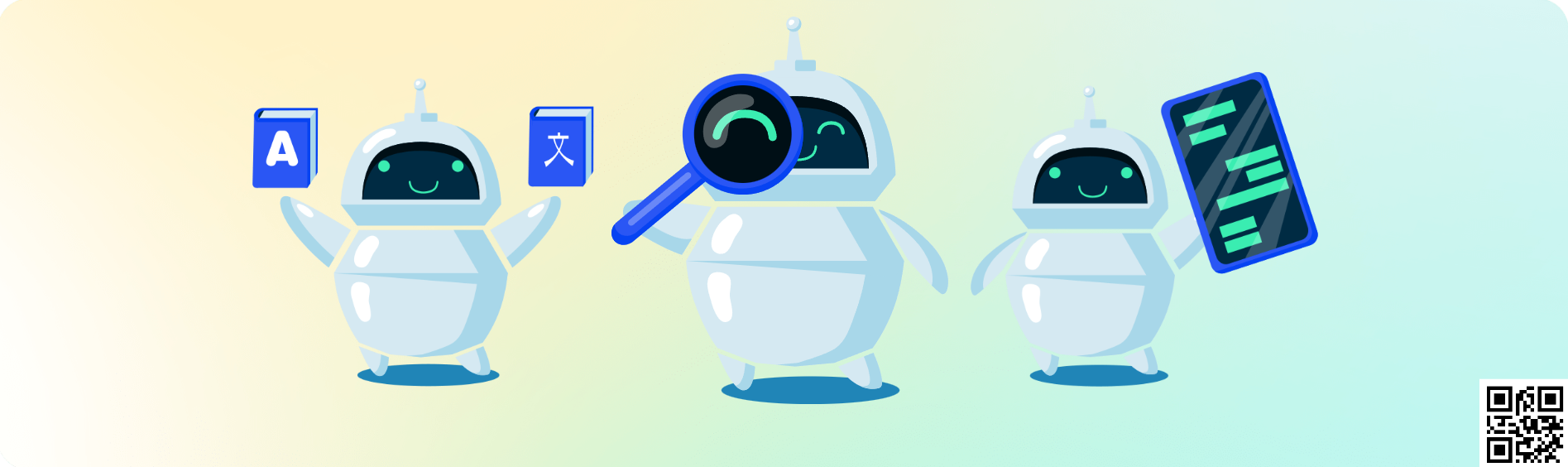
1. Installing Selenium and Required Components
- The installation of Selenium and browser drivers remains the same. Ensure Selenium and the necessary drivers for your browser (like ChromeDriver for Google Chrome or GeckoDriver for Firefox) are installed.
2. Configuring the Capsolver Extension
- Download and unzip the Capsolver extension as in the previous steps.
- Modify the settings in
./assets/config.json
to enable support for FunCaptcha. Specifically, setenabledForFunCaptcha
totrue
, and adjust thefunCaptchaMode
if necessary (similar toreCaptchaMode
andhCaptchaMode
).
Example configuration for FunCaptcha:
{
"enabledForFunCaptcha": true,
"funCaptchaMode": "token", // or "click", depending on your needs
// other settings remain as previously configured
}
3. Setting Up Selenium to Solve FunCaptcha with Capsolver Extension
- Setup Selenium WebDriver with the Capsolver extension loaded.
- The script will need to be tailored to interact with FunCaptcha elements on the target webpage.
Example Selenium script for FunCaptcha:
const { Builder, By, until } = require('selenium-webdriver');
const chrome = require('selenium-webdriver/chrome');
const path = require('path');
(async function solveCaptcha() {
let options = new chrome.Options();
options.addArguments(`--load-extension=${path.join(__dirname, 'CapSolver.Browser.Extension')}`);
let driver = await new Builder()
.forBrowser('chrome')
.setChromeOptions(options)
.build();
try {
await driver.get('https://site-with-funcaptcha.example');
// Locate the FunCaptcha frame or checkbox and interact accordingly
await driver.wait(until.elementLocated(By.css('selector-for-funcaptcha')), 10000);
await driver.findElement(By.css('selector-for-funcaptcha')).click();
// Add additional steps as per your requirement
} finally {
await driver.quit();
}
})();
In this script, replace 'selector-for-funcaptcha'
with the correct selector for the FunCaptcha element on the webpage you are working with.
By following these steps, you can configure Selenium with the Capsolver extension to automate the solving of FunCaptcha challenges.