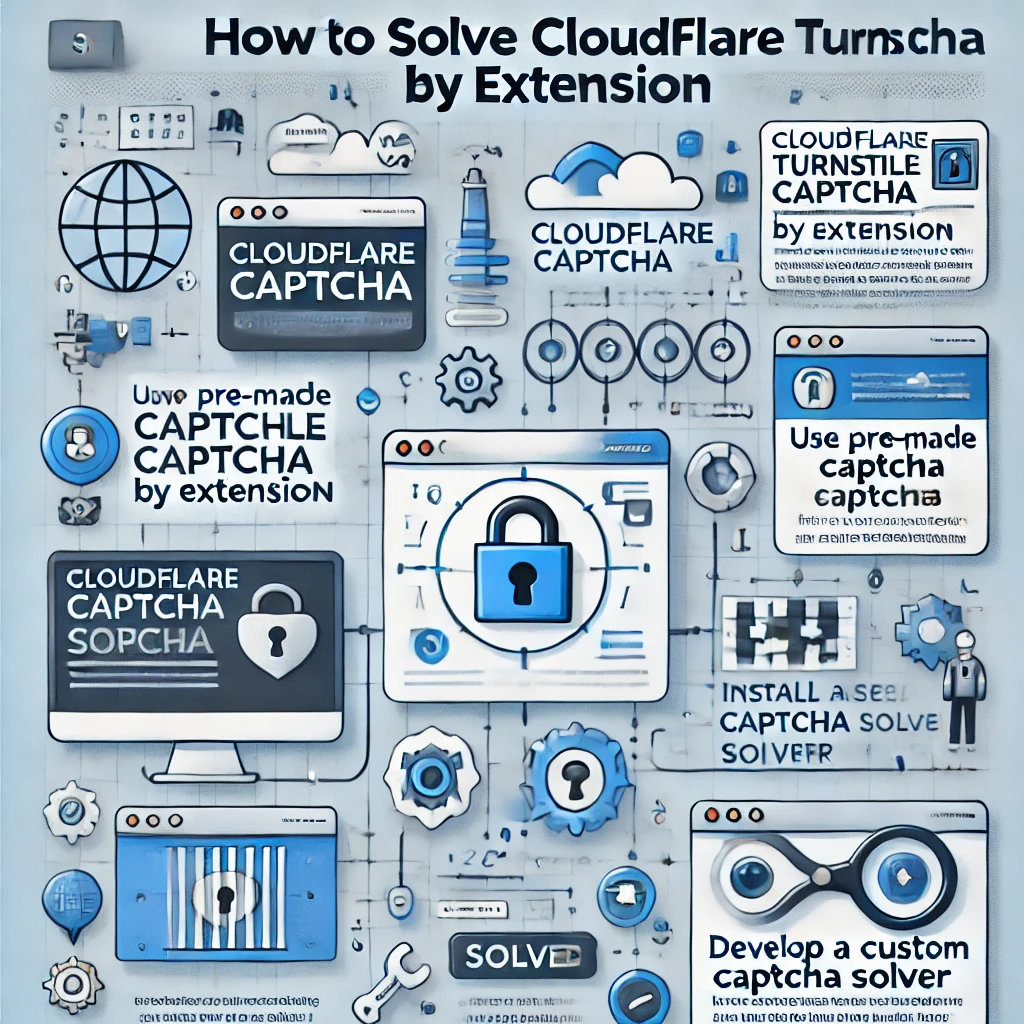
How to Solve Cloudflare Turnstile CAPTCHA by Extension
Learn how to bypass Cloudflare Turnstile CAPTCHA with Capsolver’s extension. Install guides for Chrome, Firefox, and automation tools like Puppeteer.
