Using Playwright with Ruby: Step-by-Step Guide for 2024
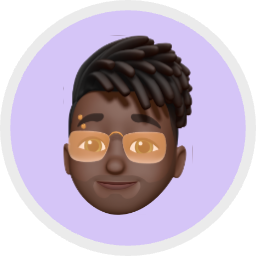
Lucas Mitchell
Automation Engineer
02-Sep-2024
Using Playwright with Ruby: Step-by-Step Guide for 2024
Web scraping has become an essential skill for developers who need to gather data from websites. Playwright, a powerful browser automation tool, is often used for this purpose. In this guide, we will explore how to use Playwright with Ruby to scrape data from a website. We will walk through a practical example using the website Quotes to Scrape.
Prerequisites
Before we begin, make sure you have the following installed on your machine:
- Ruby (Version 2.7 or later)
- Node.js (Playwright requires Node.js to run)
- Playwright Gem (Ruby wrapper for Playwright)
You can install the necessary dependencies by running:
bash
gem install playwright-ruby-client
Setting Up Playwright
After installing the playwright-ruby-client
gem, you need to set up Playwright in your Ruby script. Here’s how you can do it:
ruby
require 'playwright'
Playwright.create(playwright_cli_executable_path: '/path/to/node_modules/.bin/playwright') do |playwright|
browser = playwright.chromium.launch(headless: false)
page = browser.new_page
page.goto('http://quotes.toscrape.com/')
# Example scraping code will go here
browser.close
end
Replace '/path/to/node_modules/.bin/playwright'
with the actual path to the Playwright CLI on your system.
Scraping Quotes from the Website
Now, let's write the code to scrape quotes from the website. We will extract the text of each quote and the corresponding author.
ruby
require 'playwright'
Playwright.create(playwright_cli_executable_path: '/path/to/node_modules/.bin/playwright') do |playwright|
browser = playwright.chromium.launch(headless: false)
page = browser.new_page
page.goto('http://quotes.toscrape.com/')
quotes = page.query_selector_all('.quote')
quotes.each do |quote|
quote_text = quote.query_selector('.text').text_content.strip
author = quote.query_selector('.author').text_content.strip
puts "#{quote_text} - #{author}"
end
browser.close
end
Explanation
- Navigating to the Website: The script navigates to the
http://quotes.toscrape.com/
URL using thepage.goto
method. - Selecting Quotes: We use
page.query_selector_all('.quote')
to select all elements that have the classquote
. - Extracting Text and Author: For each quote, we extract the text content and the author using the respective selectors.
- Output: Finally, we print each quote followed by its author to the console.
Running the Script
You can run this Ruby script from your terminal:
bash
ruby playwright_scraper.rb
Make sure to replace playwright_scraper.rb
with the filename of your script.
Handling CAPTCHA Challenges with Playwright and Ruby
CAPTCHA challenges are a common obstacle when scraping websites, designed to differentiate between human users and bots. For developers using Playwright with Ruby, overcoming these challenges is essential to successfully automate data extraction. In this guide, we'll explore how to integrate CAPTCHA solving services using CapSolver with Playwright. Depending on the type of CAPTCHA implemented by the website, you can either configure CapSolver via an extension (the simplest method) or through their API for more advanced use cases.
For detailed instructions on setting up the extension, visit CapSolver Extension Documentation. For API integration, refer to the CapSolver API Documentation.
Conclusion
This guide has shown you how to set up Playwright with Ruby and scrape data from a website. The example used here is simple but can be expanded for more complex tasks. Playwright’s ability to automate browser tasks makes it a powerful tool for web scraping and testing.
Happy scraping!
