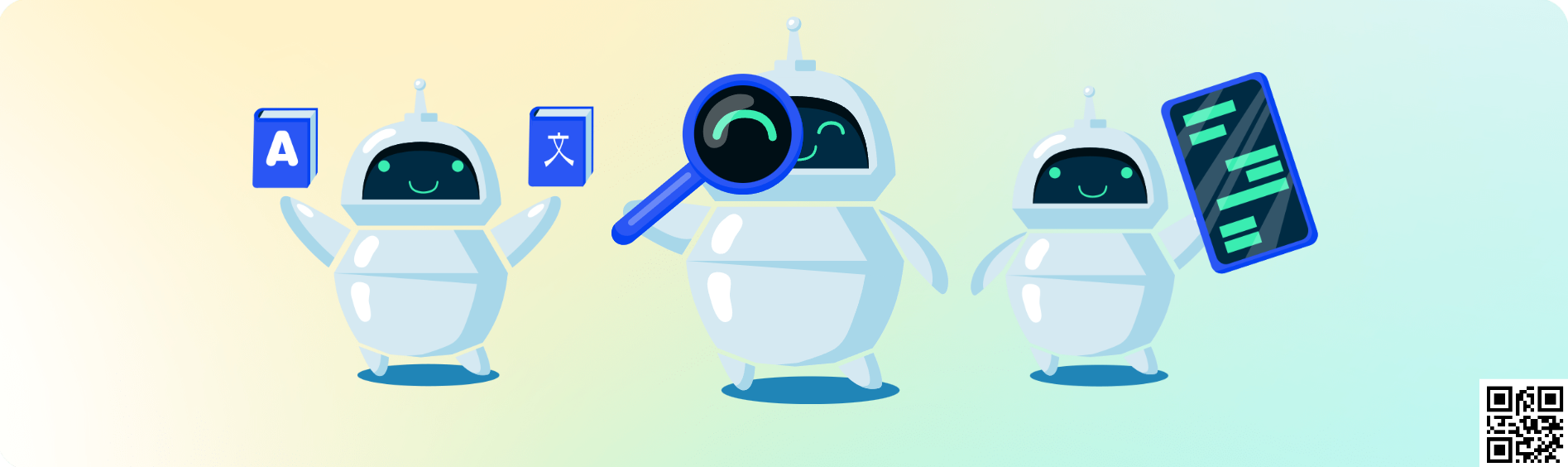
⚙️ Prerequisites
- A working proxy
- NodeJS installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
npm install axios
👨💻 Node JS Code for solve Datadome with Proxy
Here's a NodeJS sample script to accomplish the task:
const axios = require('axios');
// Constants
const CAPSOLVER_API_KEY = "capsolver api key";
const PROXY = "your proxy";
//Get the user Agent from documentation
const USER_AGENT = "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/119.0.0.0 Safari/537.36";
const USER_AGENT_FOR_TRIGGER_DATADOME = "Mozilla/5.0";
const SITE_URL_WITH_DATADOME = "page with datadome";
// Headers Template
const headersTemplate = (triggerDatadome = false) => ({
'Accept': 'text/html,application/xhtml+xml,application/xml;q=0.9,image/avif,image/webp,image/apng,*/*;q=0.8,application/signed-exchange;v=b3;q=0.7',
'Accept-Encoding': 'gzip, deflate, br',
'Accept-Language': 'en,en-US;q=0.9',
'Cache-Control': 'max-age=0',
'DNT': '1',
'Sec-Ch-Device-Memory': '8',
'Sec-Ch-Ua': '"Google Chrome";v="119", "Chromium";v="119", "Not?A_Brand";v="24"',
'Sec-Ch-Ua-Arch': '"x86"',
'Sec-Ch-Ua-Full-Version-List': '"Google Chrome";v="119.0.6045.200", "Chromium";v="119.0.6045.200", "Not?A_Brand";v="24.0.0.0"',
'Sec-Ch-Ua-Mobile': '?0',
'Sec-Ch-Ua-Model': '""',
'Sec-Ch-Ua-Platform': '"Windows"',
'Sec-Fetch-Dest': 'document',
'Sec-Fetch-Mode': 'navigate',
'Sec-Fetch-Site': 'same-origin',
'Sec-Fetch-User': '?1',
'Sec-Gpc': '1',
'Upgrade-Insecure-Requests': '1',
'User-Agent': triggerDatadome ? USER_AGENT_FOR_TRIGGER_DATADOME : USER_AGENT,
'Referer': SITE_URL_WITH_DATADOME
});
// Helper functions
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function createTask(payload) {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: CAPSOLVER_API_KEY,
task: payload
});
console.log("Created capsolver task ")
return res.data;
}
async function getTaskResult(taskId) {
let success = false;
while (!success) {
await sleep(1000);
console.log("Getting results " + taskId)
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: CAPSOLVER_API_KEY,
taskId: taskId
});
console.log(res)
if (res.data.status === "ready") {
success = true;
return res.data;
}
}
}
async function solveDataDome(captchaUrl) {
const taskPayload = {
type: "DatadomeSliderTask",
websiteURL: SITE_URL_WITH_DATADOME,
captchaUrl: captchaUrl,
userAgent: USER_AGENT,
proxy: PROXY
};
const taskData = await createTask(taskPayload);
return getTaskResult(taskData.taskId);
}
function extractCaptchaUrl(response) {
if (!response.data.includes('https://geo.captcha-delivery.com/captcha/?initialCid=') &&
