How to Solve Cloudflare by Using Python and Go in 2025
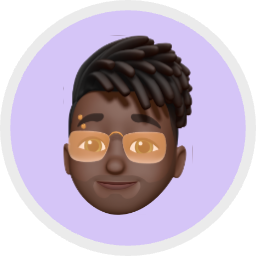
Lucas Mitchell
Automation Engineer
05-Nov-2024
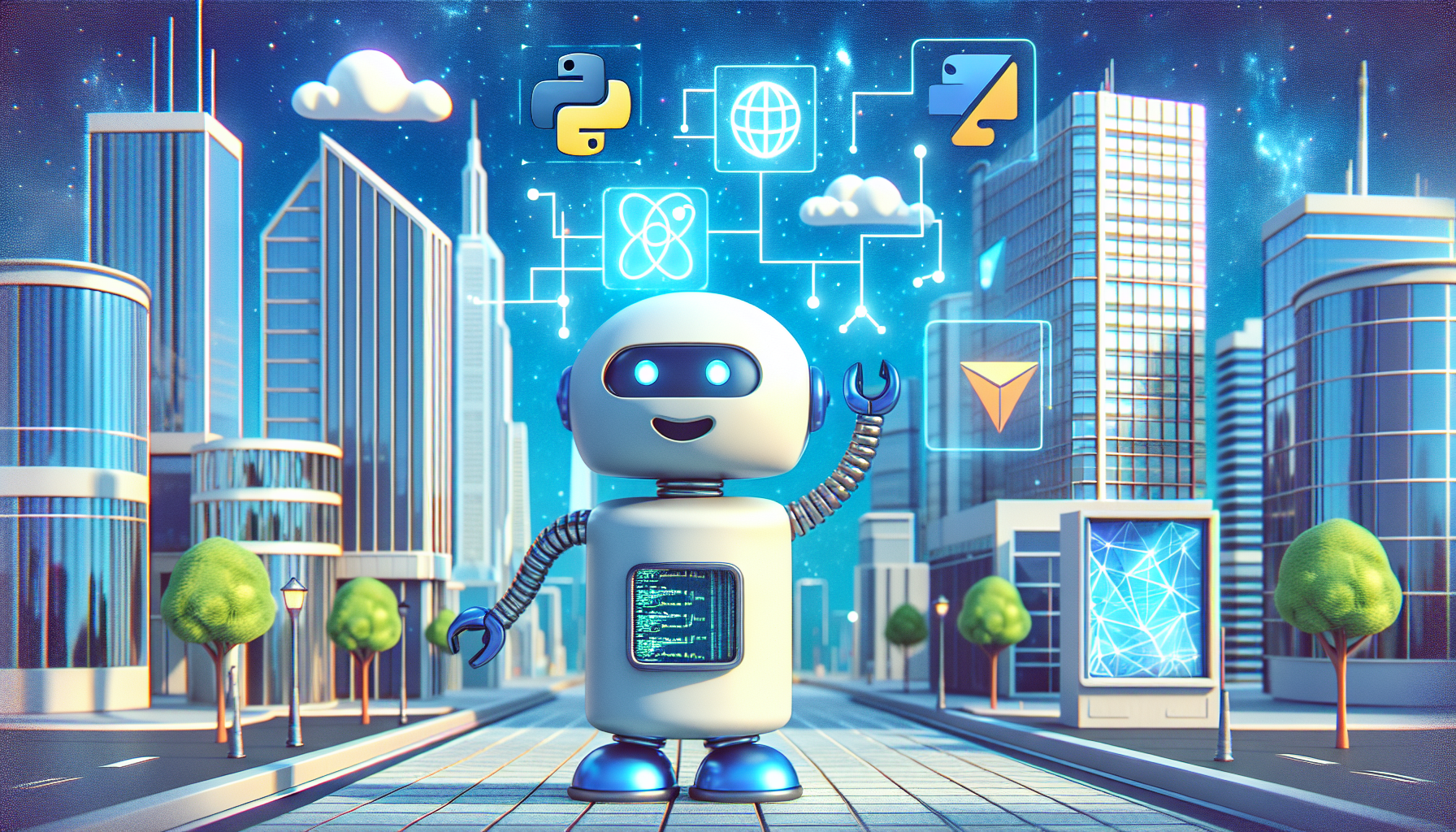
As a developer working with web automation and scraping, I've witnessed firsthand the challenges posed by increasingly sophisticated security measures. One such challenge is Cloudflare's Turnstile CAPTCHA system, which is now utilized by over 26 million websites globally. This advanced solution has redefined how we approach bot detection, boasting a remarkable ability to filter out 80% of malicious traffic while allowing genuine users to navigate websites without cumbersome puzzles.
In 2025, I've found that mastering the techniques to solve systems like Turnstile using programming languages such as Python and Go is essential for anyone looking to optimize their web scraping capabilities. In this article, I will share insights on what Cloudflare Turnstile is, why I prefer using Python and Go for these tasks, whether Turnstile can detect Python scrapers, and how to effectively solve it using solutions like CapSolver.
