How to Handle Multiple reCAPTCHA Challenges Concurrently
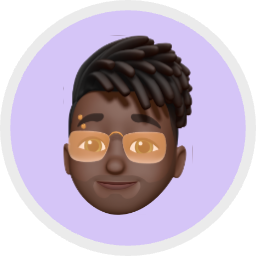
Lucas Mitchell
Automation Engineer
10-Sep-2024
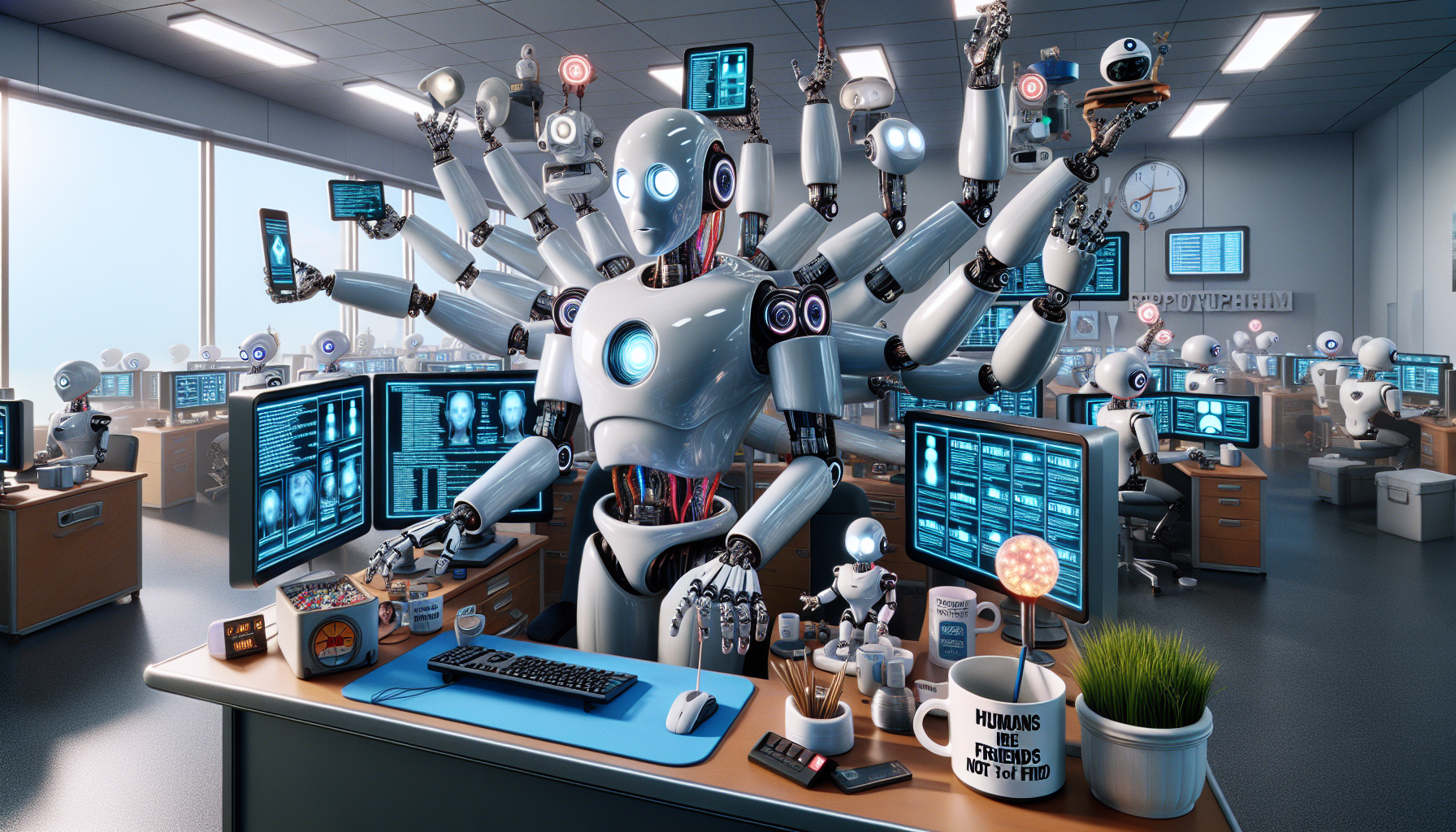
When performing web scraping, one of the common obstacles you might encounter is CAPTCHA challenges. Websites often use CAPTCHAs to prevent bots from accessing their content. CAPTCHA (Completely Automated Public Turing test to tell Computers and Humans Apart) is a widely-used technique to ensure that the user is human, not an automated bot.