!response.data.includes('https://geo.captcha-delivery.com/interstitial/?initialCid=')) {
if (response.data.includes('dd=')) {
let ddStr = response.data.split('dd=')[1].split('</script')[0];
const dd = JSON.parse(ddStr.replace(/'/g, '"'));
const cid = response.headers['set-cookie'][0].split('datadome=')[1].split(';')[0];
return `https://geo.captcha-delivery.com/captcha/?initialCid=${dd.cid}&hash=${dd.hsh}&cid=${cid}&t=${dd.t}&referer=${encodeURIComponent(SITE_URL_WITH_DATADOME)}&s=${dd.s}&e=${dd.e}`;
}
} else {
const possiblePaths = ["/captcha/", "/interstitial/"];
let dataToSearch = typeof response.data === 'string' ? response.data : response.data.url;
if (!dataToSearch) {
console.error('response.data is not in the expected format');
return null;
}
for (const path of possiblePaths) {
const startIndex = dataToSearch.indexOf(`https://geo.captcha-delivery.com${path}`);
if (startIndex !== -1) {
const endIndex = dataToSearch.indexOf('"', startIndex);
return endIndex !== -1 ? dataToSearch.substring(startIndex, endIndex) : dataToSearch.substring(startIndex);
}
}
}
return "Not DataDome Captcha / Challenge found";
}
async function getRegisterPage(cookie, triggerDatadome = false) {
const customHeaders = headersTemplate(triggerDatadome);
if (cookie) {
customHeaders['Cookie'] = `datadome=${cookie}`;
}
try {
const response = await axios.get(SITE_URL_WITH_DATADOME, { headers: customHeaders });
return response;
} catch (error) {
if (axios.isAxiosError(error) && error.response && error.response.status === 403) {
console.log('Encountered a 403 error, but it is being passed to extractCaptchaUrl.');
return error.response;
} else {
console.error('Error in getRegisterPage:', error);
return null;
}
}
}
async function testRegisterPage() {
const response = await getRegisterPage("", true);
if (response) {
const captchaUrl = extractCaptchaUrl(response);
if (captchaUrl && captchaUrl.includes('geo.captcha')) {
const captchaSolution = await solveDataDome(captchaUrl);
if (captchaSolution) {
let cookie_datadome = captchaSolution.solution.cookie.split(';')[0].split('=')[1];
const response2 = await getRegisterPage(cookie_datadome);
console.log(response2 && response2.status);
}
} else {
console.log("Captcha URL not found or error occurred.");
}
}
}
testRegisterPage();
⚠️ Change these variables
- PROXY: Update with your proxy details. The format should be http://username:password@ip:port.
- CAPSOLVER_API_KEY: Obtain your API key from the Capsolver Dashboard.
- SITE_URL_WITH_DATADOME: Replace with the URL of the website for which you wish to solve datadome captcha
- USER_AGENT: Obtain the latest user agent from the documentation.