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
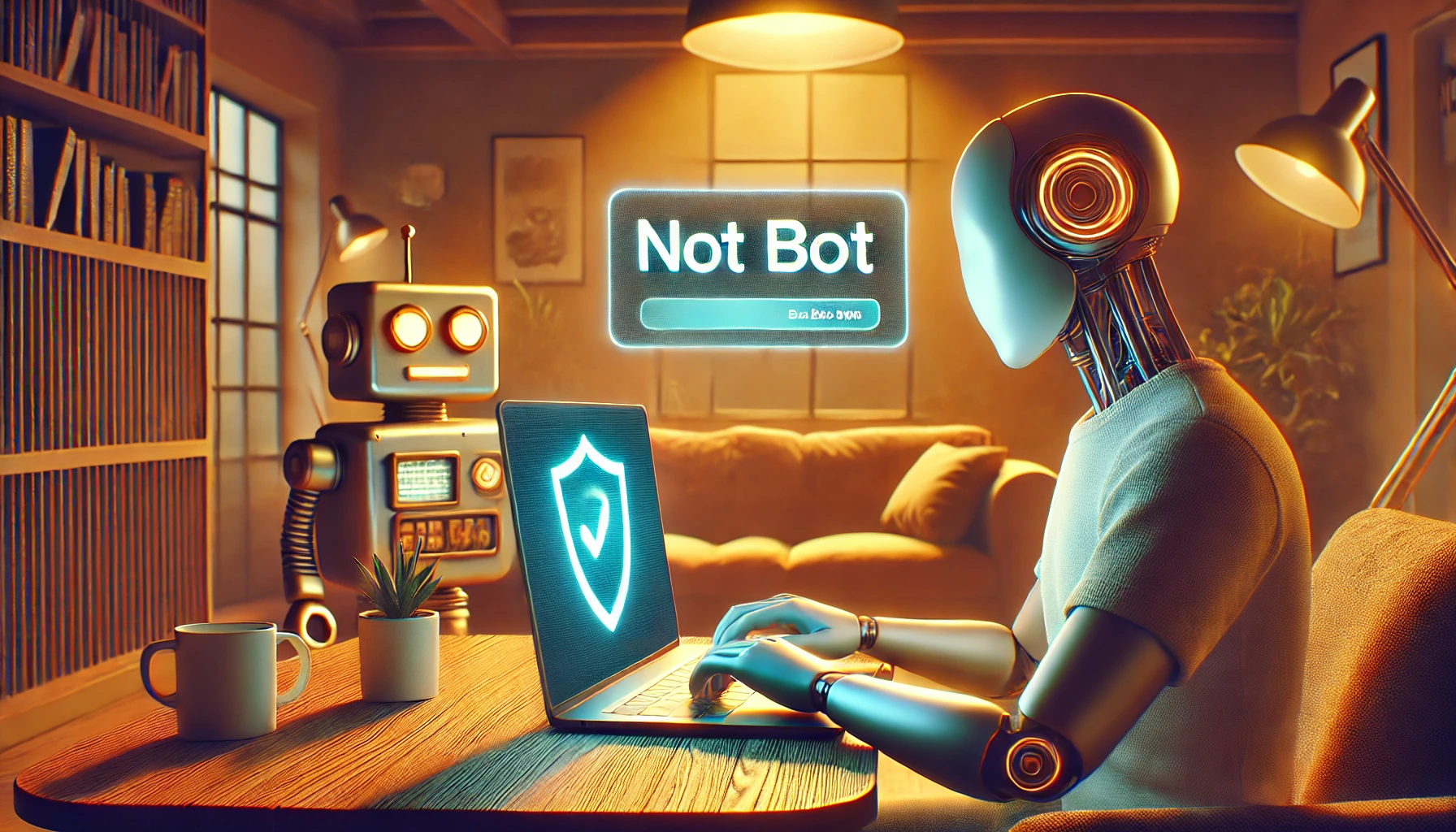
Why do I keep getting asked to verify I'm not a robot?
Learn why Google prompts you to verify you're not a robot and explore solutions like using CapSolver’s API to solve CAPTCHA challenges efficiently.
