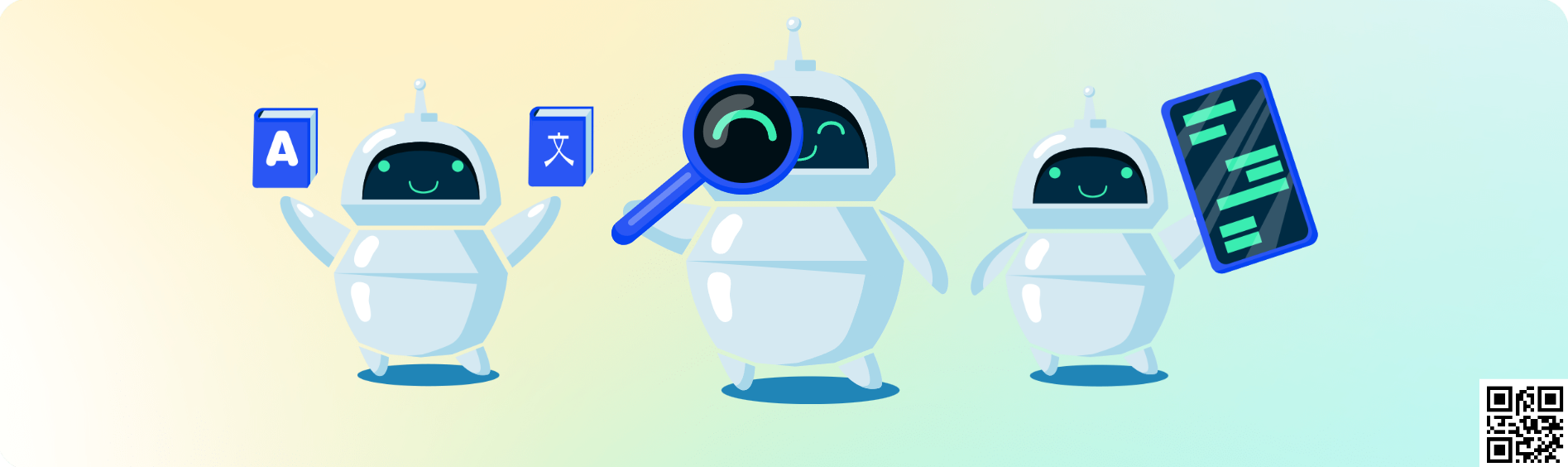
⚙️ Prerequisites
- Node.JS Installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
npm install axios
👨💻 Step 2: Node.JS Code for solve reCaptcha v3 and get 0.7-0.9 score
Here's a Node.JS sample script to accomplish the task:
const PAGE_URL = "https://antcpt.com/score_detector";
const PAGE_KEY = "6LcR_okUAAAAAPYrPe-HK_0RULO1aZM15ENyM-Mf";
const PAGE_ACTION = "homepage";
const CAPSOLVER_KEY = "YourKey"
async function createTask(url, key, pageAction) {
try {
// Define the API endpoint and payload as per the service documentation.
const apiUrl = "https://api.capsolver.com/createTask";
const payload = {
clientKey: CAPSOLVER_KEY,
task: {
type: "ReCaptchaV3TaskProxyLess",
websiteURL: url,
websiteKey: key,
pageAction: pageAction
}
};
const headers = {
'Content-Type': 'application/json',
};
const response = await axios.post(apiUrl, payload, { headers });
return response.data.taskId;
} catch (error) {
console.error("Error creating CAPTCHA task: ", error);
throw error;
}
}
async function getTaskResult(taskId) {
try {
const apiUrl = "https://api.capsolver.com/getTaskResult";
const payload = {
clientKey: CAPSOLVER_KEY,
taskId: taskId,
};
const headers = {
'Content-Type': 'application/json',
};
let result;
do {
const response = await axios.post(apiUrl, payload, { headers });
result = response.data;
if (result.status === "ready") {
return result.solution;
}
await new Promise(resolve => setTimeout(resolve, 5000)); // wait 5 seconds before retrying
} while (true);
} catch (error) {
console.error("Error getting CAPTCHA result: ", error);
throw error;
}
}
function setSessionHeaders() {
return {
'cache-control': 'max-age=0',
'sec-ch-ua': '"Not/A)Brand";v="99", "Google Chrome";v="107", "Chromium";v="107"',
'sec-ch-ua-mobile': '?0',
'sec-ch-ua-platform': 'Windows',
'upgrade-insecure-requests': '1',
'user-agent': 'Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/108.0.0.0 Safari/537.36',
'accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7',
'sec-fetch-site': 'same-origin',
'sec-fetch-mode': 'navigate',
'sec-fetch-user': '?1',
'sec-fetch-dest': 'document',
'accept-encoding': 'gzip, deflate',
'accept-language': 'en,fr-FR;q=0.9,fr;q=0.8,en-US;q=0.7',
};
}
async function main() {
const headers = setSessionHeaders();
console.log("Creating CAPTCHA task...");
const taskId = await createTask(PAGE_URL, PAGE_KEY, PAGE_ACTION);
console.log(`Task ID: ${taskId}`);
console.log("Retrieving CAPTCHA result...");
const solution = await getTaskResult(taskId);
const token = solution.gRecaptchaResponse;
console.log(`Token Solution ${token}`);
const res = await axios.post("https://antcpt.com/score_detector/verify.php", { "g-recaptcha-response": token }, { headers });
const response = res.data;
console.log(`Score: ${response.score}`);
}
main().catch(err => {
console.error(err);
});
⚠️ Change these variables
- capsolver.api_key: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve the reCaptcha v3.
- PAGE_KEY: Update with the specific key of the site with recaptcha.
- PAGE_ACTION: Replace with the pageAction of the page. You can read this blog