How to solve reCaptcha v3 with Python
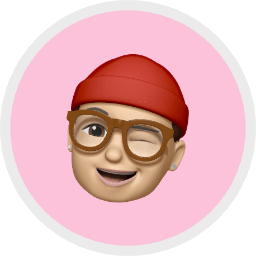
Sora Fujimoto
AI Solutions Architect
15-Sep-2023
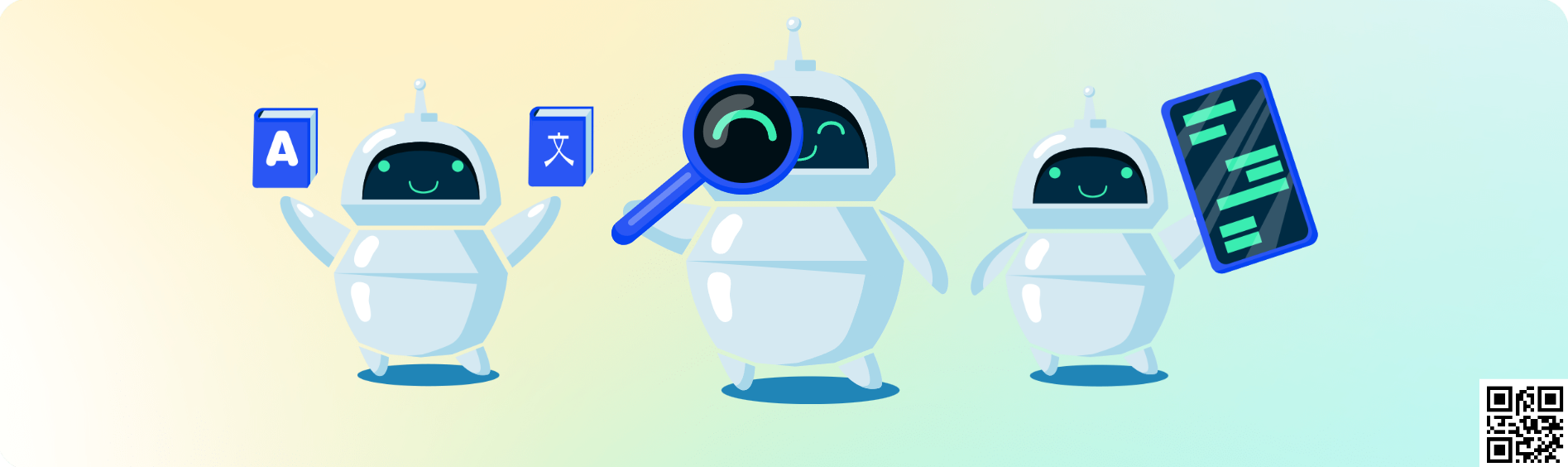
⚙️ Prerequisites
- Python installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
python
pip install capsolver
👨💻 Step 2: Python Code for solve reCaptcha v3 and get 0.7-0.9 score
Here's a Python sample script to accomplish the task:
python
import json
import os
import capsolver
from urllib.parse import urlparse
# Change these values
capsolver.api_key = "YourApiKey"
PAGE_URL = ""
PAGE_KEY = ""
PAGE_ACTION = ""
def solve_recaptcha_v3(url,key,pageAction):
solution = capsolver.solve({
"type": "ReCaptchaV3TaskProxyLess",
"websiteURL": url,
"websiteKey":key,
"pageAction":pageAction
})
return solution
def main():
print("Solving reCaptcha v3")
solution = solve_recaptcha_v3(PAGE_URL, PAGE_KEY, PAGE_ACTION)
print("Solution: ", solution)
if __name__ == "__main__":
main()
⚠️ Change these variables
- capsolver.api_key: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve the reCaptcha v3.
- PAGE_KEY: Update with the specific key of the site with recaptcha.