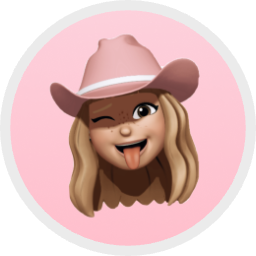
Adélia Cruz
23-Jan-2025
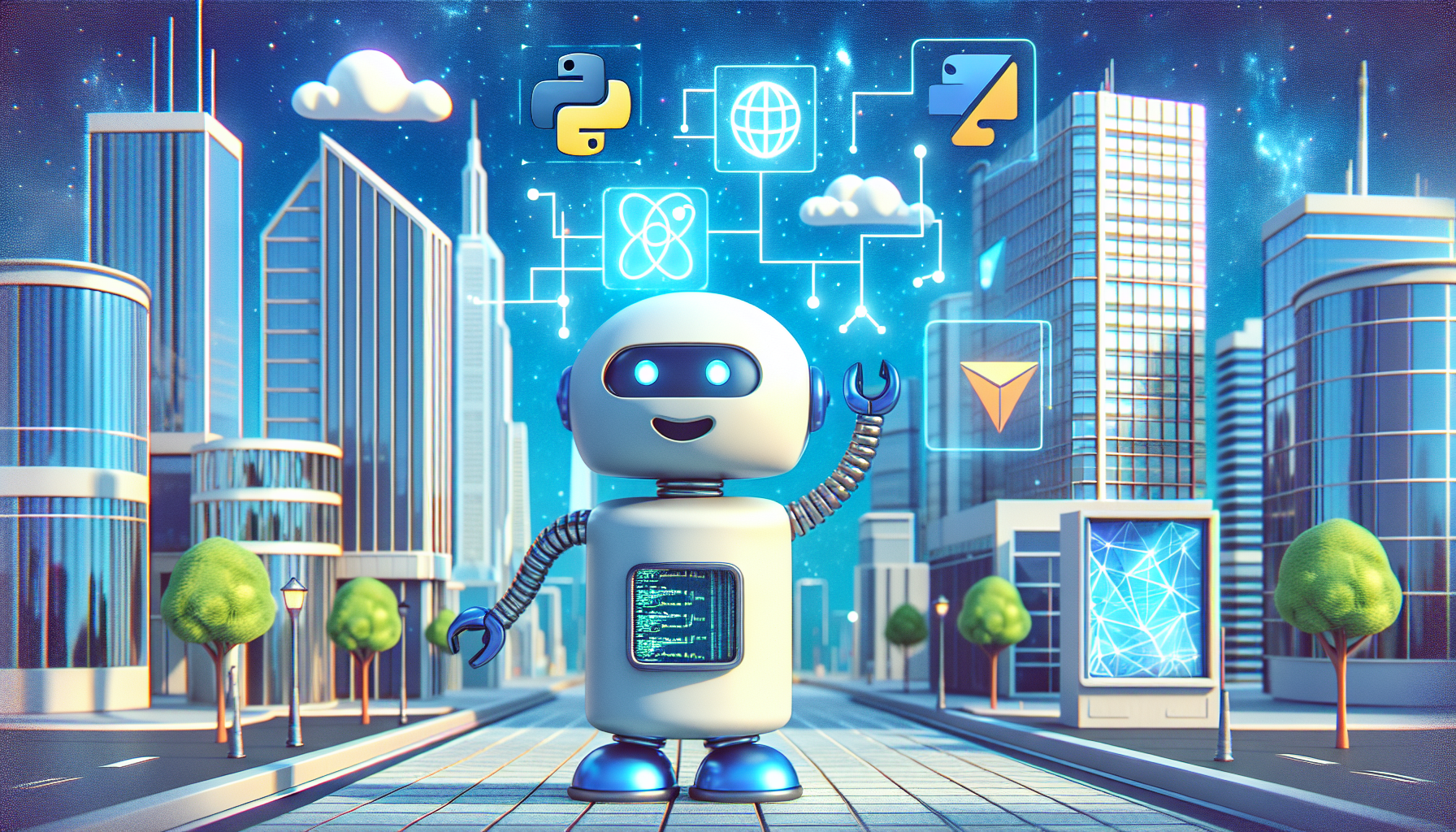
How to Solve Cloudflare by Using Python and Go in 2025
Will share insights on what Cloudflare Turnstile is, using Python and Go for these tasks, whether Turnstile can detect Python scrapers, and how to effectively it using solutions like CapSolver.
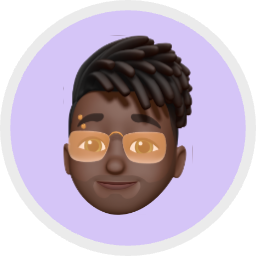
Lucas Mitchell
05-Nov-2024