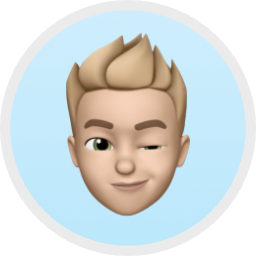
Ethan Collins
27-Feb-2025
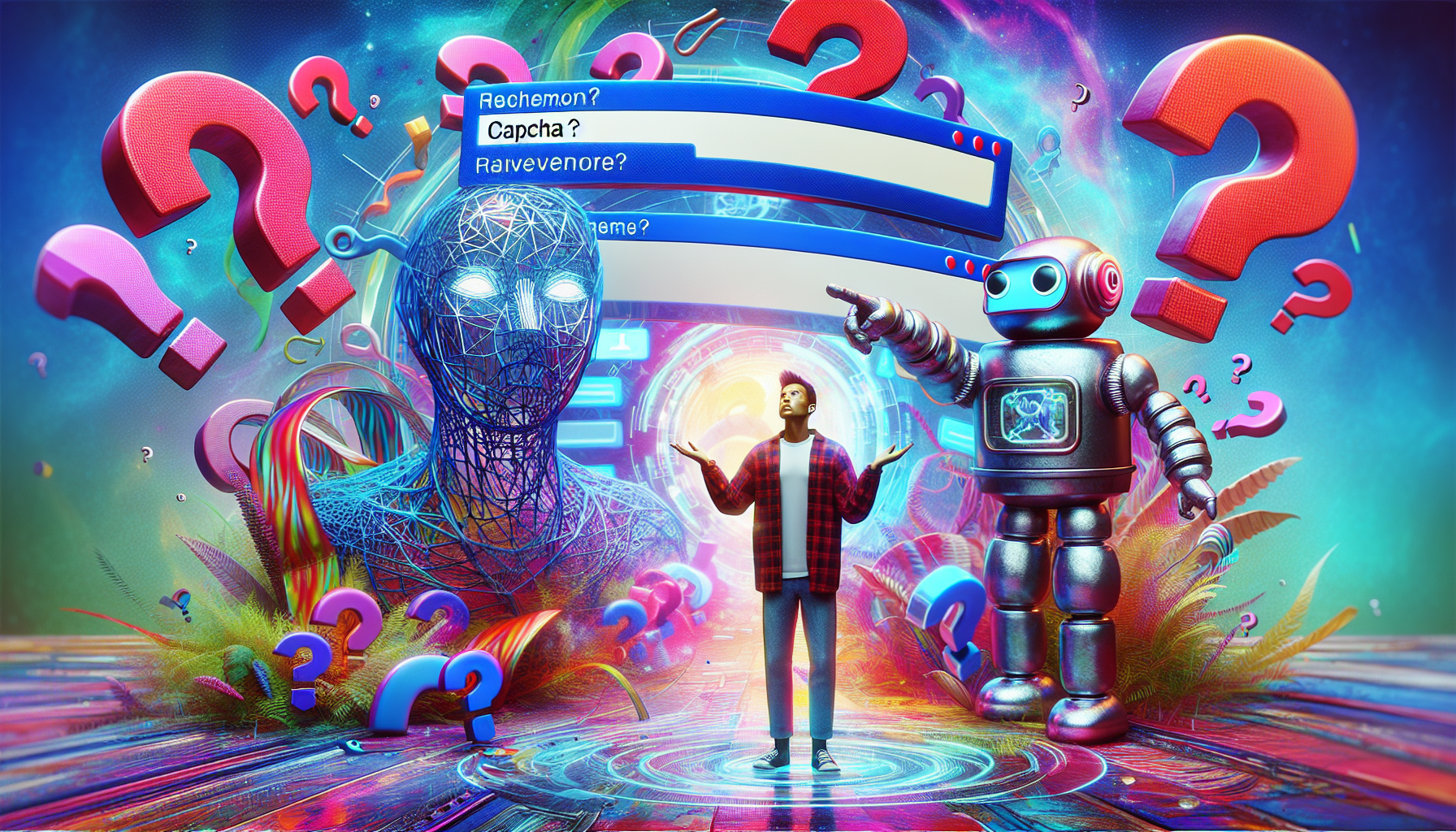
Why Do Websites Think I'm a Bot? And How to Solve Them
Understand why websites flag you as a bot and how to avoid detection. Key triggers include CAPTCHA challenges, suspicious IPs, and unusual browser behavior.