- PAGE_ACTION: Replace with the pageAction of the page. You can read this blog
👀 More information
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
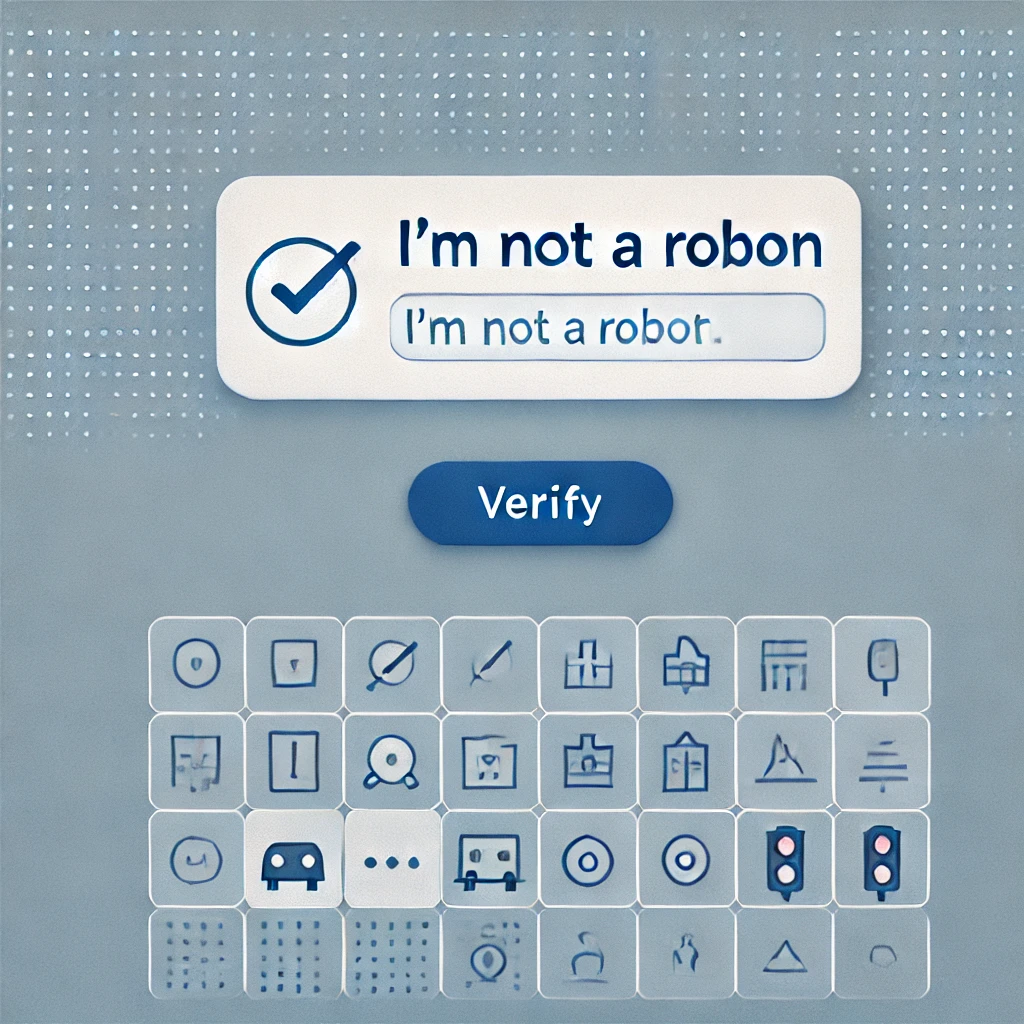
Top 5 Captcha Solvers for reCAPTCHA Recognition in 2025
Explore 2025's top 5 CAPTCHA solvers, including AI-driven CapSolver for fast reCAPTCHA recognition. Compare speed, pricing, and accuracy here
