How to Automate Cloudflare Turnstile Solve for Web Crawling
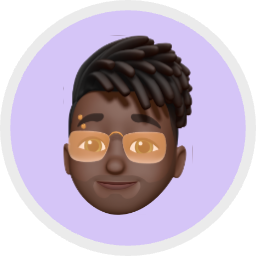
Lucas Mitchell
Automation Engineer
27-Sep-2024
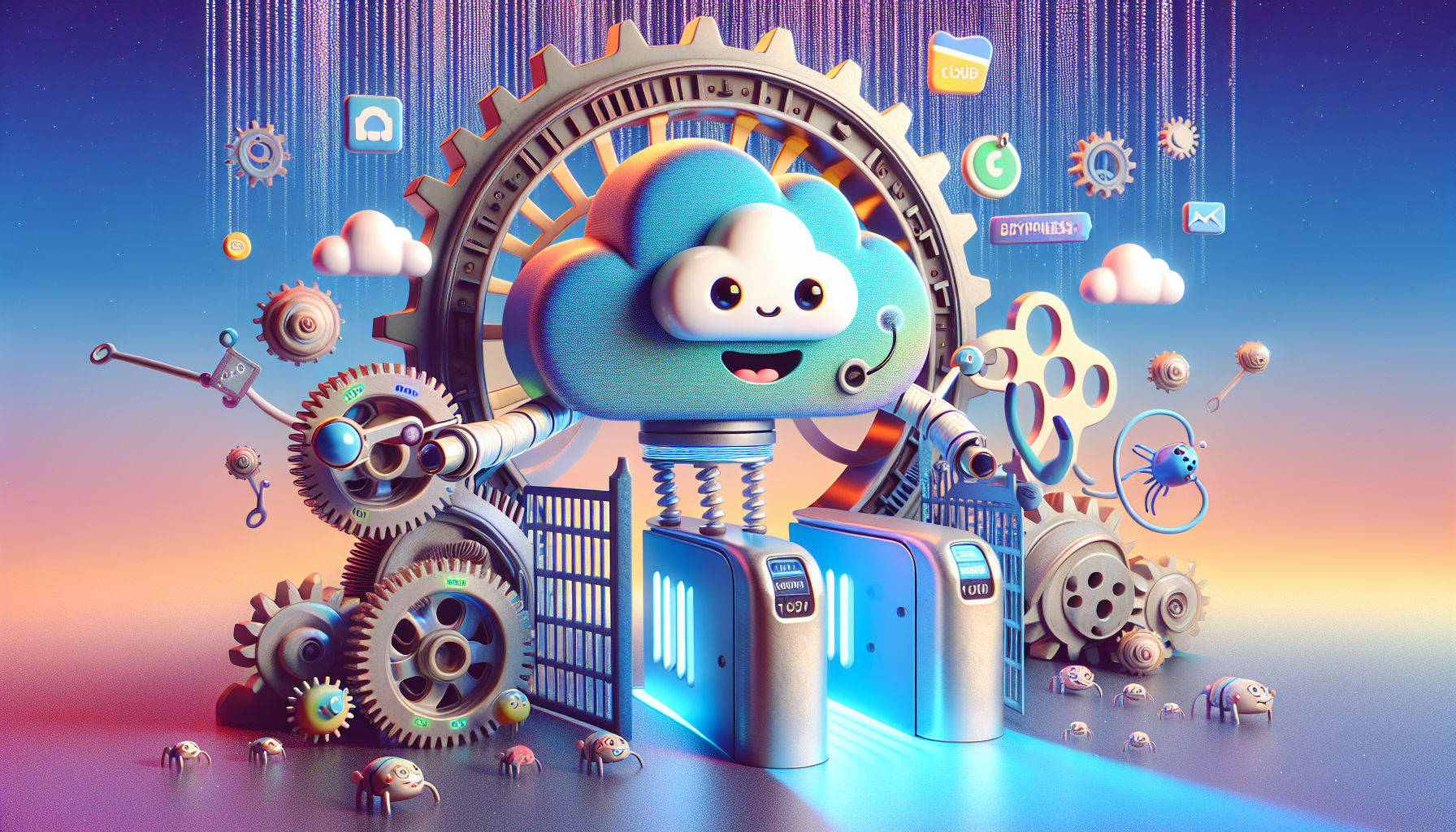
Cloudflare's Turnstile CAPTCHA presents a significant obstacle for web crawlers and automation tools. As a security feature, it ensures that requests made to a website are legitimate, preventing malicious bots from accessing protected content. However, for legitimate automation and web scraping tasks, solving Cloudflare Turnstile CAPTCHA is crucial to maintaining the workflow without interruptions.