What is Cloudflare Turnstile?
Cloudflare Turnstile is a modern CAPTCHA system designed to distinguish human users from automated bots. Unlike traditional CAPTCHAs that often require users to solve complex puzzles, Turnstile operates primarily in the background, utilizing behavioral analysis and risk assessment to determine user authenticity. This means that users can often access websites without having to complete frustrating challenges, thus enhancing their experience.
Turnstile uses JavaScript-based techniques to evaluate various signals, such as mouse movements and interaction patterns, to ascertain whether a visitor is a human or a bot. This technology is rapidly gaining popularity among websites seeking to improve user engagement while maintaining a high level of security.
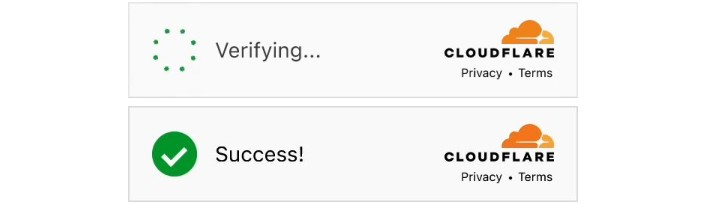
Why Use Python and Go?
Python and Go are powerful languages for overcoming challenges presented by Turnstile and are particularly well-suited for web automation. Here’s a closer look at why they’re ideal for this task:
Here’s a version with hyperlinks to resources for further exploration:
-
Python’s High Readability and Flexibility
Python’s simplicity and readability make it one of the most widely used languages for web automation and scraping. Tools like Selenium, Requests, and BeautifulSoup provide powerful ways to manage web interactions, handle cookies, and perform HTTP requests. These tools, combined with Python’s adaptability, make it a top choice for tasks that require fine-tuning or regular adjustments, especially in CAPTCHA handling. -
Go’s Speed and Concurrency
Go’s compiled nature means it runs more efficiently than interpreted languages like Python, ideal for high-performance tasks such as solving rate-limited CAPTCHAs. Go’s concurrency model, through goroutines, allows for handling multiple requests at once, reducing overall processing time in automation workflows. This makes Go a great choice for situations where speed and parallel processing are essential. -
Reliability of Combined Approaches
Combining Python and Go means leveraging Python for web navigation and complex interactions while using Go’s speed to handle frequent HTTP requests. This hybrid approach maintains performance and scalability, especially important when dealing with advanced security measures like Cloudflare Turnstile), which may use behavioral patterns for bot detection. -
Strong Community and Support
Both languages are backed by large, active communities that offer extensive libraries, forums, and documentation. This ecosystem means developers can easily find solutions, tools, and libraries to streamline the process of solving CAPTCHAs and handling complex automation tasks. Python, with its mature ecosystem, and Go, with its efficiency in concurrent tasks, complement each other well for use cases that demand resilience and adaptability.
By using Python and Go together, developers can tackle the intricate challenges posed by Cloudflare Turnstile and other CAPTCHA systems with an approach that balances flexibility, speed, and efficiency.
Can Cloudflare Turnstile Detect Python Scrapers?
While Turnstile mainly focuses on behavior rather than programming language, it’s possible for Python-based scrapers to be flagged by observing specific indicators:
- Unnatural User Behavior: Rapid and precise actions can signal bot-like behavior.
- IP Reputation: Requests from known data centers or proxy IPs may trigger Turnstile’s scrutiny.
- User-Agent Strings: Default User-Agent strings associated with libraries (e.g., Requests) may mark traffic as suspicious.
To avoid detection, mimic human interaction patterns and use residential or high-quality proxies.
How to Solve Cloudflare Turnstile
Solving Turnstile can be challenging, but with the right tools, it’s achievable. Below, I’ll introduce a Python and Go-based method, integrating CapSolver’s API, to solve Turnstile CAPTCHA efficiently.
Bonus Code
Claim Your Bonus Code for top captcha solutions; CapSolver: WEBS. After redeeming it, you will get an extra 5% bonus after each recharge, Unlimited
Python Implementation with CapSolver
The following code demonstrates how to solve Turnstile using Python and CapSolver's API.