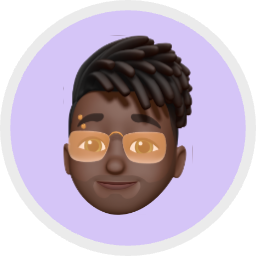
Lucas Mitchell
20-Feb-2025
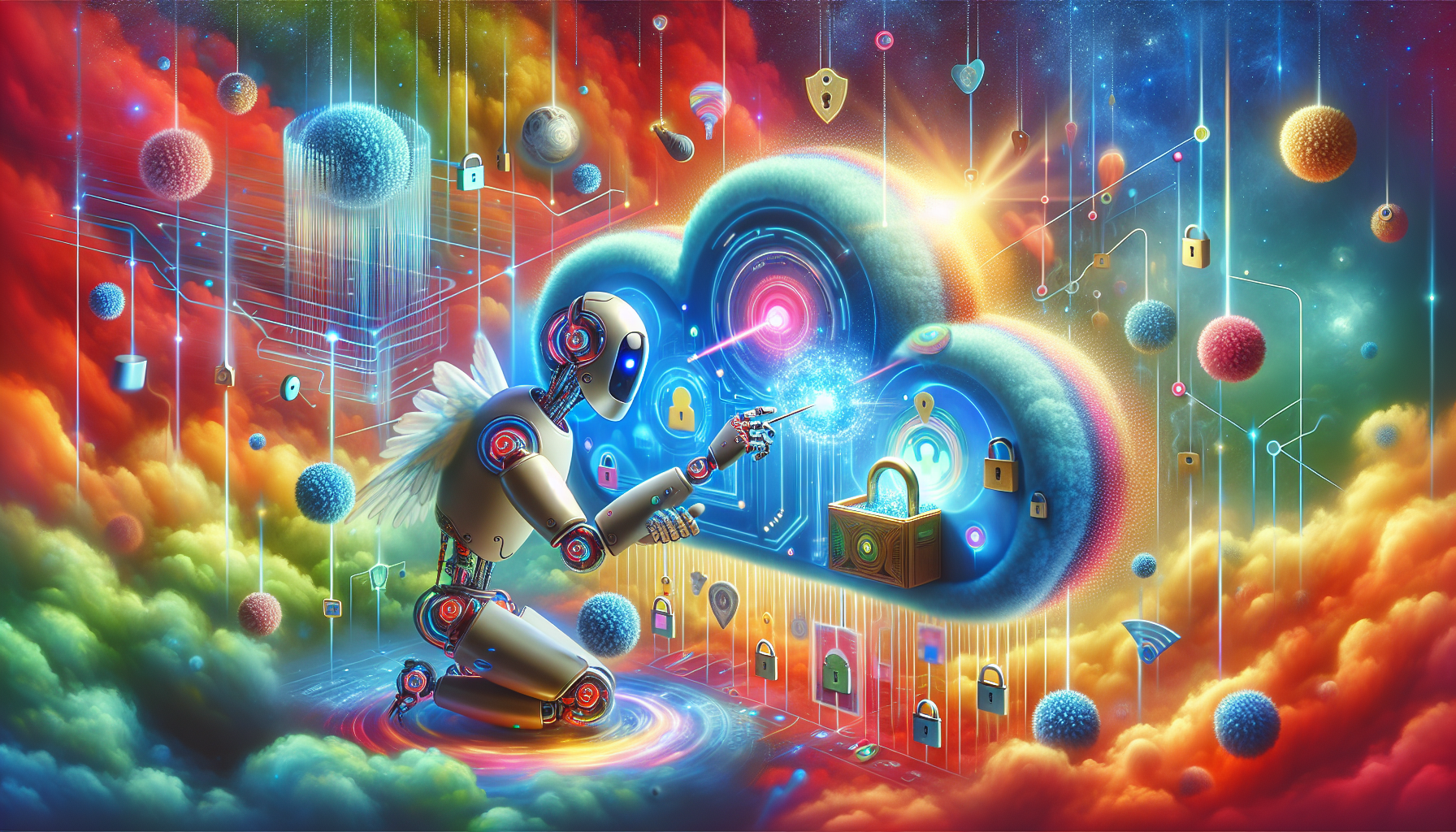
How to Extract Data from a Cloudflare-Protected Website
In this guide, we'll explore ethical and effective techniques to extract data from Cloudflare-protected websites.