In this guide, we’ll discuss different types of reCAPTCHA challenges, how to identify them using tools, and finally, how to solve multiple reCAPTCHA challenges concurrently using Python and threading.
What is Web Scraping?
WebScraping is the process of extracting data from websites. It is often used for tasks such as collecting prices from e-commerce websites, gathering news articles, or aggregating information from various web sources. Scraping involves making HTTP requests to a website and parsing the data from the response. However, many websites use CAPTCHAs to prevent automated access.
Types of reCAPTCHA Challenges
reCAPTCHA v2
reCAPTCHA V2, this type of CAPTCHA is the most widely used and appears as a "checkbox" challenge labeled "I'm not a robot." It might ask the user to select certain images to verify they are human.
reCAPTCHA v3
reCAPTCHA V3 works in the background, scoring user interactions to detect bot-like behavior. This system is designed to avoid disrupting the user experience by providing a score to the website, which can be used to block bots or require additional verification steps.
Invisible reCAPTCHA
Invisible reCAPTCHA is a more user-friendly version of reCAPTCHA v2, where the challenge only appears if the system suspects bot-like behavior.
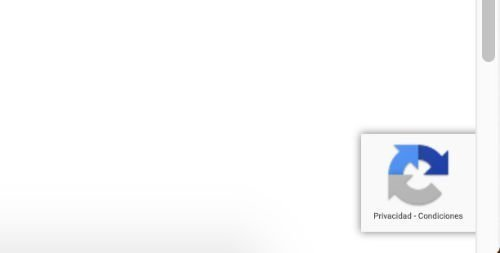
Identifying CAPTCHA Types
Installation
To identify the type of CAPTCHA being used on a website, you can use the following tools:
- Chrome users: Install the Captcha Solver Auto Solve extension.
- Firefox users: Install the Captcha Solver Auto Solver FireFox Version.
Capsolver Setup
Capsolver is a service that allows you to solve CAPTCHA challenges programmatically. To detect CAPTCHA parameters:
- Go to Capsolver.
- Press the "F12" key on your keyboard to open the developer tools in your browser.
- Navigate to the tab labeled Capsolver Captcha Detector.

CAPTCHA Detection
Once you've set up Capsolver, follow these steps to detect CAPTCHA parameters:
- Without closing the Capsolver panel, visit the website where you want to trigger the CAPTCHA.
- Trigger the CAPTCHA manually.
- Make sure not to close the Capsolver panel before triggering the CAPTCHA.
reCAPTCHA Detection
The Capsolver Captcha Detector can return detailed information about reCAPTCHAs:

Key Parameters for reCAPTCHA:
Website URL
Site Key
pageAction
isInvisible
isEnterprise
isSRequired
isReCaptchaV3
API Domain
Once these parameters are detected, Capsolver will return a JSON object with all the necessary details to submit the CAPTCHA to their service.
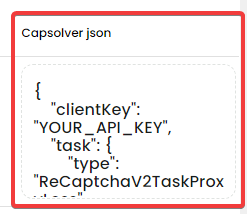
Solving Multiple reCAPTCHA Challenges Concurrently
When working on web scraping projects, solving CAPTCHAs can become time-consuming, especially when you need to solve multiple CAPTCHAs simultaneously. Here's how you can automate solving multiple reCAPTCHA challenges concurrently using Python.