python
import time
import requests
import tls_client
CAPSOLVER_API_KEY = "YOUR_CAPSOLVER_API_KEY"
PAGE_URL = "https://dash.cloudflare.com/login"
SITE_KEY = "0x4AAAAAAAJel0iaAR3mgkjp"
PROXY = "YOUR_PROXY"
# Create a task with CapSolver to bypass Turnstile
def call_capsolver():
data = {
"clientKey": CAPSOLVER_API_KEY,
"task": {
"type": "AntiTurnstileTaskProxyLess",
"websiteURL": PAGE_URL,
"websiteKey": SITE_KEY,
"metadata": {"action": "login"}
}
}
uri = 'https://api.capsolver.com/createTask'
res = requests.post(uri, json=data)
task_id = res.json().get('taskId')
if not task_id:
print("Failed to create task:", res.text)
return None
# Poll for task completion
while True:
time.sleep(1)
data = {
"clientKey": CAPSOLVER_API_KEY,
"taskId": task_id
}
response = requests.post('https://api.capsolver.com/getTaskResult', json=data)
resp = response.json()
if resp.get('status') == "ready":
print("Task successful:", resp)
return resp.get('solution')
if resp.get('status') == "failed" or resp.get("errorId"):
print("Task failed:", response.text)
return None
def login(token, userAgent):
headers = {
'Cookie': f'cf_clearance={token}',
'Host': 'dash.cloudflare.com',
'User-Agent': userAgent
}
session = tls_client.Session(client_identifier="chrome_120", random_tls_extension_order=True)