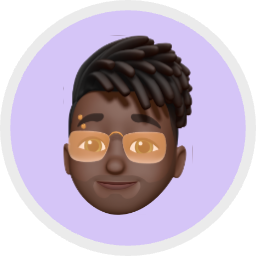
Lucas Mitchell
23-Jan-2025
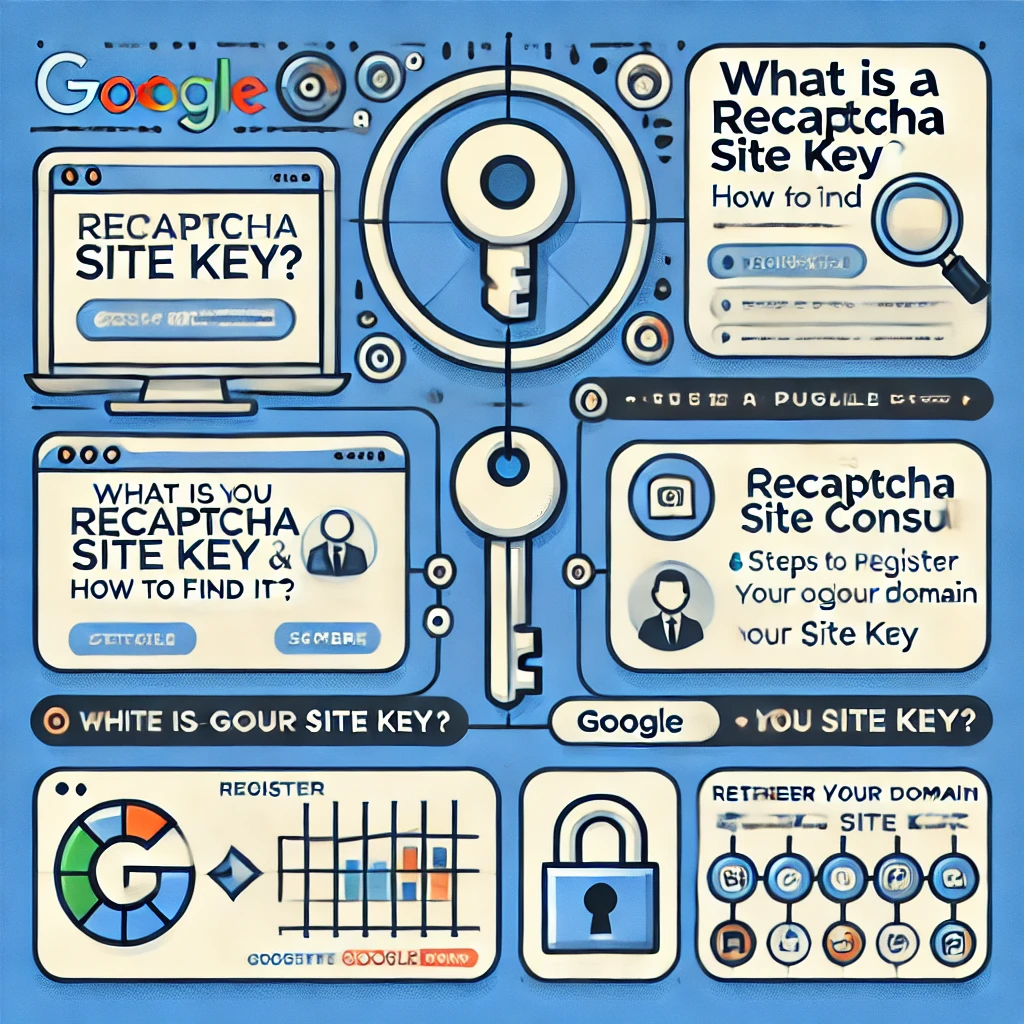
What is a reCAPTCHA Site Key and How to Find It?
Learn how to find a reCAPTCHA Site Key manually or with tools like Capsolver. Fix common issues and automate CAPTCHA solving for developers and web scraping.