In this guide, we will explore strategies for handling Cloudflare Turnstile CAPTCHA in web crawling and discuss techniques to automate its solution using Puppeteer and CapSolver in Python.
What Is Cloudflare Turnstile CAPTCHA?
Cloudflare Turnstile CAPTCHA is a sophisticated anti-bot mechanism. Unlike traditional CAPTCHA challenges that require users to solve puzzles or click on images, Turnstile employs invisible security checks to identify whether a request comes from a bot or a real user without interrupting the user experience.
This CAPTCHA uses a combination of factors such as:
- User behavior: Patterns that indicate bot-like or human-like activity.
- IP reputation: The history of the IP address, including whether it has been flagged for suspicious activity.
- Browser fingerprints: Information about the browser and system being used to access the site.
For web crawlers and scrapers, Turnstile CAPTCHA can block your script from completing its task. To continue crawling efficiently, you'll need to automate the process of solving this CAPTCHA.
Bonus Code
Claim Your Bonus Code for top captcha solutions; CapSolver: WEBS. After redeeming it, you will get an extra 5% bonus after each recharge, Unlimited
Challenges for Web Crawlers
Cloudflare Turnstile CAPTCHA is designed to be resilient to most common automation attempts. Web scrapers often encounter this CAPTCHA when trying to access protected content, resulting in denied access or incomplete data collection. Solving this challenge manually is not feasible for large-scale scraping, making automation crucial.
A typical approach to solving Cloudflare Turnstile CAPTCHA involves:
- Simulating human-like interactions to avoid triggering the CAPTCHA.
- Rotating IP addresses through residential or datacenter proxies.
- Using third-party CAPTCHA-solving services to solve challenges when they appear.
Let's explore the tools you can use to achieve this.
Tools and Libraries for Automating Cloudflare Turnstile CAPTCHA
To solve Cloudflare Turnstile CAPTCHA in your web crawler, you'll need a combination of scraping tools, proxies, and CAPTCHA-solving services. Here's a breakdown:
-
Web Scraping Libraries:
- Tools like Selenium, Puppeteer, or Playwright are commonly used to automate browsers and interact with web pages. They allow you to handle JavaScript-heavy sites and pass through basic bot detection measures.
- Puppeteer, in particular, is a Node.js library that provides high-level APIs to control Chrome or Chromium browsers. It’s ideal for managing browser sessions in scraping tasks, especially when dealing with CAPTCHAs.
-
Proxies:
- Residential or rotating proxies are essential to simulate different users and prevent IP bans or throttling. Proxies help distribute requests across multiple IPs to avoid triggering anti-bot measures like Turnstile.
- Rotating proxies dynamically assign a different IP for each request, making it harder for Cloudflare to identify patterns in scraping behavior.
-
CAPTCHA-Solving Services:
- Services like CapSolver are designed to automatically solve CAPTCHA challenges. These services integrate with web scraping tools and can solve Cloudflare Turnstile CAPTCHA in real time by providing the necessary tokens for bypassing the CAPTCHA without manual intervention.
How to Solve Cloudflare Turnstile CAPTCHA with Puppeteer and CapSolver
In this example, we will demonstrate how to solve Cloudflare Turnstile CAPTCHA using Puppeteer and CapSolver.
Prerequisites
Make sure you have the following installed:
- Puppeteer:
npm install puppeteer
- Axios:
npm install axios
(for making API requests)
Step-by-Step Guide
javascript
const puppeteer = require('puppeteer');
const axios = require('axios');
const clientKey = 'your-client-key-here'; // Replace with your CapSolver client key
const websiteURL = 'https://example.com'; // Replace with your target website URL
const websiteKey = 'your-site-key-here'; // Replace with the site key from the target website
// Function to create a task for solving Turnstile CAPTCHA
async function createTask() {
const response = await axios.post('https://api.capsolver.com/createTask', {
clientKey: clientKey,
task: {
type: "AntiTurnstileTaskProxyLess",
websiteURL: websiteURL,
websiteKey: websiteKey
}
}, {
headers: {
'Content-Type': 'application/json',
'Pragma': 'no-cache'
}
});
return response.data.taskId;
}
// Function to retrieve the task result
async function getTaskResult(taskId) {
let response;
while (true) {
response = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: clientKey,
taskId: taskId
}, {
headers: {
'Content-Type': 'application/json'
}
});
if (response.data.status === 'ready') {
return response.data.solution;
}
console.log('Solution not ready yet, checking again in 5 seconds...');
await new Promise(resolve => setTimeout(resolve, 5000));
}
}
// Main Puppeteer script to automate browsing and solving CAPTCHA
(async () => {
const taskId = await createTask();
const result = await getTaskResult(taskId);
let solution = result.token;
const browser = await puppeteer.launch({ headless: false });
const page = await browser.newPage();
await page.goto(websiteURL);
await page.waitForSelector('input[name="cf-turnstile-response"]');
// Insert the CAPTCHA solution token into the form
await page.evaluate(solution => {
document.querySelector('input[name="cf-turnstile-response"]').value = solution;
}, solution);
// Take a screenshot of the page for verification purposes
await page.screenshot({ path: 'example.png' });
await browser.close();
})();
Setting Up a Web Scraping Environment for Turnstile
To ensure smooth scraping without interruptions, it's important to have a well-configured environment:
-
Headless Browsers: Use headless browsers like Puppeteer or Playwright to emulate human behavior while staying lightweight. These tools can handle JavaScript rendering, form submissions, and dynamic content.