response = session.post(
url='https://dash.cloudflare.com/api/v4/login',
headers=headers,
data={"cf_challenge_response": token, "email": "your_email", "password": "your_password"},
)
print("Login Response:", response.status_code)
if response.status_code != 403:
print('Login Success:', response.text)
if __name__ == "__main__":
solution = call_capsolver()
if solution:
login(solution.get("token"), "Mozilla/5.0 (Macintosh; Intel Mac OS X 10_15_7) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/129.0.0.0 Safari/537.36")
Go Implementation with CapSolver
Here’s how to accomplish the same task in Go:
go
package main
import (
"fmt"
"github.com/imroc/req/v3"
"github.com/tidwall/gjson"
"log"
"time"
)
func createTask(apiKey, pageURL, siteKey string) string {
client := req.C()
postData := map[string]interface{}{
"clientKey": apiKey,
"task": map[string]interface{}{
"type": "AntiTurnstileTaskProxyLess",
"websiteURL": pageURL,
"websiteKey": siteKey,
"metadata": map[string]string{"action": "login"},
},
}
resp, err := client.R().SetBodyJsonMarshal(postData).Post("https://api.capsolver.com/createTask")
if err != nil {
log.Fatal(err)
}
return gjson.Get(resp.String(), "taskId").String()
}
func getTaskResult(apiKey, taskId string) map[string]gjson.Result {
client := req.C()
for {