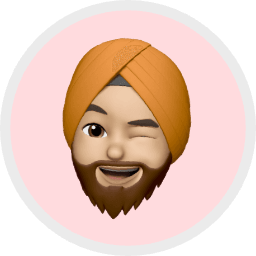
Rajinder Singh
23-Jan-2025
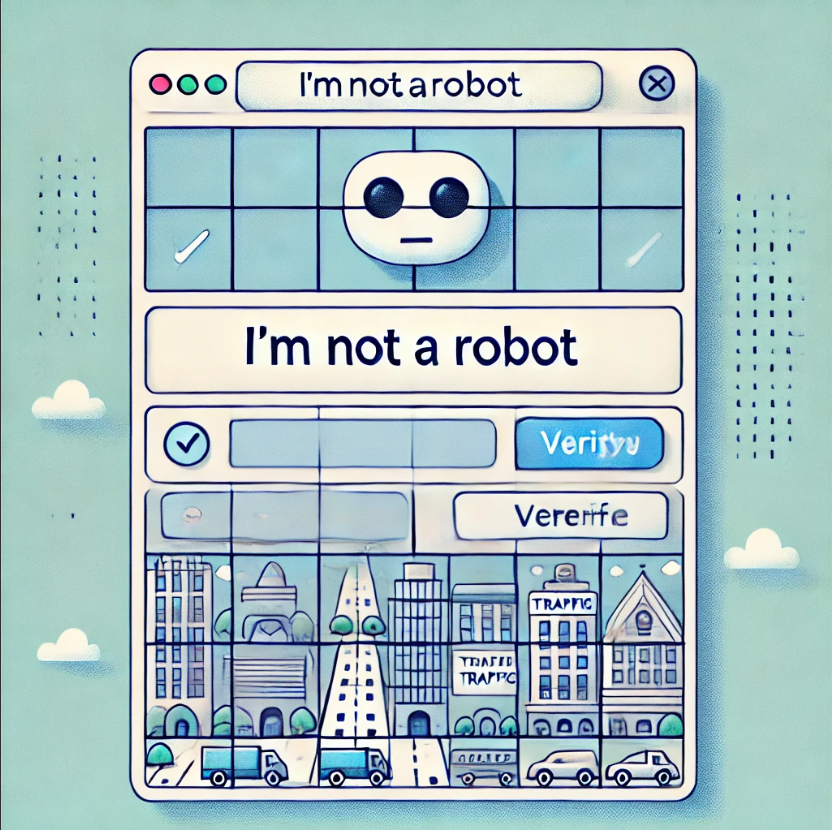
What Is reCAPTCHA Recognition? A Beginner's Guide
Struggling with reCAPTCHA image grids? Discover how Capsolver's AI-powered recognition solves 'Select all ' challenges instantly. Learn API integration, browser extensions, and pro tips to automate CAPTCHA solving with 95%+ accuracy