-
Proxy Rotation: Implement proxy rotation to avoid getting blocked. Residential proxies are less likely to be flagged than datacenter ones. You can also integrate proxy providers like IPRoyal for reliable proxy services.
-
Session Management: Maintain and reuse browser sessions when possible to avoid raising suspicion by logging in repeatedly or triggering security mechanisms.
-
CAPTCHA Solvers: Leverage CAPTCHA-solving services like CapSolver to solve complex CAPTCHA challenges. These services provide APIs that handle CAPTCHA-solving behind the scenes, allowing your scraper to continue its workflow.
Conclusion
Solving Cloudflare Turnstile CAPTCHA is essential for legitimate web crawling tasks that require uninterrupted access to data. Combining web automation libraries like Puppeteer, proxies, and third-party CAPTCHA solvers such as CapSolver can help you overcome this challenge effectively. With the right tools and strategies, your scraper can continue to gather data efficiently without manual intervention.
Note on Compliance
Important: When engaging in web scraping, it's crucial to adhere to legal and ethical guidelines. Always ensure that you have permission to scrape the target website, and respect the site's
robots.txt
file and terms of service. CapSolver firmly opposes the misuse of our services for any non-compliant activities. Misuse of automated tools to bypass CAPTCHAs without proper authorization can lead to legal consequences. Make sure your scraping activities are compliant with all applicable lcaptcha and regulations to avoid potential issues.
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
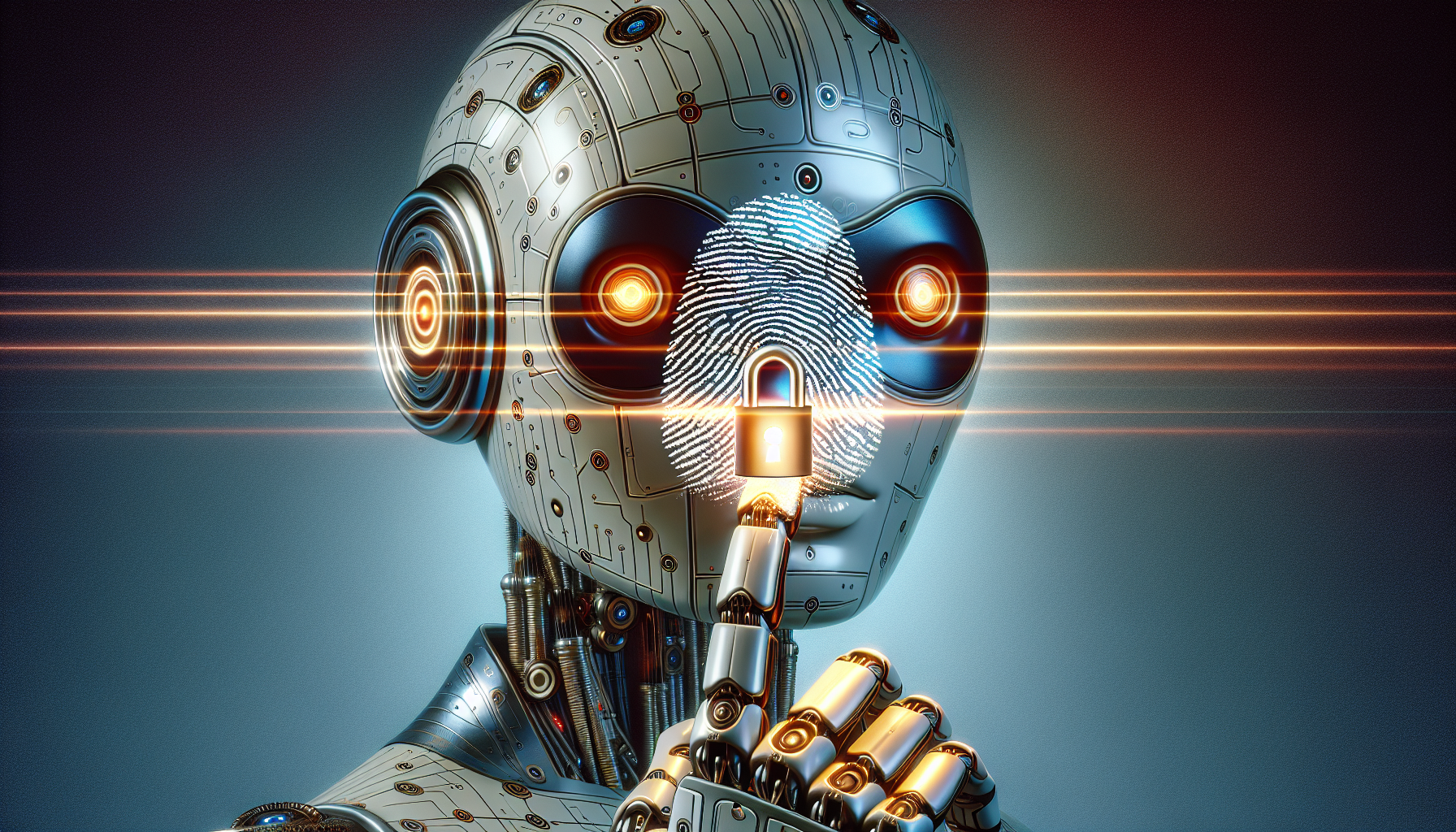
Cloudflare TLS Fingerprinting: What It Is and How to Solve It
Learn about Cloudflare's use of TLS fingerprinting for security, how it detects and blocks bots, and explore effective methods to solve it for web scraping and automated browsing tasks.
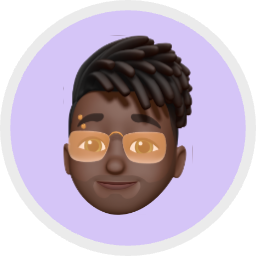
Lucas Mitchell
28-Feb-2025
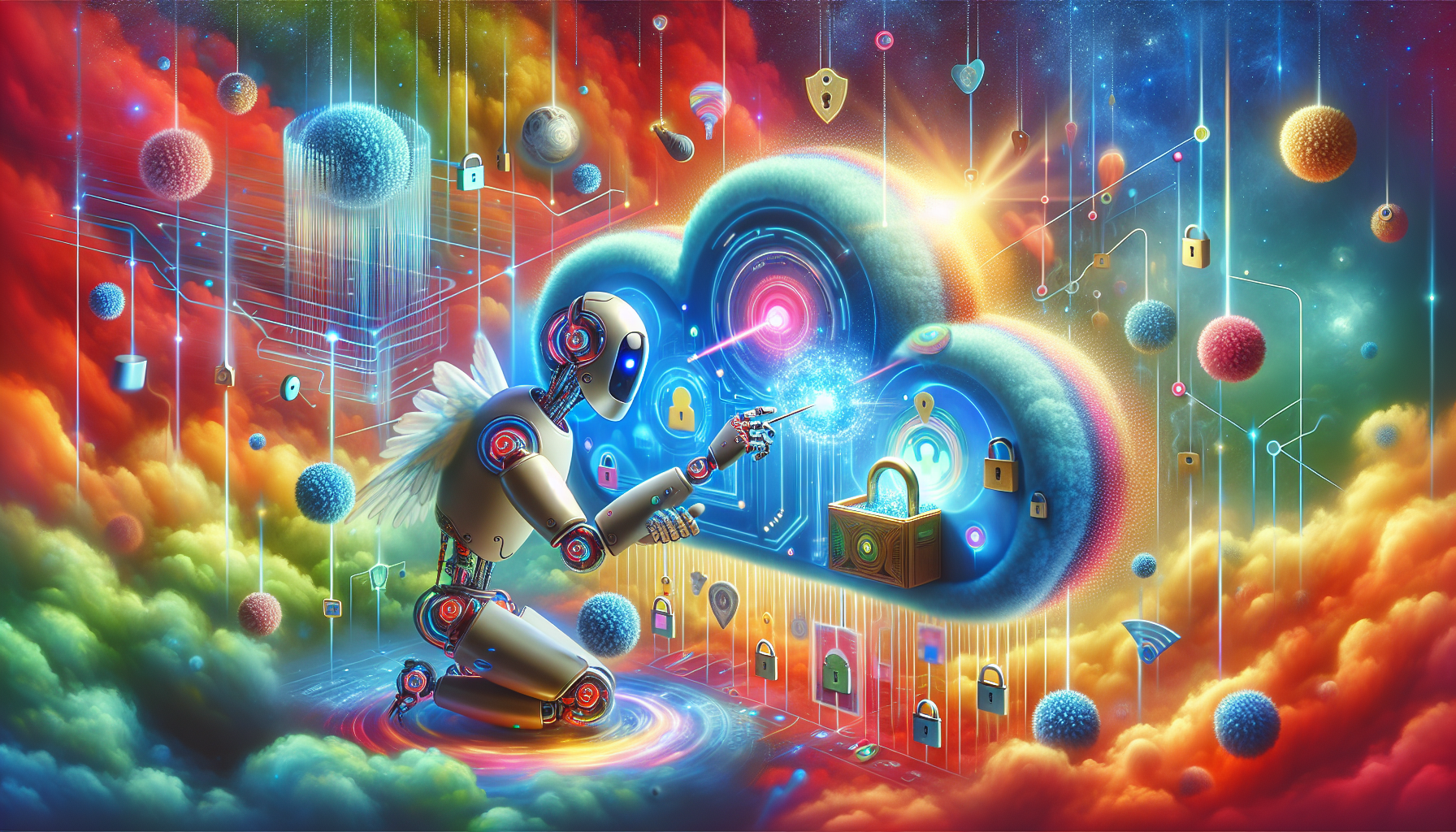
How to Extract Data from a Cloudflare-Protected Website
In this guide, we'll explore ethical and effective techniques to extract data from Cloudflare-protected websites.
