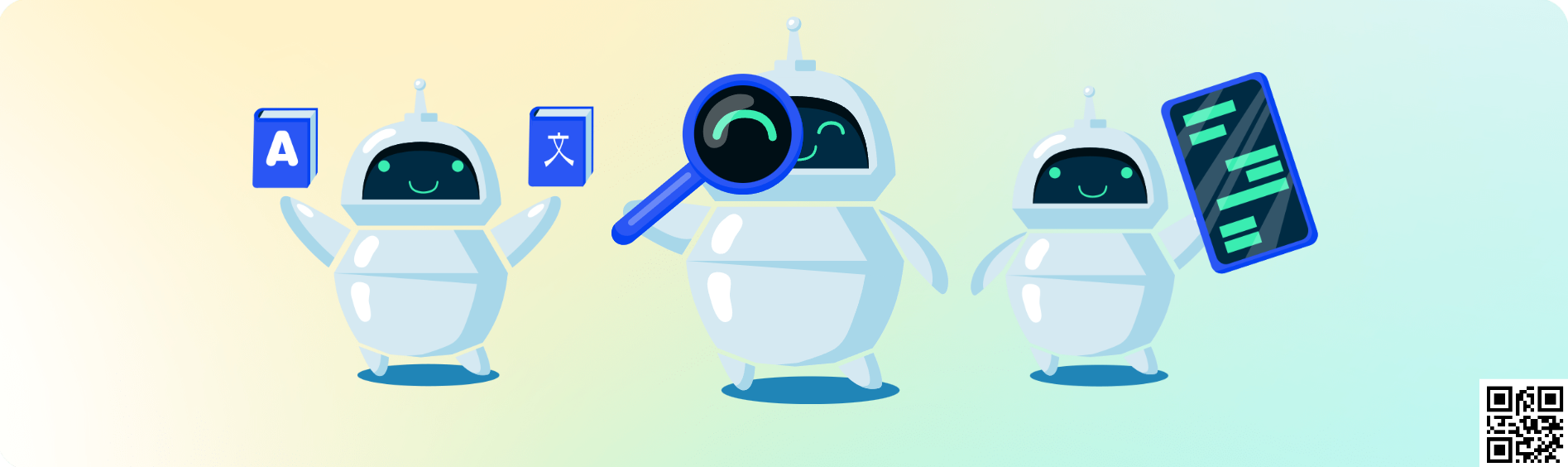
⚙️ Prerequisites
- Proxy (Optional)
- Node.JS installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
npm install axios
👨💻 Node.JS Code for solve AWS Captcha with proxy
Here's a Node.JS sample script to accomplish the task:
const axios = require('axios');
const cheerio = require('cheerio');
const { URL } = require('url');
const PROXY = "your proxy" // Replace with your proxy
const PAGE_URL = "https://norway-meetup.aws.wslab.no/"; // Replace with your Website
const CLIENT_KEY = "Capsolver Api key"; // Replace with your CAPSOLVER API Key
const parsedUrl = new URL(PAGE_URL);
const domain = parsedUrl.hostname;
const formattedDomain = `.${domain}`;
const headers = {
"cache-control": "max-age=0",
"sec-ch-ua": '"Not/A)Brand";v="99", "Google Chrome";v="107", "Chromium";v="107"',
"sec-ch-ua-mobile": "?0",
"sec-ch-ua-platform": "Windows",
"upgrade-insecure-requests": "1",
"user-agent": "Mozilla/5.0 (Windows NT 10.0; Win64; x64) AppleWebKit/537.36 (KHTML, like Gecko) Chrome/112.0.0.0 Safari/537.36",
"accept": "*/*",
"sec-fetch-site": "same-origin",
"sec-fetch-mode": "navigate",
"sec-fetch-user": "?1",
"sec-fetch-dest": "document",
"accept-encoding": "gzip, deflate, br",
"accept-language": "en,fr-FR;q=0.9,fr;q=0.8,en-US;q=0.7"
};
async function createTask(payload) {
try {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: CLIENT_KEY,
task: payload
});
return res.data;
} catch (error) {
console.error(error);
}
}
async function getTaskResult(taskId) {
try {
success = false;
while(success == false){
await sleep(1000);
console.log("Getting task result for task ID: " + taskId);
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: CLIENT_KEY,
taskId: taskId
});
if( res.data.status == "ready") {
success = true;
console.log(res.data)
return res.data;
}
}
} catch (error) {
console.error(error);
return null;
}
}
async function solveAwsCaptcha(websiteURL, awsKey, awsIv, awsContext, awsChallengeJS) {
const taskPayload = {
type: "FunCaptchaTaskProxyless",
websiteURL,
awsKey,
awsIv,
awsContext,
awsChallengeJS,
proxy: PROXY
};
const taskData = await createTask(taskPayload);
console.log(taskData)
return await getTaskResult(taskData.taskId);
}
async function solveAwsChallenge(awsChallengeJS) {
const taskPayload = {
type: "AntiAwsWafTask",
websiteURL: PAGE_URL,
awsKey: "",
awsIv: "",
awsContext: "",
awsChallengeJS,
proxy: PROXY
};
const taskData = await createTask(taskPayload);
return await getTaskResult(taskData.taskId);
}
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function main() {
try {
console.log(PROXY)
let cookie = "";
const response = await axios.get('https://norway-meetup.aws.wslab.no/', {
headers: {
headers
},
method: 'GET',
validateStatus: function (status) {
return status >= 200 && status < 500; // Resolve the promise for status codes in this range
}
});
console.log(response.status);
//console.log(response.data);
if (response.status === 202) {
console.log("AWS Challenge Solve required");
const $ = cheerio.load(response.data);
const scriptTags = $('script[src*="token.awswaf.com"]');
const awsChallengeJS = scriptTags.attr('src');
const response = await solveAwsChallenge(awsChallengeJS);
console.log(`Received AWS Cookie: ${response.solution.cookie}`);
cookie = response.solution.cookie;
}
if (response.status === 405) {
console.log("AWS Captcha + Challenge Solve required");
let src = "";
const $ = cheerio.load(response.data);
$('script').each(function() {
src = $(this).attr('src');
if (src && src.includes('token.awswaf.com')) {
console.log(`Found URL: ${src}`);
}
});
const scriptContent = $("script[type='text/javascript']").last().html();
if (scriptContent) {
// Extract the necessary data
const keyMatch = /"key":"(.*?)"/.exec(scriptContent);
const key = keyMatch ? keyMatch[1] : null;
const ivMatch = /"iv":"(.*?)"/.exec(scriptContent);
const iv = ivMatch ? ivMatch[1] : null;
const contextMatch = /"context":"(.*?)"/.exec(scriptContent);
const context = contextMatch ? contextMatch[1] : null;
console.log("Key:", key);
console.log("IV:", iv);
console.log("Context:", context);
console.log("Solving AWS Captcha + Challenge")
const response = await solveAwsCaptcha(PAGE_URL, key, iv, context, src);
console.log(`Received AWS Cookie:`+response.solution.cookie);
cookie = response.solution.cookie;
} else
{
console.log("Couldn't find the script containing the required data.");
}
const solution = {
cookie: cookie
};
// Prepare Axios instance with headers
const response2 = await axios.get({
baseURL: PAGE_URL,
headers: {
'Cookie': `aws-waf-token=${solution.cookie}`
}
});
console.log(response2.data);
}
}catch (error) {
console.error(`Error: ${error}`);
}
}
main();
⚠️ Change these variables
- PROXY: Change to your proxy
- CLIENT_KEY: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve aws captcha