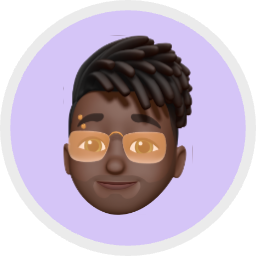
Lucas Mitchell
20-Feb-2025
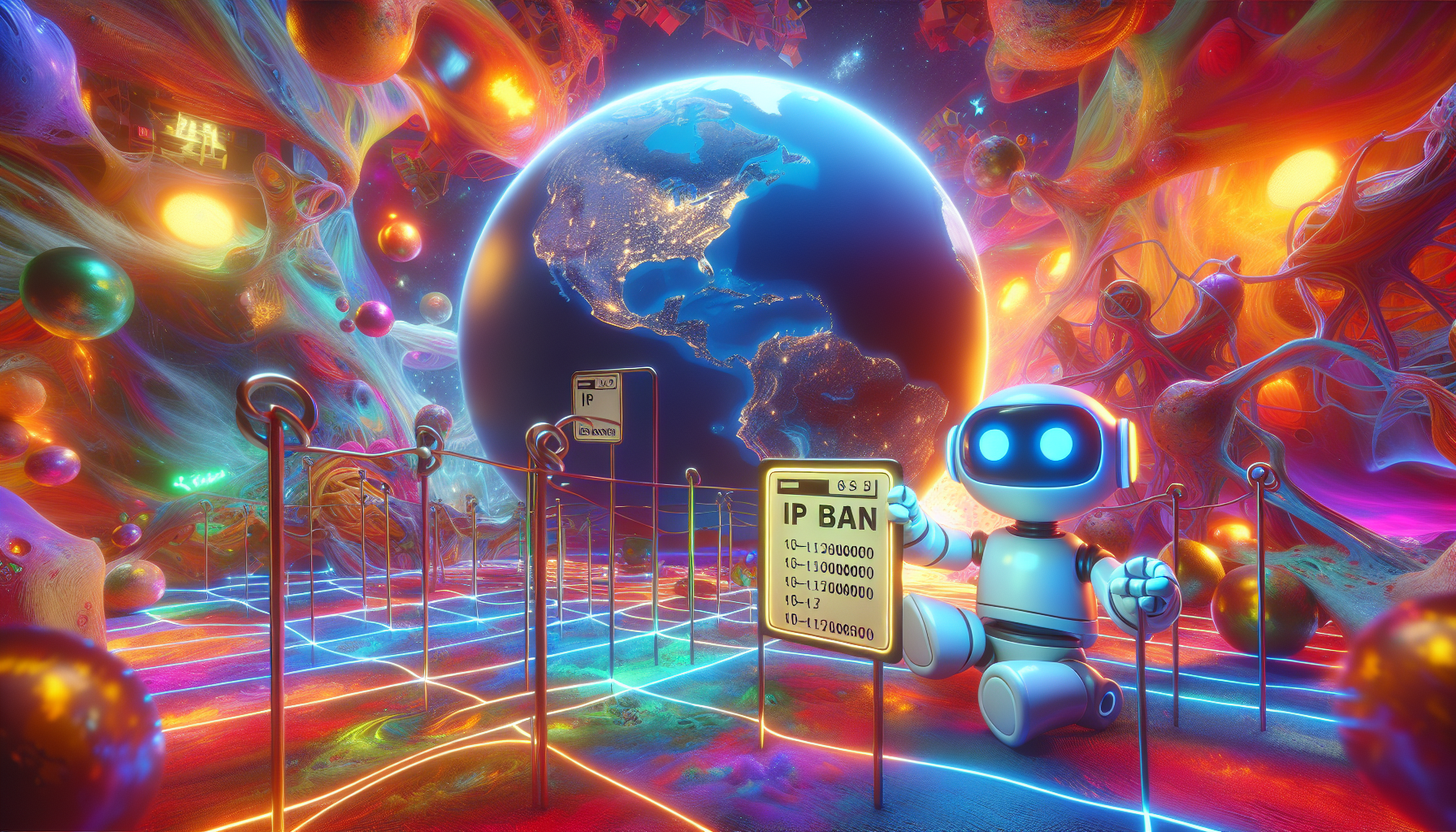
How to Avoid IP Bans when Using Captcha Solver in 2025
Learn effective strategies to prevent IP bans when using captcha solvers in 2025. Explore the best practices, tools, and techniques to safely and efficiently solve CAPTCHA challenges.