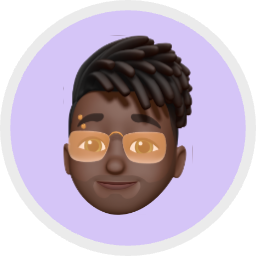
Lucas Mitchell
20-Feb-2025
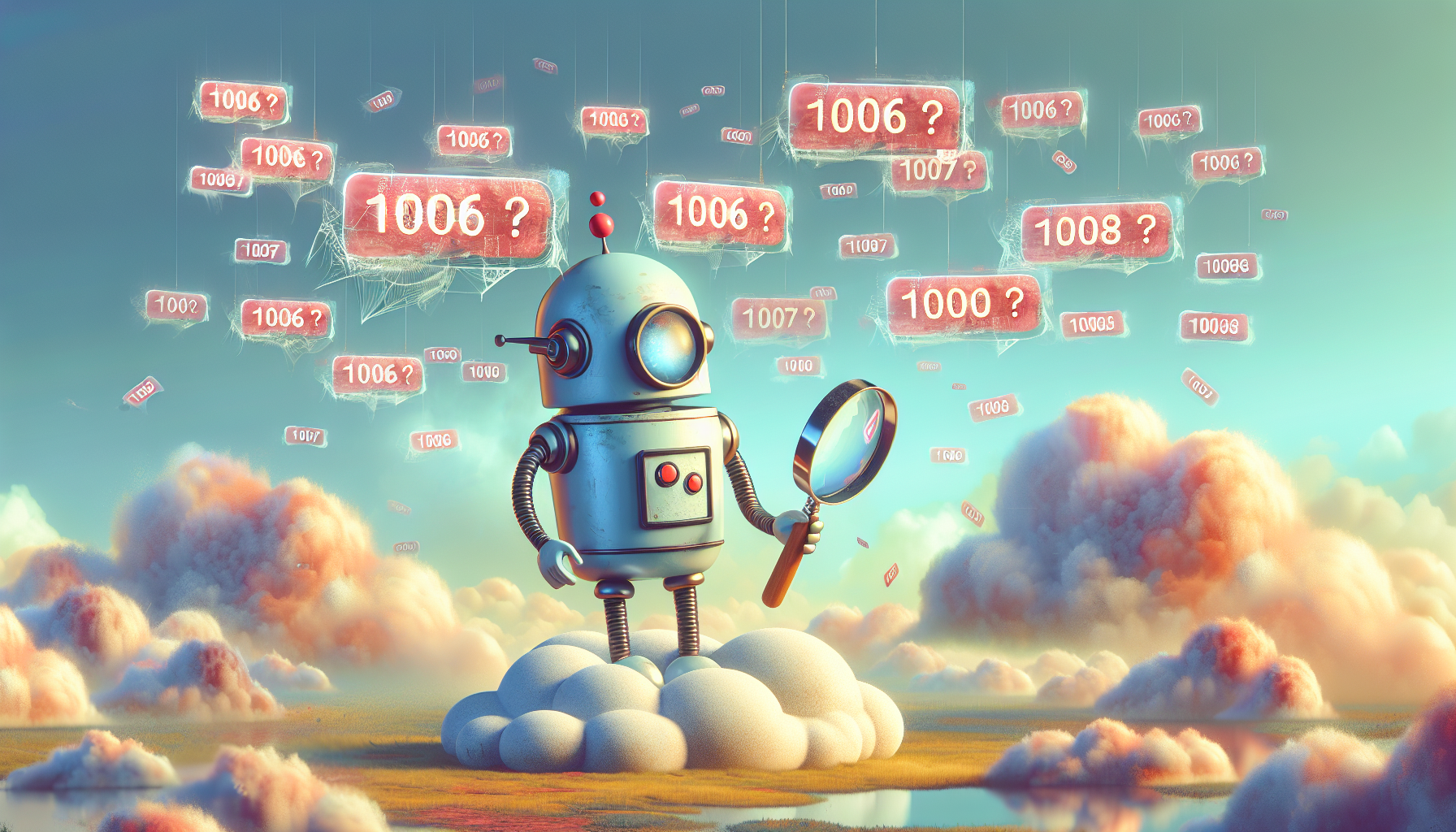
How to Fix Cloudflare Errors 1006, 1007, and 1008 Quickly
Cloudflare errors 1006, 1007, and 1008 can block your access due to suspicious or automated traffic. Learn quick fixes using premium proxies, user agent rotation, human behavior simulation, and IP address changes to overcome these roadblocks for smooth web scraping.
