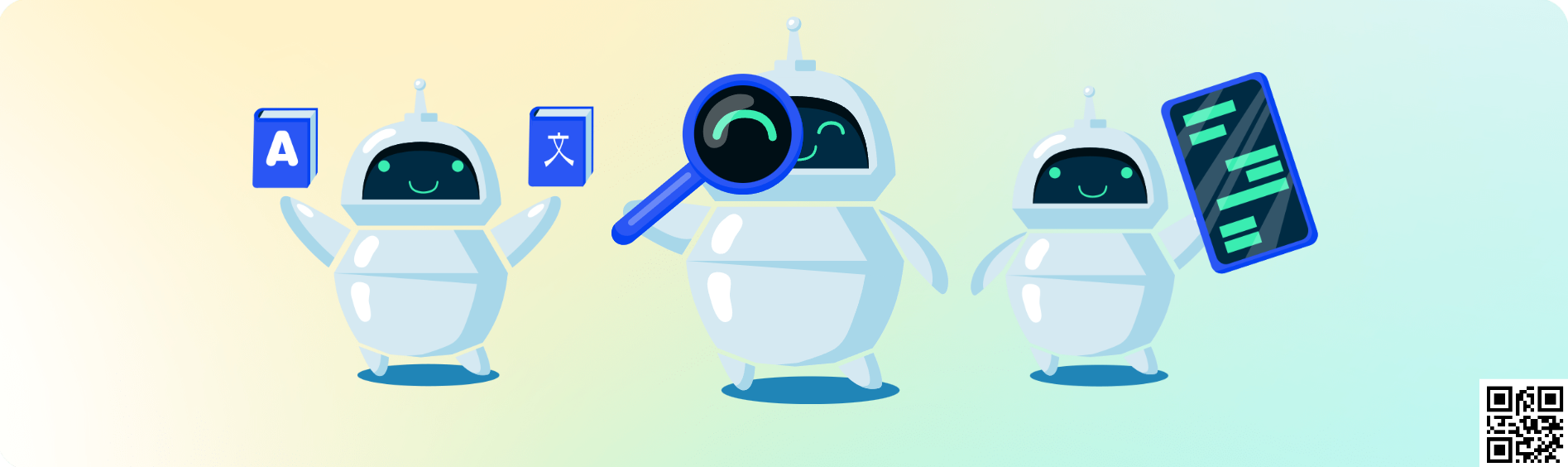
What is Cloudflare Turnstile
Cloudflare Turnstile is a free tool to replace CAPTCHAs
Turnstile delivers frustration-free, CAPTCHA-free web experiences to website visitors - with just a simple snippet of free code. Moreover, Turnstile stops abuse and confirms visitors are real without the data privacy concerns or awful user experience of CAPTCHAs.
How to identify Cloudflare Turnstile Captcha
- A non-interactive challenge.
Example: https://peet.ws/turnstile-test/non-interactive.html - A non-intrusive interactive challenge (such as clicking a button), if the visitor is a suspected bot.
Example: https://peet.ws/turnstile-test/managed.html - An invisible challenge to the browser.
Captcha don't appear on the website, but it's loaded in the html
Example:
https://peet.ws/turnstile-test/invisible.html
🛠️ Solving cloudflare turnstile captcha
⚙️ Prerequisites
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
pip install requests
👨💻 Step 2: Python Code for solve Cloudflare Turnstile Captcha
Here's a Python sample script to accomplish the task:
import time
import requests
CAPSOLVER_API_KEY = "api key"
PAGE_URL = "url"
WEBSITE_KEY = "site key"
def solvecf(metadata_action=None, metadata_cdata=None):
url = "https://api.capsolver.com/createTask"
task = {
"type": "AntiTurnstileTaskProxyLess",
"websiteURL": PAGE_URL,
"websiteKey": WEBSITE_KEY,
}
if metadata_action or metadata_cdata:
task["metadata"] = {}
if metadata_action:
task["metadata"]["action"] = metadata_action
if metadata_cdata:
task["metadata"]["cdata"] = metadata_cdata
data = {
"clientKey": CAPSOLVER_API_KEY,
"task": task
}
response_data = requests.post(url, json=data).json()
print(response_data)
return response_data['taskId']
def solutionGet(taskId):
url = "https://api.capsolver.com/getTaskResult"
status = ""
while status != "ready":
data = {"clientKey": CAPSOLVER_API_KEY, "taskId": taskId}
response_data = requests.post(url, json=data).json()
print(response_data)
status = response_data.get('status', '')
print(status)
if status == "ready":
return response_data['solution']
time.sleep(2)
def main():
taskId = solvecf()
solution = solutionGet(taskId)
if solution:
user_agent = solution['userAgent']
token = solution['token']
print("User_Agent:", user_agent)
print("Solved Turnstile Captcha, token:", token)
if __name__ == "__main__":
main()
⚠️ Change these variables
- CAPSOLVER_API_KEY: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve the CloudFlare Turnstile Captcha.
- WEBSITE_KEY: Replace with the site key of the website