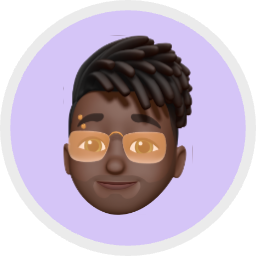
Lucas Mitchell
18-Feb-2025
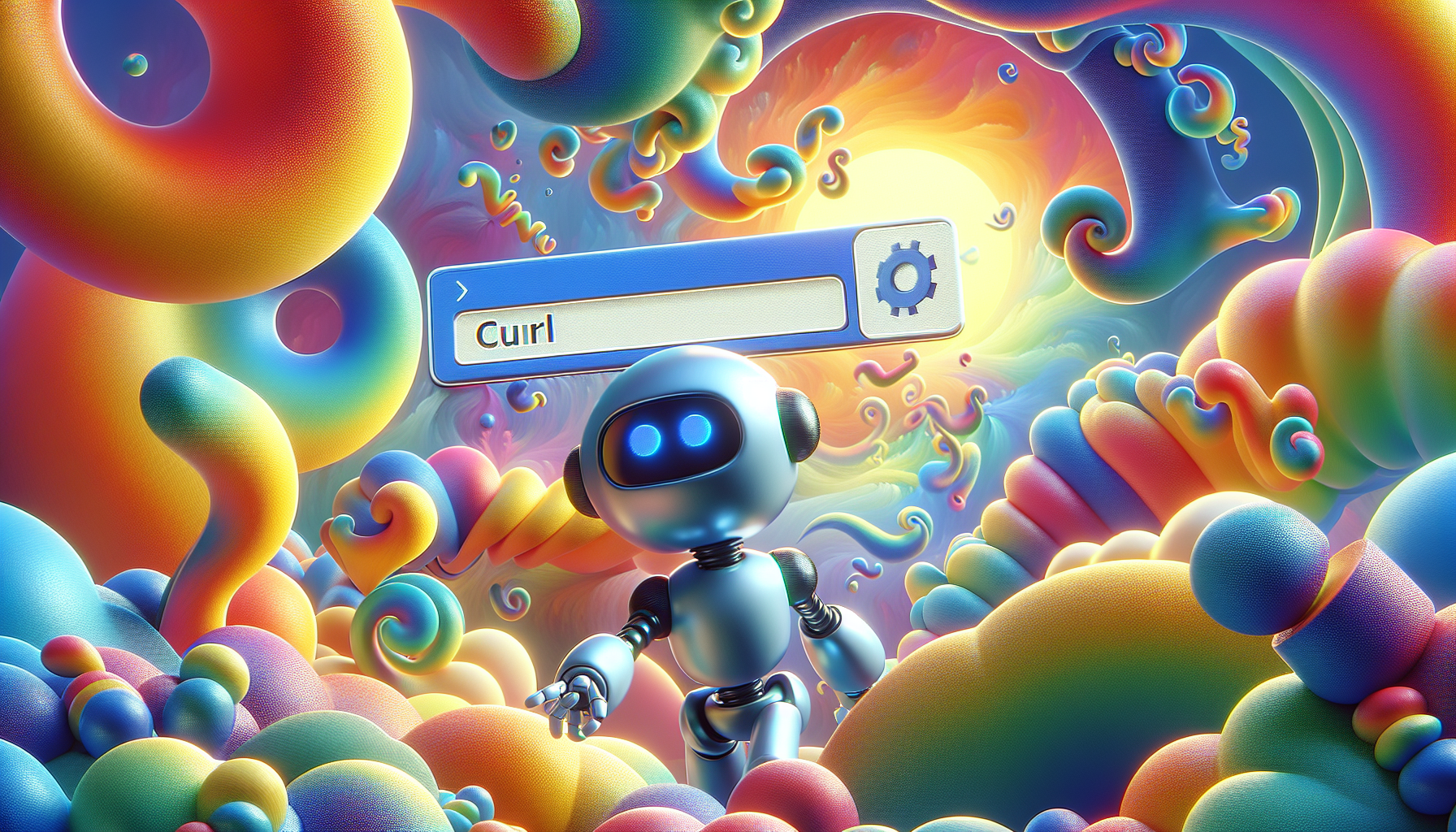
Solving CAPTCHA with cURL: A Step-by-Step Guide
Learn how to solve CAPTCHA using cURL with simple steps and automation techniques.