postData := map[string]interface{}{
"clientKey": apiKey,
"taskId": taskId,
}
resp, err := client.R().SetBodyJsonMarshal(postData).Post("https://api.capsolver.com/getTaskResult")
if err != nil {
log.Fatal(err)
}
if gjson.Get(resp.String(), "status").String() == "ready" {
return gjson.Get(resp.String(), "solution").Map()
}
time.Sleep(3 * time.Second)
}
}
func main() {
apiKey := "YOUR_CAPSOLVER_API_KEY"
pageURL := "https://dash.cloudflare.com/login"
siteKey := "0x4AAAAAAAJel0iaAR3mgkjp"
taskId := createTask(apiKey, pageURL, siteKey)
solution := getTaskResult(apiKey, taskId)
if solution != nil {
fmt.Println("Token obtained:", solution["token"].String())
}
}
These code samples illustrate how to integrate CapSolver to solve Cloudflare’s Turnstile CAPTCHA by automating CAPTCHA solution retrieval and submitting a successful login request.
Final Thoughts
Cloudflare's Turnstile continues to present challenges for web automation. Using powerful languages like Python and Go, along with services like CapSolver, offers a streamlined way to solve Turnstile challenges while retaining efficient, secure access.
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
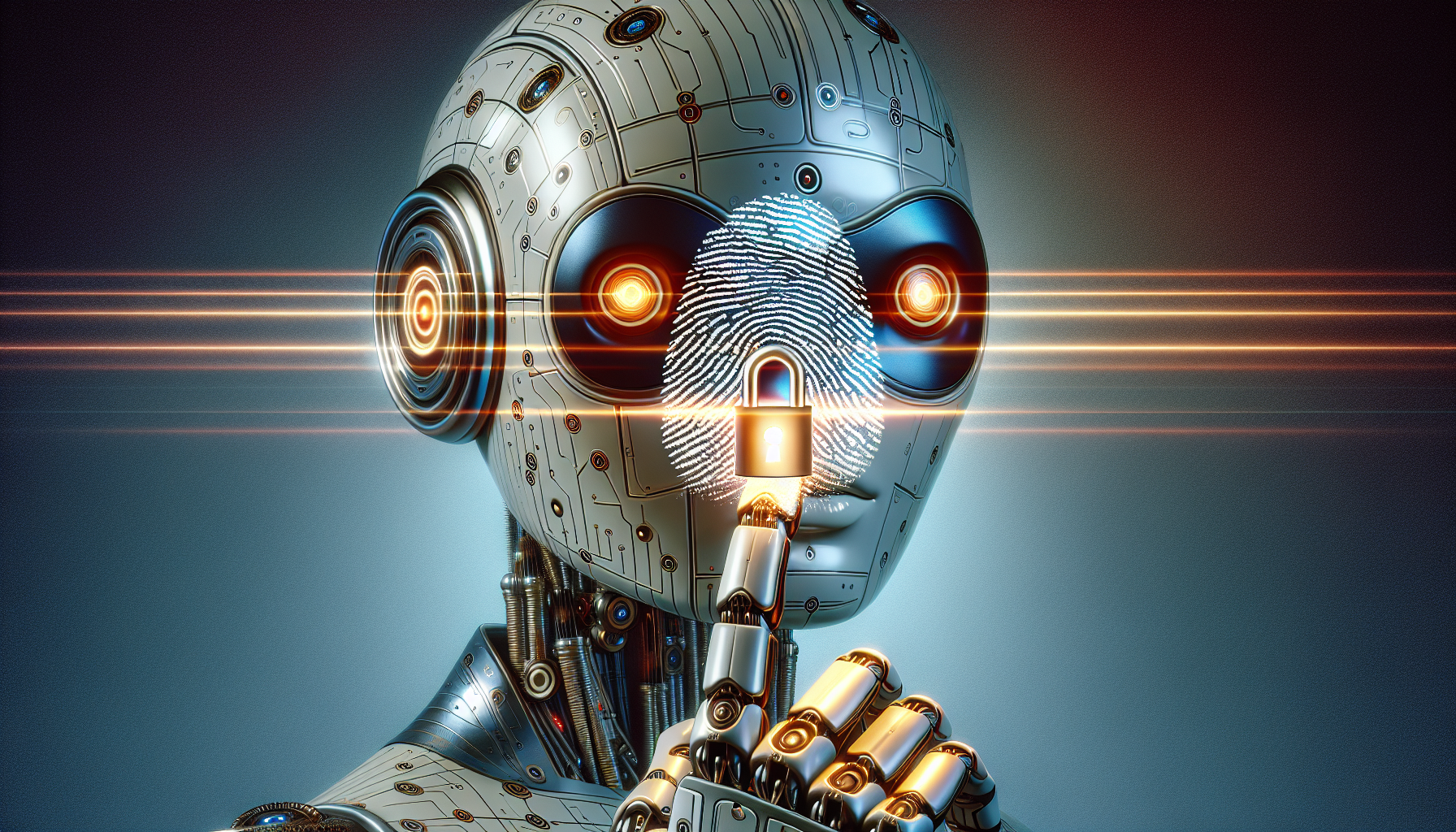
Cloudflare TLS Fingerprinting: What It Is and How to Solve It