Example: Solving Multiple reCAPTCHA v2 Challenges
python
import capsolver
import threading
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL = "PAGE_URL"
PAGE_KEY = "PAGE_SITE_KEY"
def solve_recaptcha_v2():
solution = capsolver.solve({
"type": "ReCaptchaV2TaskProxyless",
"websiteURL": PAGE_URL,
"websiteKey": PAGE_KEY,
})
return solution
def solve_recaptcha_task(result_list, index):
result = solve_recaptcha_v2()
result_list[index] = result
def solve_multiple_recaptchas(num_tasks):
threads = []
results = [None] * num_tasks
for i in range(num_tasks):
thread = threading.Thread(target=solve_recaptcha_task, args=(results, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return results
def main():
num_tasks = 10 # Number of simultaneous tasks
print(f"Solving {num_tasks} reCaptcha v2 tasks simultaneously")
solutions = solve_multiple_recaptchas(num_tasks)
for i, solution in enumerate(solutions):
print(f"Solution {i+1}: {solution}")
if __name__ == "__main__":
main()
Solving Multiple reCAPTCHA v3 Challenges
The process for solving reCAPTCHA v3 is quite similar to v2, but you'll need to adjust the CAPTCHA type accordingly.
python
```python
import capsolver
import threading
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL = "PAGE_URL"
PAGE_KEY = "PAGE_SITE_KEY"
def solve_recaptcha_v3():
solution = capsolver.solve({
"type": "ReCaptchaV3TaskProxyless",
"websiteURL": PAGE_URL,
"websiteKey": PAGE_KEY,
})
return solution
def solve_recaptcha_task(result_list, index):
result = solve_recaptcha_v3()
result_list[index] = result
def solve_multiple_recaptchas(num_tasks):
threads = []
results = [None] * num_tasks
for i in range(num_tasks):
thread = threading.Thread(target=solve_recaptcha_task, args=(results, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return results
def main():
num_tasks = 10 # Number of simultaneous tasks
print(f"Solving {num_tasks} reCaptcha v3 tasks simultaneously")
solutions = solve_multiple_recaptchas(num_tasks)
for i, solution in enumerate(solutions):
print(f"Solution {i+1}: {solution}")
if __name__ == "__main__":
main()
Solving Multiple reCAPTCHA v3 Challenges
The process for solving reCAPTCHA v3 is quite similar to v2, but you'll need to adjust the CAPTCHA type accordingly.
python
```python
import capsolver
import threading
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL = "PAGE_URL"
PAGE_KEY = "PAGE_SITE_KEY"
def solve_recaptcha_v3():
solution = capsolver.solve({
"type": "ReCaptchaV3TaskProxyless",
"websiteURL": PAGE_URL,
"websiteKey": PAGE_KEY,
})
return solution
def solve_recaptcha_task(result_list, index):
result = solve_recaptcha_v3()
result_list[index] = result
def solve_multiple_recaptchas(num_tasks):
threads = []
results = [None] * num_tasks
for i in range(num_tasks):
thread = threading.Thread(target=solve_recaptcha_task, args=(results, i))
threads.append(thread)
thread.start()
for thread in threads:
thread.join()
return results
def main():
num_tasks = 10 # Number of simultaneous tasks
print(f"Solving {num_tasks} reCaptcha v3 tasks simultaneously")
solutions = solve_multiple_recaptchas(num_tasks)
for i, solution in enumerate(solutions):
print(f"Solution {i+1}: {solution}")
if __name__ == "__main__":
main()
Solving reCAPTCHA v3 Challenges and reCAPTCHA v2 Challenges
python
import capsolver
# Consider using environment variables for sensitive information
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL_V2 = "PAGE_URL"
PAGE_KEY_V2 = "PAGE_SITE_KEY"
PAGE_URL_V3 = "PAGE_URL"
PAGE_KEY_V3 = "PAGE_SITE_KEY"
def solve_recaptcha_v2(url, key):
solution = capsolver.solve({
"type": "ReCaptchaV2TaskProxyless",
"websiteURL": url,
"websiteKey": key,
})
return solution
def solve_recaptcha_v3(url, key):
solution = capsolver.solve({
"type": "ReCaptchaV3TaskProxyless",
"websiteURL": url,
"websiteKey": key,
"minScore": 0.5 # Adjust the minimum score if needed
})
return solution
def main():
print("Solving reCaptcha v2")
solution_v2 = solve_recaptcha_v2(PAGE_URL_V2, PAGE_KEY_V2)
print("Solution (v2): ", solution_v2)
print("Solving reCaptcha v3")
solution_v3 = solve_recaptcha_v3(PAGE_URL_V3, PAGE_KEY_V3)
print("Solution (v3): ", solution_v3)
if __name__ == "__main__":
main()
Bonus Code
Claim Your Bonus Code for top captcha solutions; CapSolver: scrape. After redeeming it, you will get an extra 5% bonus after each recharge, Unlimited
For more information, read this blog
Conclusion
Handling multiple CAPTCHA challenges is an important skill for anyone working in web scraping, especially as websites increase their security measures. With tools like Capsolver and the power of Python's threading, you can efficiently automate solving CAPTCHA challenges, ensuring smoother scraping processes for your projects.