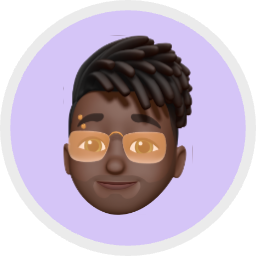
Lucas Mitchell
18-Feb-2025
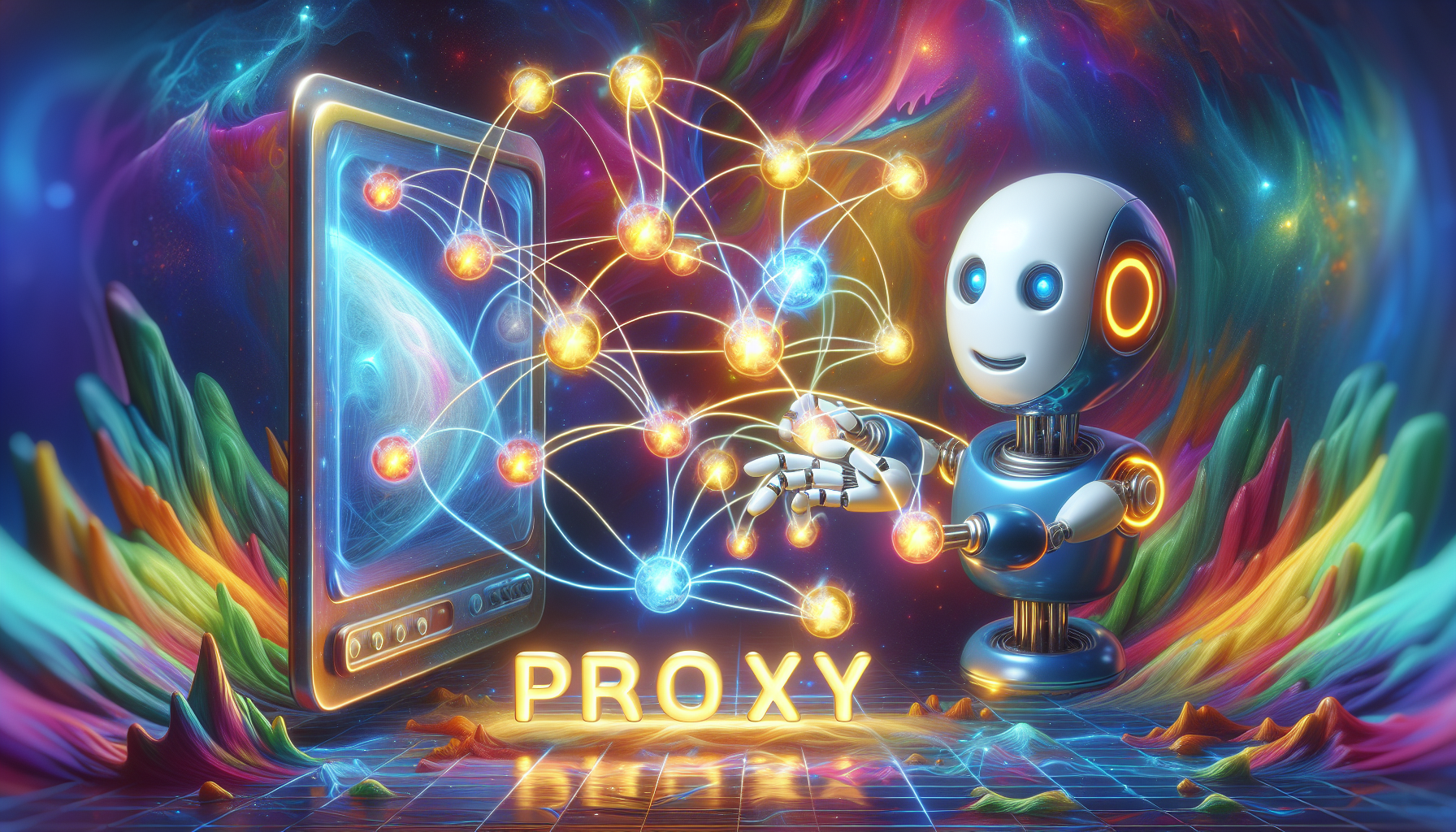
How to Set Up Proxies for CAPTCHA Solving
Discover how to set up proxies for CAPTCHA solving using CapSolver. This comprehensive guide explains proxy configurations, task types, and integration strategies for efficient web scraping and automation while bypassing CAPTCHA challenges.
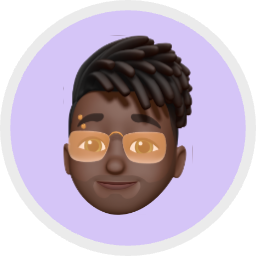
Lucas Mitchell
17-Feb-2025