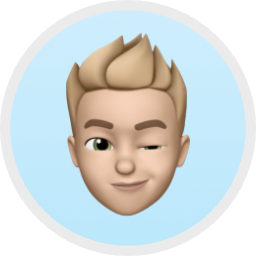
Ethan Collins
23-Jan-2025
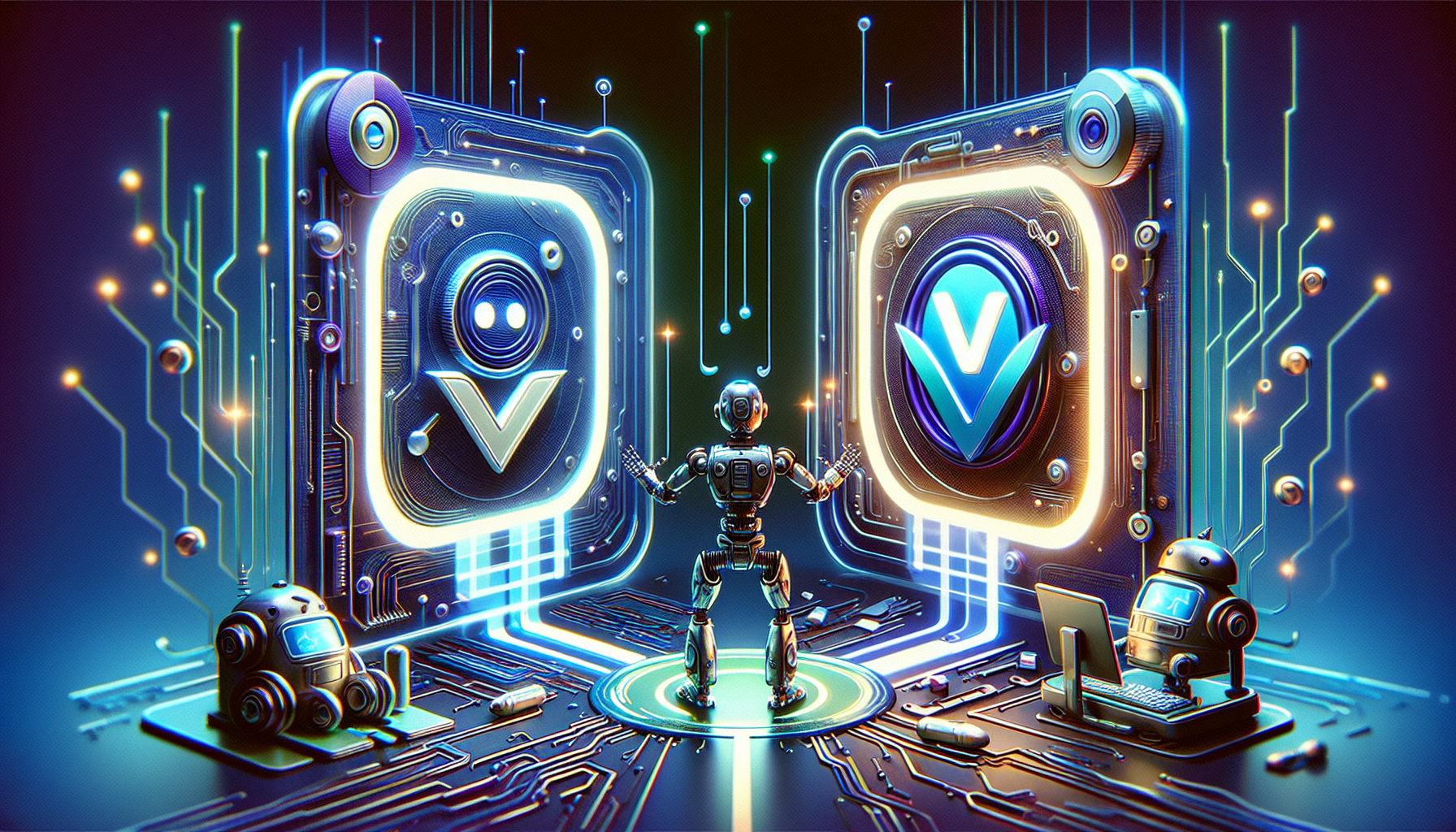
What is the best reCAPTCHA v2 and v3 Solver while web scraping in 2025
In 2025, with the heightened sophistication of anti-bot systems, finding reliable reCAPTCHA solvers has become critical for successful data extraction.