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
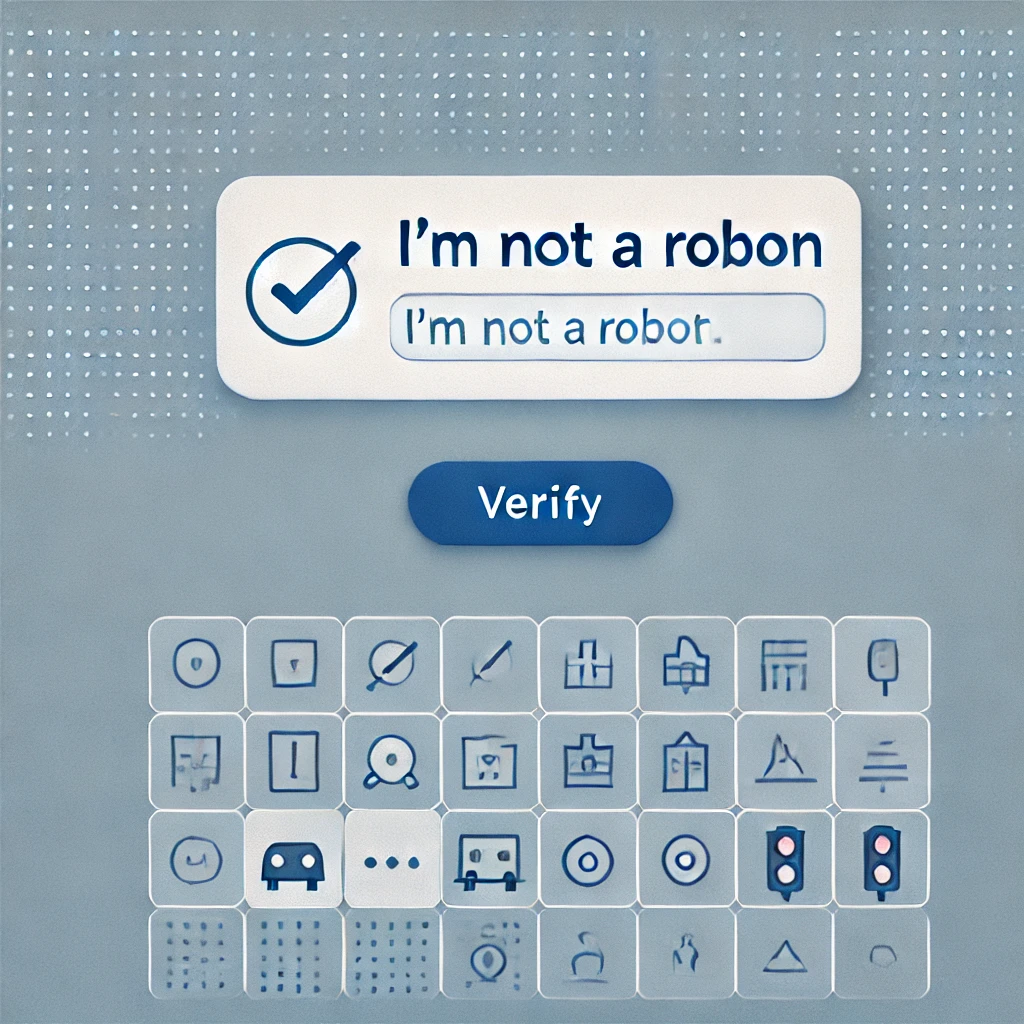
Top 5 Captcha Solvers for reCAPTCHA Recognition in 2025
Explore 2025's top 5 CAPTCHA solvers, including AI-driven CapSolver for fast reCAPTCHA recognition. Compare speed, pricing, and accuracy here
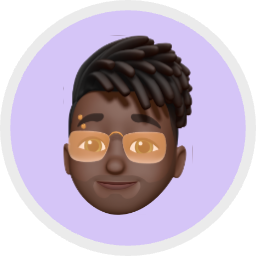