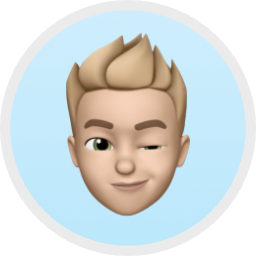
Ethan Collins
05-Feb-2025
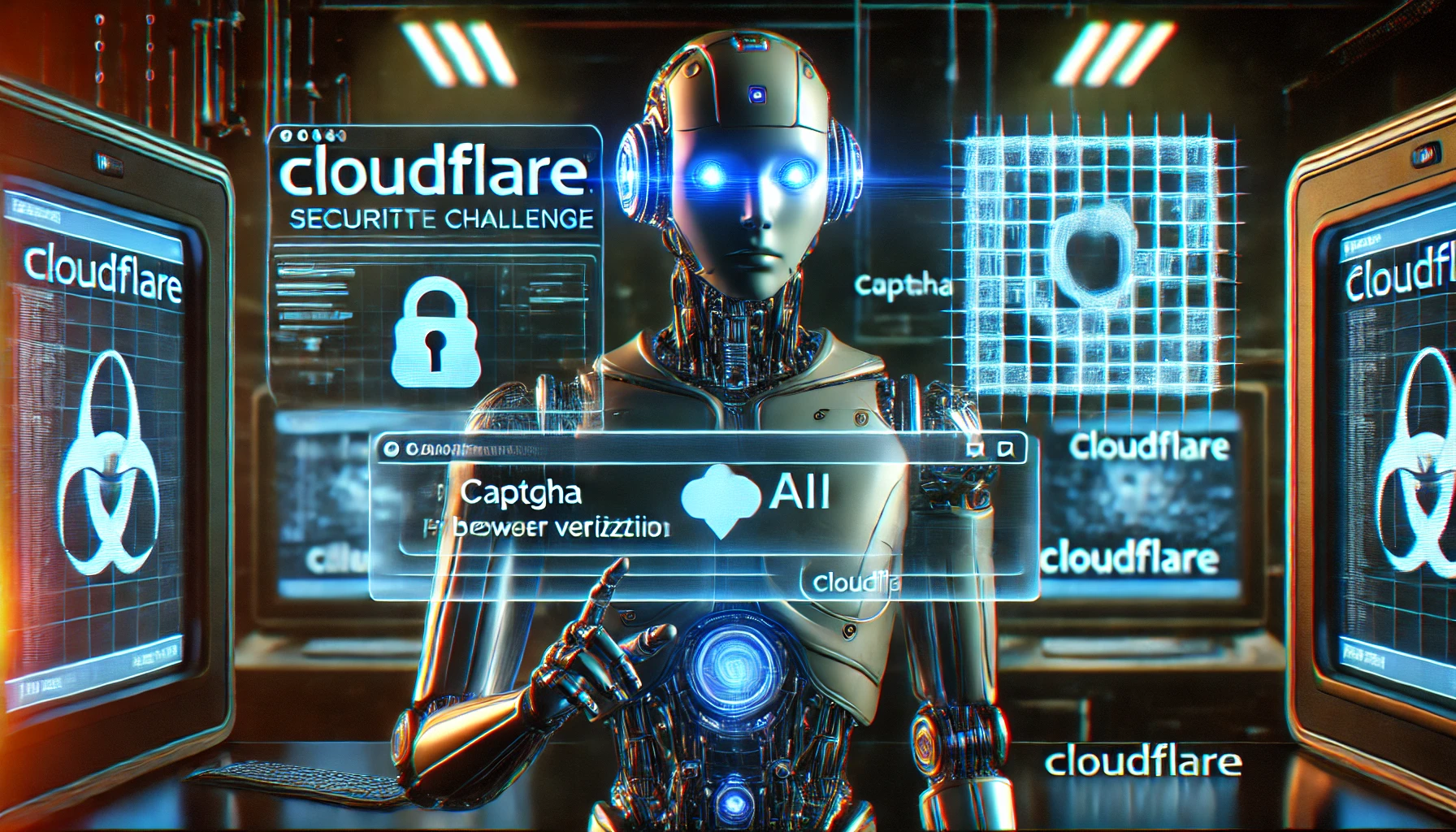
How to Bypass Cloudflare Challenge While Web Scraping in 2025
Learn how to bypass Cloudflare Challenge and Turnstile in 2025 for seamless web scraping. Discover Capsolver integration, TLS fingerprinting tips, and fixes for common errors to avoid CAPTCHA hell. Save time and scale your data extraction.