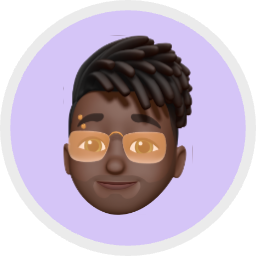
Lucas Mitchell
17-Jan-2025
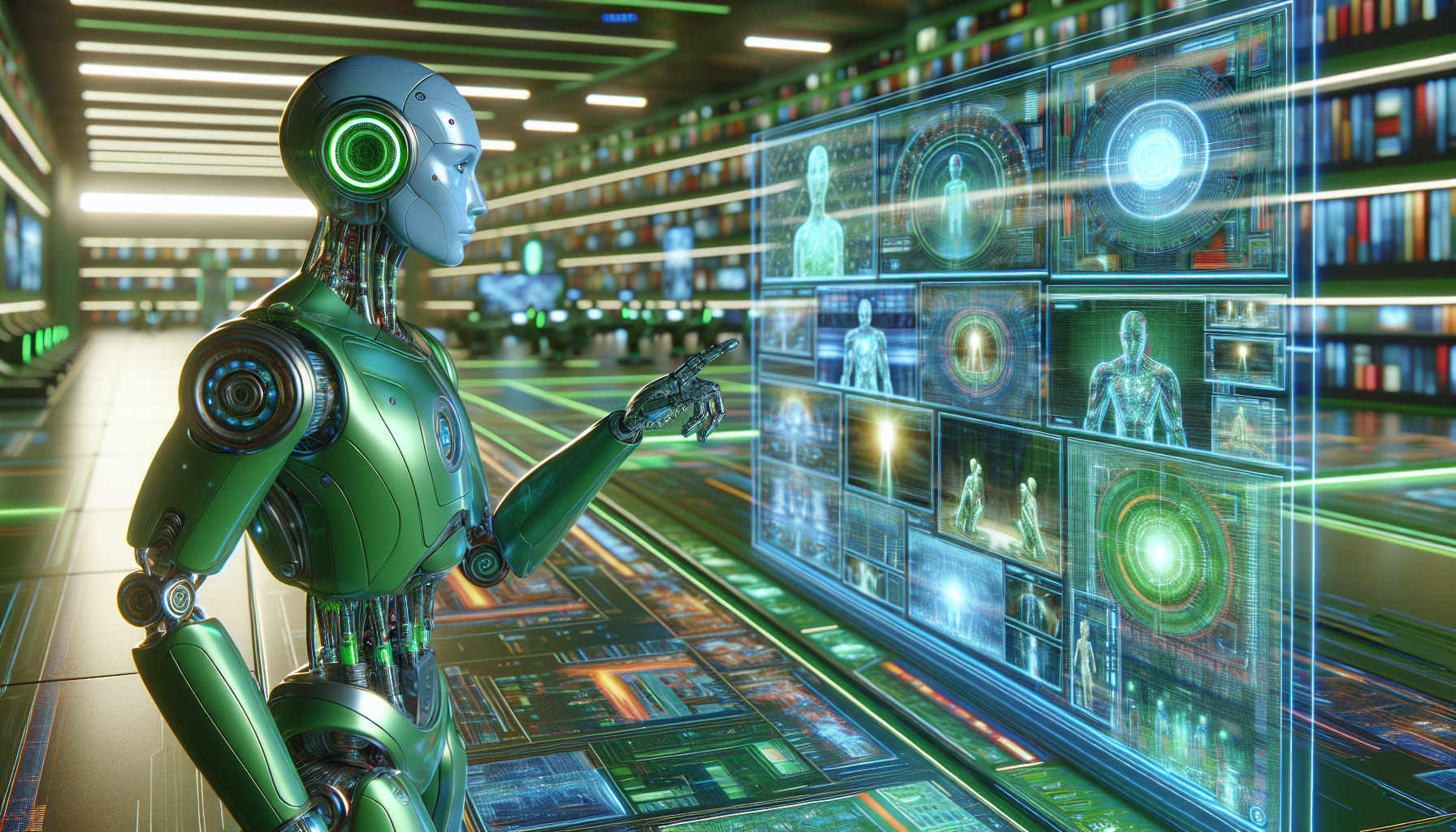
Solving reCAPTCHA with AI Recognition in 2025
Explore how AI is transforming reCAPTCHA-solving, CapSolver's solutions, and the evolving landscape of CAPTCHA security in 2025.