Learn about Cloudflare's use of TLS fingerprinting for security, how it detects and blocks bots, and explore effective methods to solve it for web scraping and automated browsing tasks.
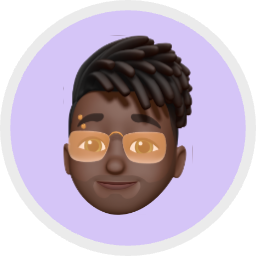
Lucas Mitchell
28-Feb-2025
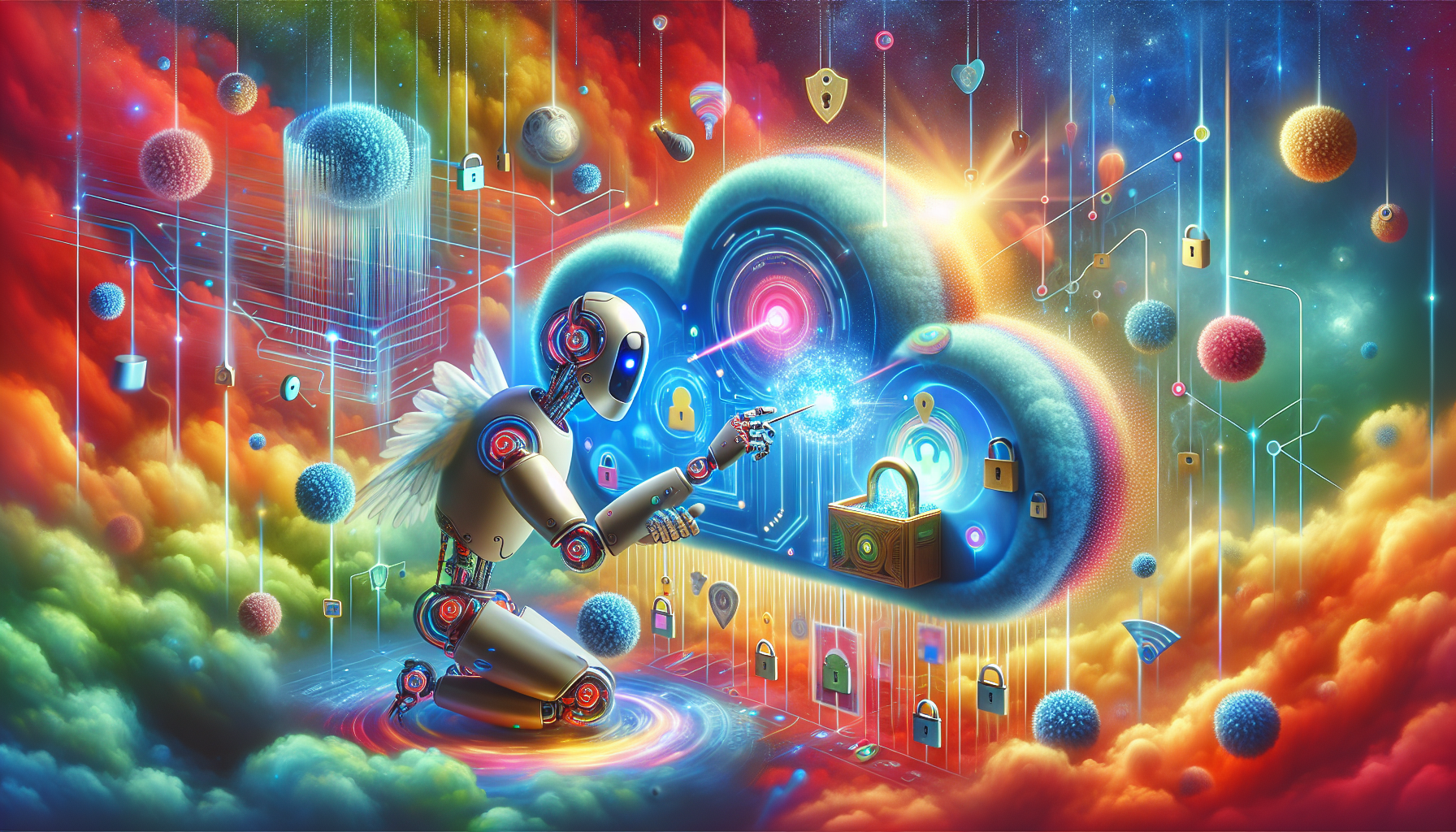
How to Extract Data from a Cloudflare-Protected Website
In this guide, we'll explore ethical and effective techniques to extract data from Cloudflare-protected websites.
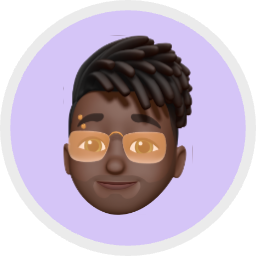
Lucas Mitchell
20-Feb-2025
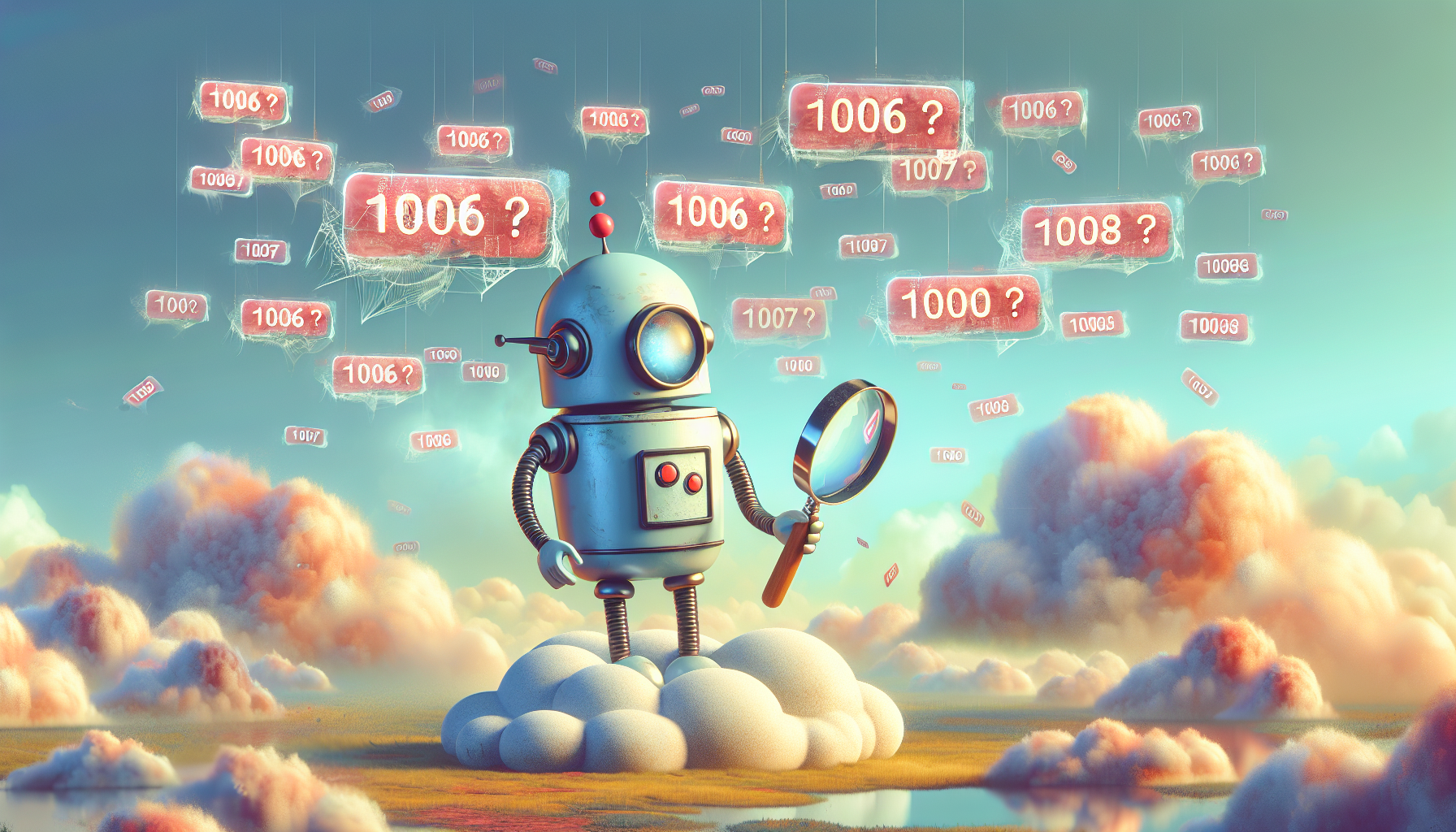
How to Fix Cloudflare Errors 1006, 1007, and 1008 Quickly
Cloudflare errors 1006, 1007, and 1008 can block your access due to suspicious or automated traffic. Learn quick fixes using premium proxies, user agent rotation, human behavior simulation, and IP address changes to overcome these roadblocks for smooth web scraping.
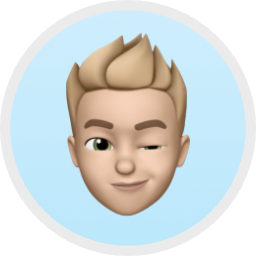
Ethan Collins
05-Feb-2025
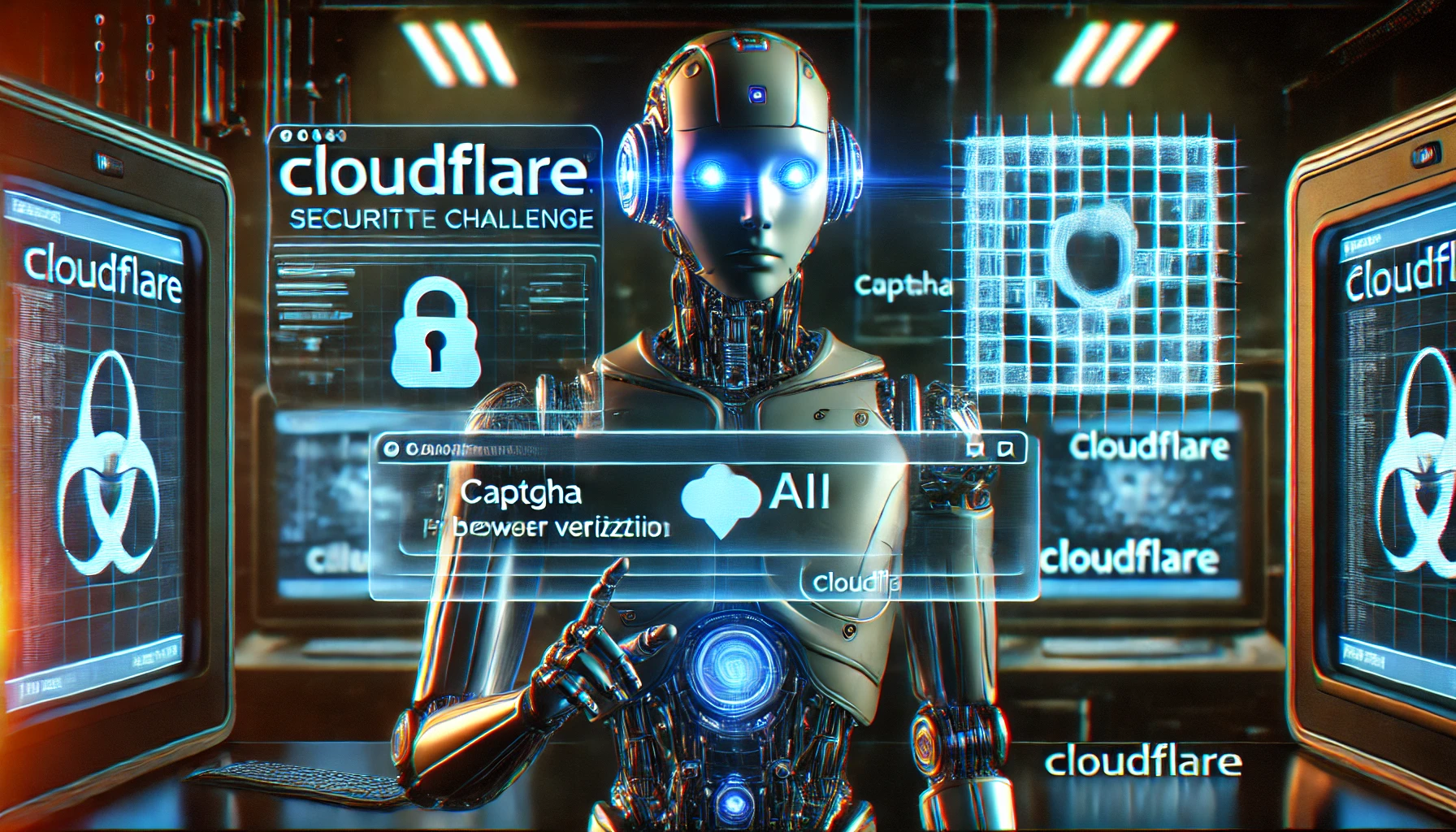
How to Bypass Cloudflare Challenge While Web Scraping in 2025
Learn how to bypass Cloudflare Challenge and Turnstile in 2025 for seamless web scraping. Discover Capsolver integration, TLS fingerprinting tips, and fixes for common errors to avoid CAPTCHA hell. Save time and scale your data extraction.