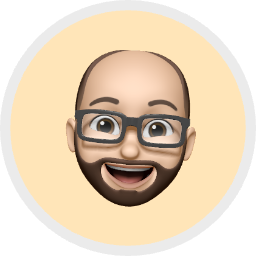
Aloísio Vítor
23-Jan-2025
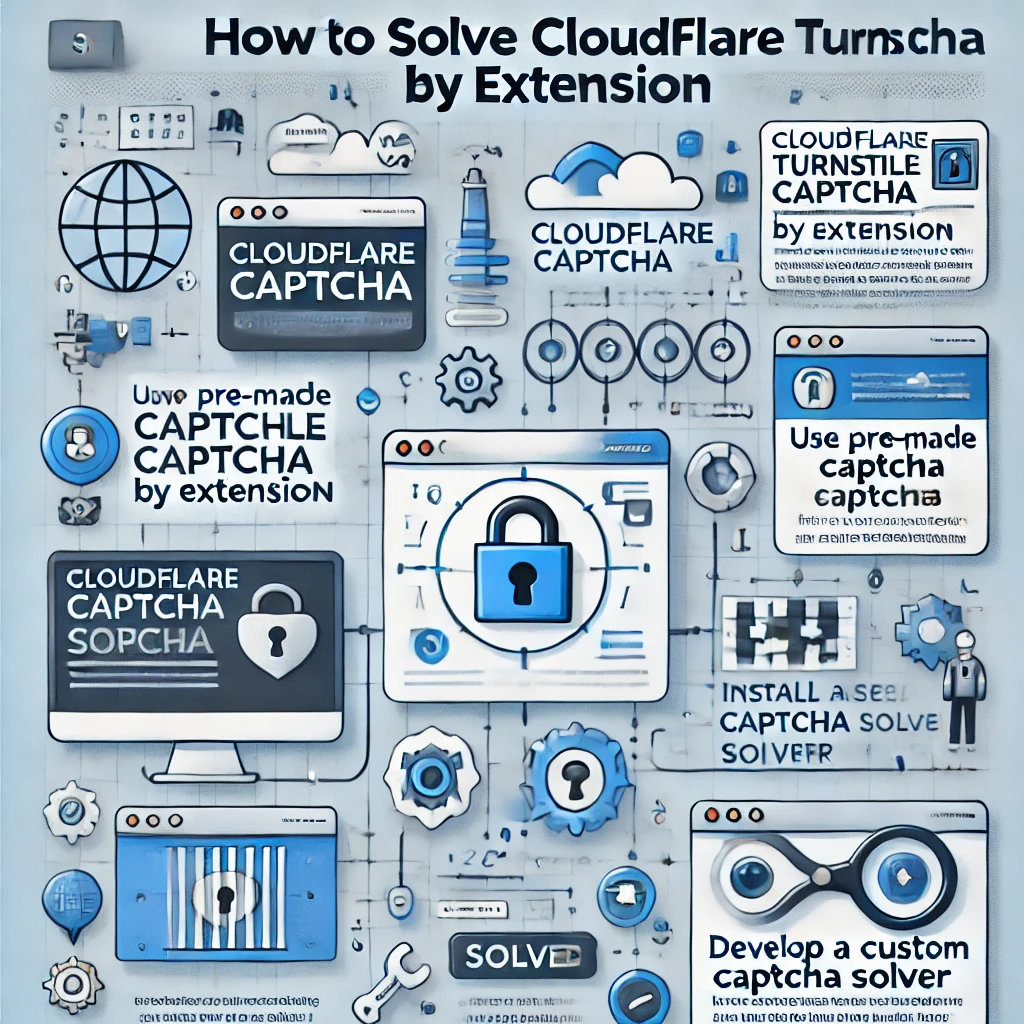
How to Solve Cloudflare Turnstile CAPTCHA by Extension
Learn how to bypass Cloudflare Turnstile CAPTCHA with Capsolver’s extension. Install guides for Chrome, Firefox, and automation tools like Puppeteer.