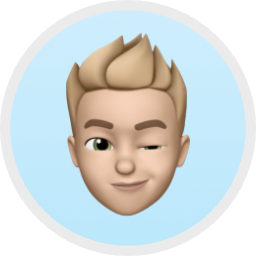
Ethan Collins
11-Nov-2024
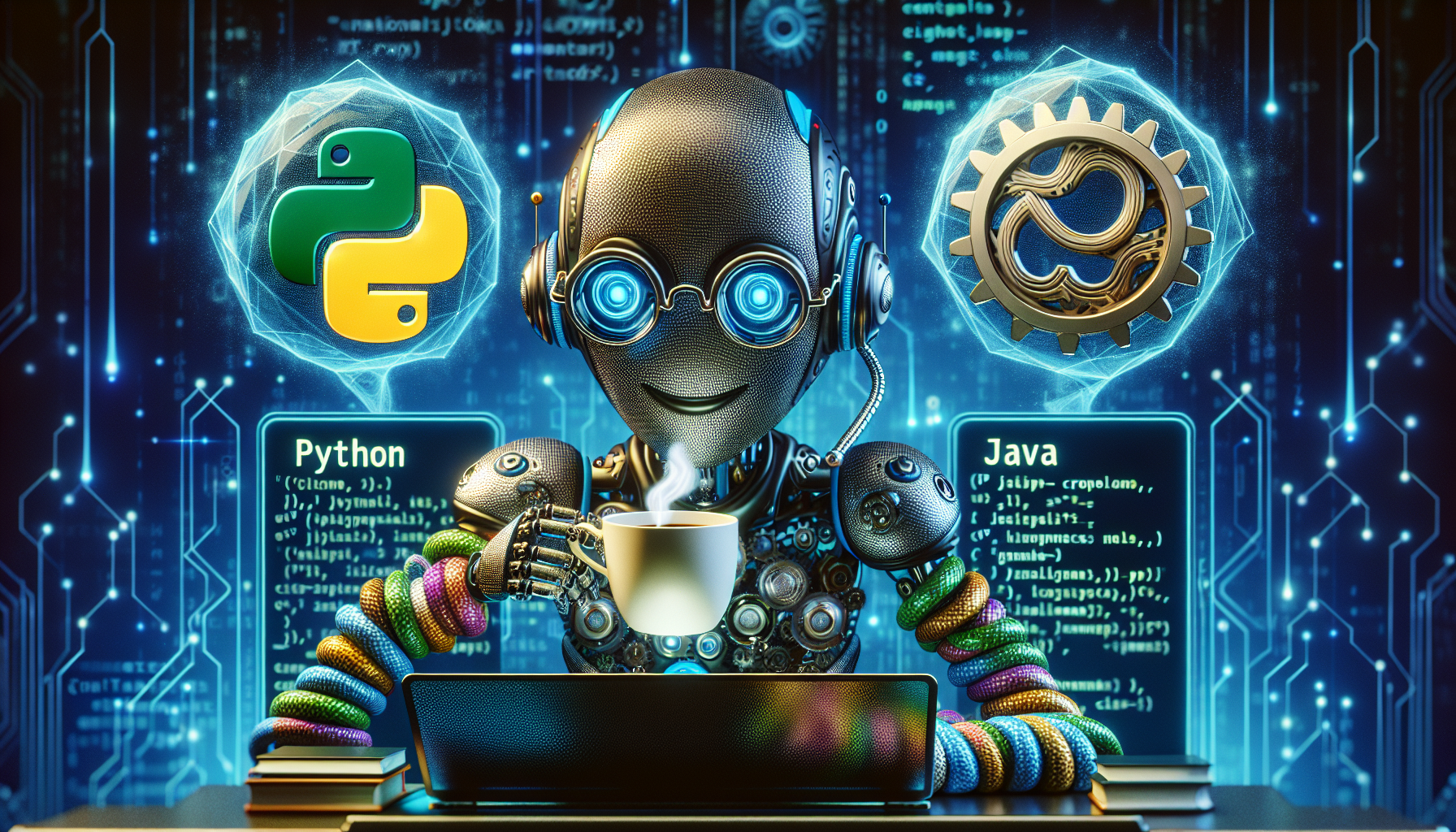
Solving reCAPTCHA Using Python, Java, and C++
What to know how to successfully solve reCAPTCHA using three powerful programming languages: Python, Java, and C++ in one blog? Get in!
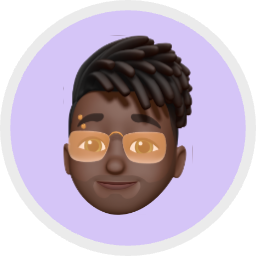
Lucas Mitchell
25-Oct-2024