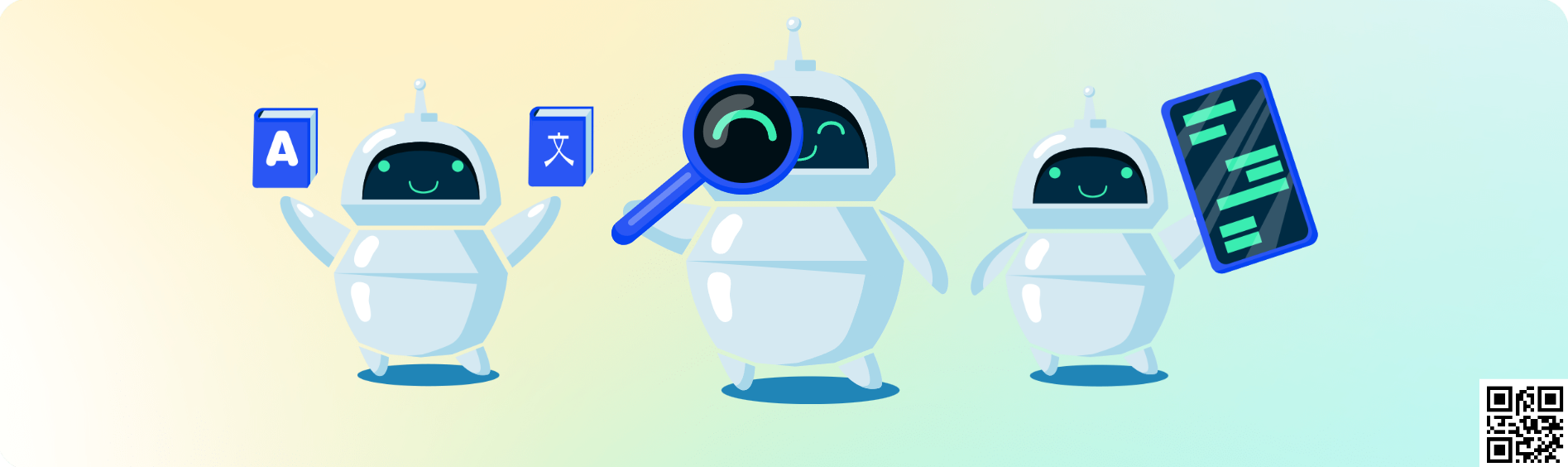
⚙️ Prerequisites
- Proxy
- Node.JS installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
npm install axios
👨💻 Node.JS Code for solve DataDome with proxy
Here's a Node.JS sample script to accomplish the task:
const axios = require('axios');
const CLIENT_KEY = "your capsolver.com api key"; // Replace with your CAPSOLVER API Key
const headers = {
"cache-control": "max-age=0",
"sec-ch-ua": '"Not/A)Brand";v="99", "Google Chrome";v="107", "Chromium";v="107"',
"sec-ch-ua-mobile": "?0",
"sec-ch-ua-platform": "Windows",
"upgrade-insecure-requests": "1",
"user-agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"accept": "*/*",
"sec-fetch-site": "same-origin",
"sec-fetch-mode": "navigate",
"sec-fetch-user": "?1",
"sec-fetch-dest": "document",
"accept-encoding": "gzip, deflate, br",
"accept-language": "en,fr-FR;q=0.9,fr;q=0.8,en-US;q=0.7"
};
async function createTask(payload) {
try {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: CLIENT_KEY,
task: payload
});
return res.data;
} catch (error) {
console.error(error);
}
}
async function getTaskResult(taskId) {
try {
success = false;
while(success == false){
await sleep(1000);
console.log("Getting task result for task ID: " + taskId);
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: CLIENT_KEY,
taskId: taskId
});
if( res.data.status == "ready") {
success = true;
return res.data;
}
}
} catch (error) {
console.error(error);
return null;
}
}
async function solveDatadome(CaptchaURL, UserAgent, proxy) {
const taskPayload = {
type: "DatadomeSliderTask",
captchaUrl: CaptchaURL,
userAgent: UserAgent,
proxy: proxy
};
const taskData = await createTask(taskPayload);
return await getTaskResult(taskData.taskId);
}
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function main() {
try {
//Obtain these values for create the geoCaptcha URL. Sometimes datadome provide the url directly and other times the values.
//In this case, they return the values, so we need to make the geo captcha url
let initialCid = "initial cid value"
let cid = "value cid "
let referer = "page url referer value"
let hash = "hash value"
let t = "t value"
let s = "s value"
let e = "e value"
//Capsolver values
let userAgent = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/119.0.0.0 Safari/537.36" //This user agent, please check the documentation to get latest user agent https://docs.capsolver.com/guide/antibots/datadome.html
let proxy = "your proxy"
let geoCaptchaURL = `https://geo.captcha-delivery.com/captcha/?initialCid=${initialCid}&cid=${cid}&referer=${encodeURIComponent(referer)}&hash=${hash}&t=${t}&s=${s}&e=${e}`;
// Be sure to obtain always a different geo captcha url, can't be the same
let solution = await solveDatadome(geoCaptchaURL,userAgent, proxy )
console.log(solution)
}
catch (error) {
console.error(`Error: ${error}`);
}
}
main();
⚠️ Change these variables
- PROXY: Change to your proxy
- CLIENT_KEY: Obtain your API key from the Capsolver Dashboard.
- captchaUrl: Replace with the URL of the datadome captcha website
- userAgent: Replace with the userAgent that is on the documentation https://docs.capsolver.com/guide/antibots/datadome.html