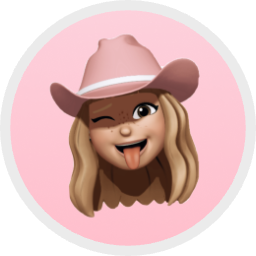
Adélia Cruz
23-Jan-2025
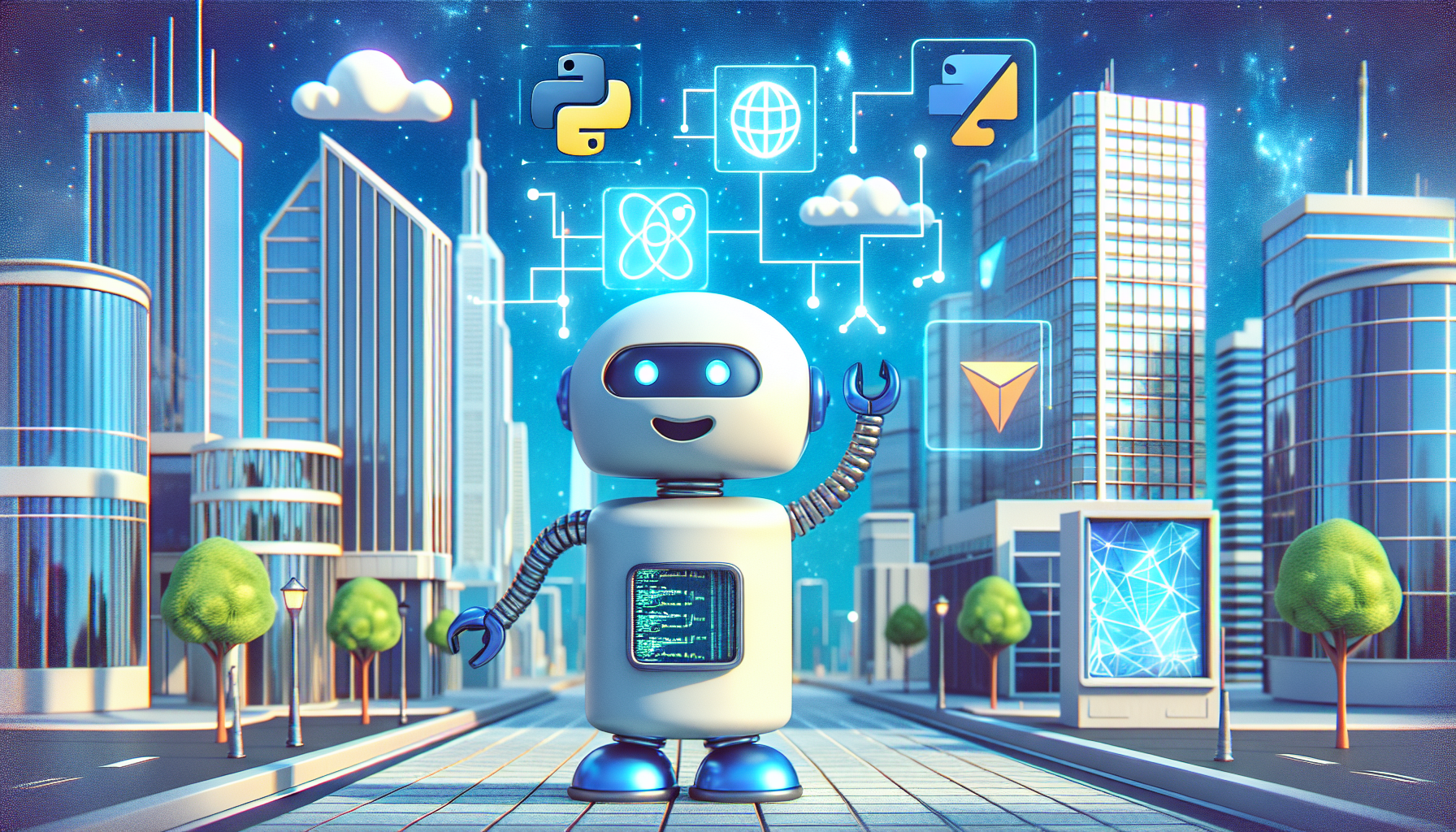
How to Solve Cloudflare by Using Python and Go in 2025
Will share insights on what Cloudflare Turnstile is, using Python and Go for these tasks, whether Turnstile can detect Python scrapers, and how to effectively it using solutions like CapSolver.
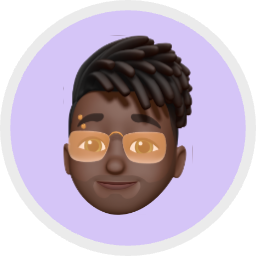
Lucas Mitchell
05-Nov-2024