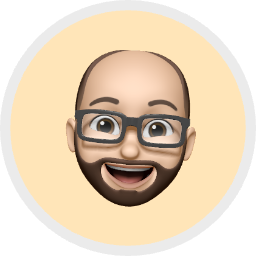
Aloísio Vítor
23-Jan-2025
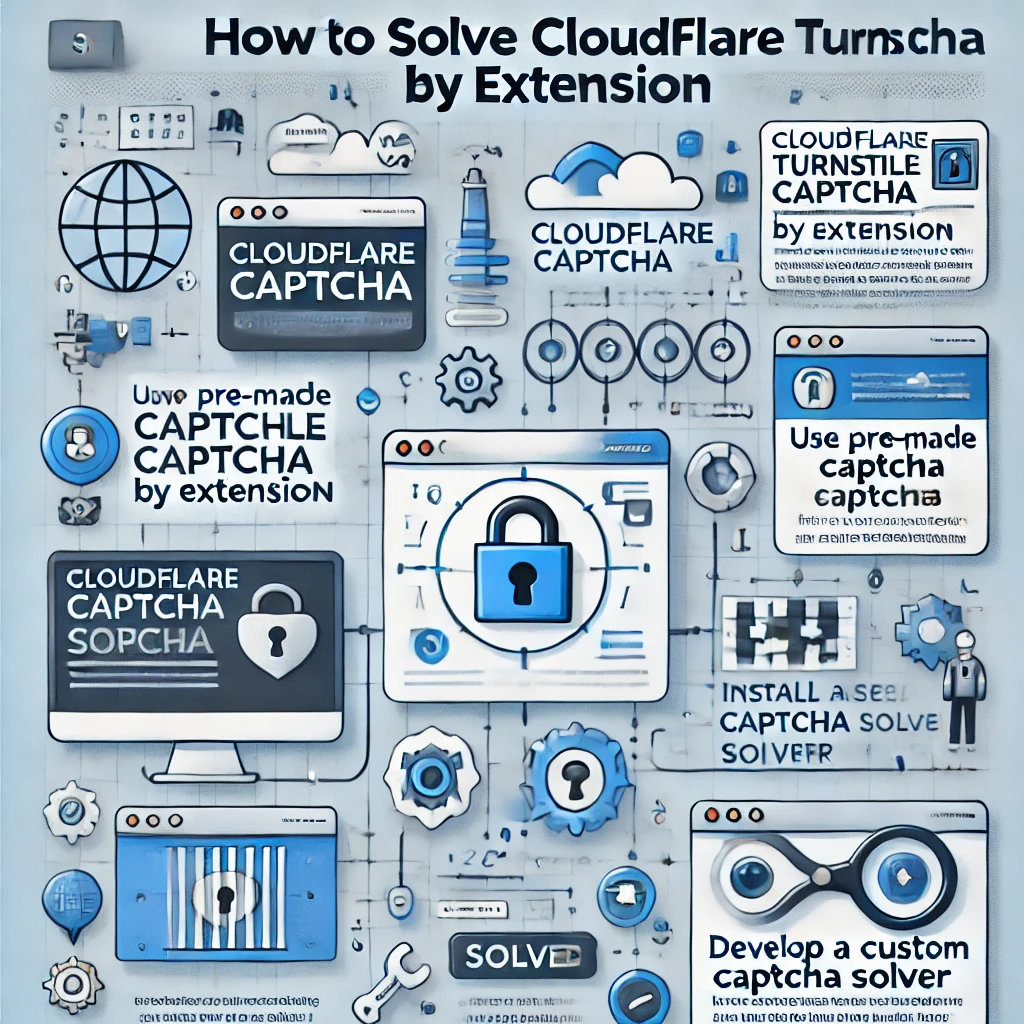
How to Solve Cloudflare Turnstile CAPTCHA by Extension
Learn how to bypass Cloudflare Turnstile CAPTCHA with Capsolver’s extension. Install guides for Chrome, Firefox, and automation tools like Puppeteer.
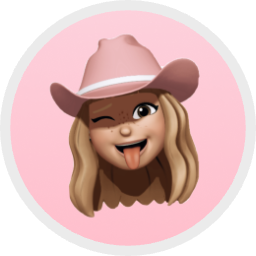
Adélia Cruz
23-Jan-2025
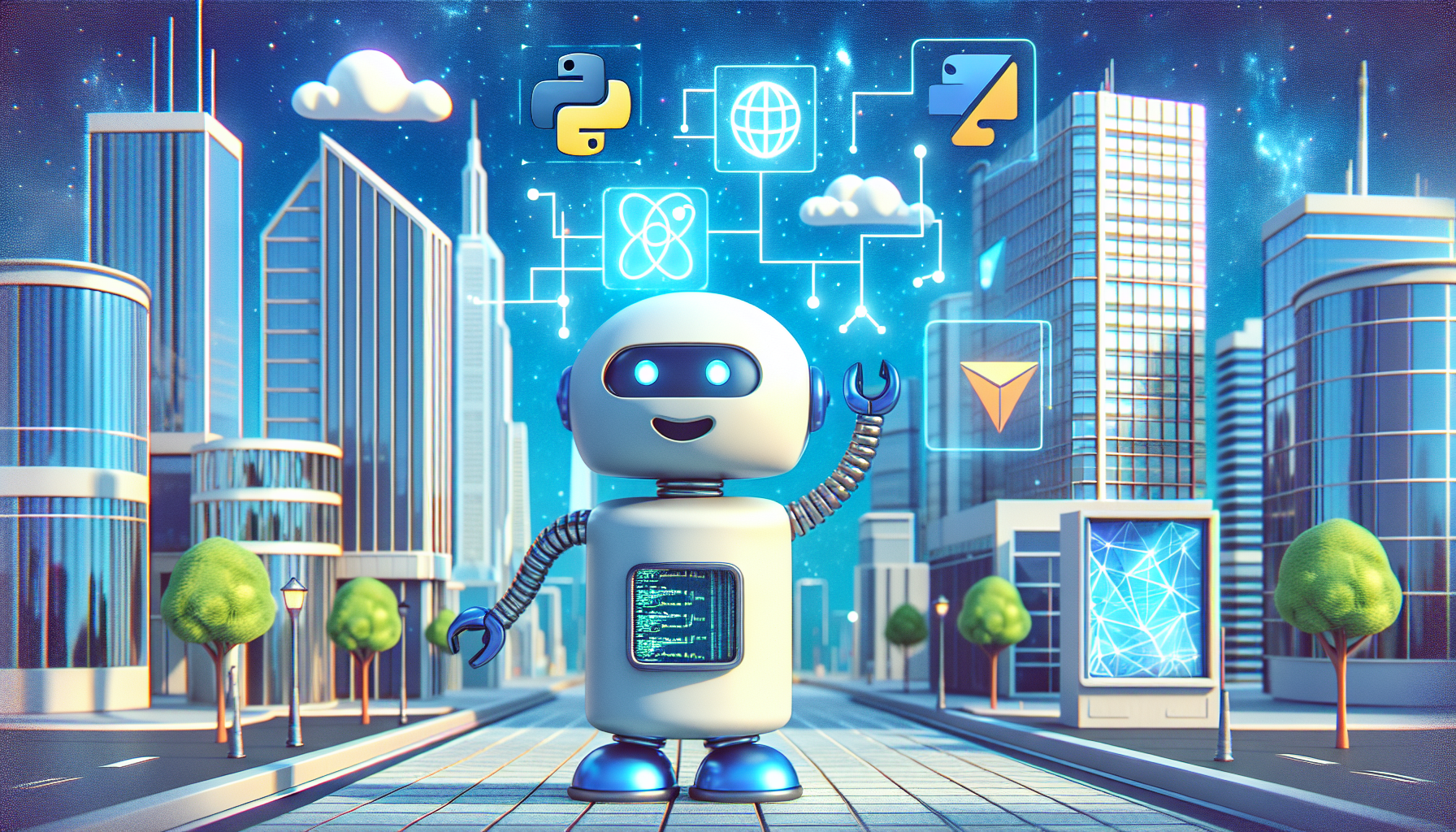
How to Solve Cloudflare by Using Python and Go in 2025
Will share insights on what Cloudflare Turnstile is, using Python and Go for these tasks, whether Turnstile can detect Python scrapers, and how to effectively it using solutions like CapSolver.
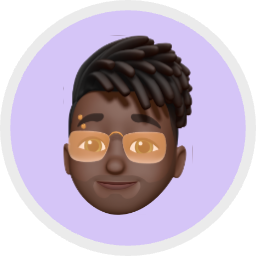
Lucas Mitchell
05-Nov-2024