Lucas Mitchell
23-Jan-2025
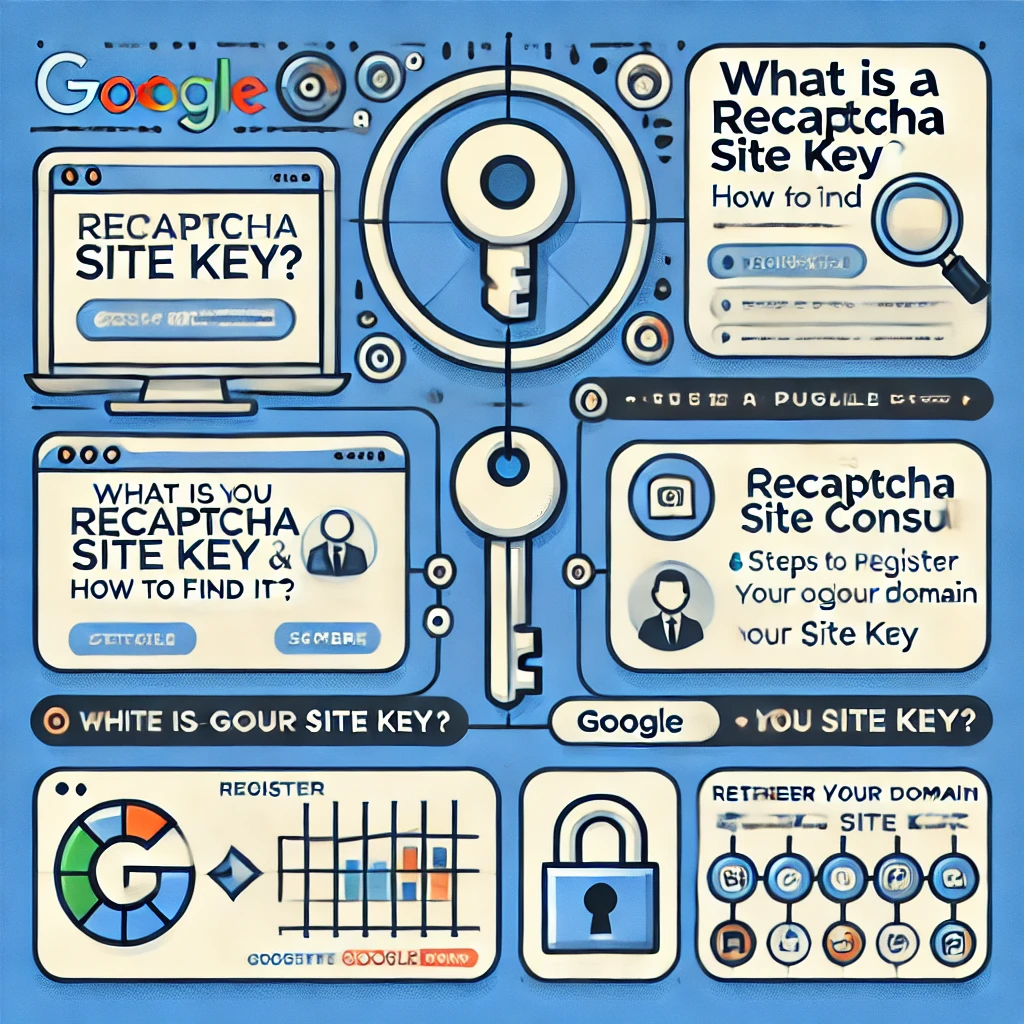
What is a reCAPTCHA Site Key and How to Find It?
Learn how to find a reCAPTCHA Site Key manually or with tools like Capsolver. Fix common issues and automate CAPTCHA solving for developers and web scraping.
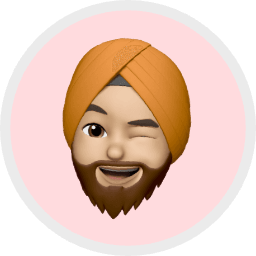
Rajinder Singh
23-Jan-2025
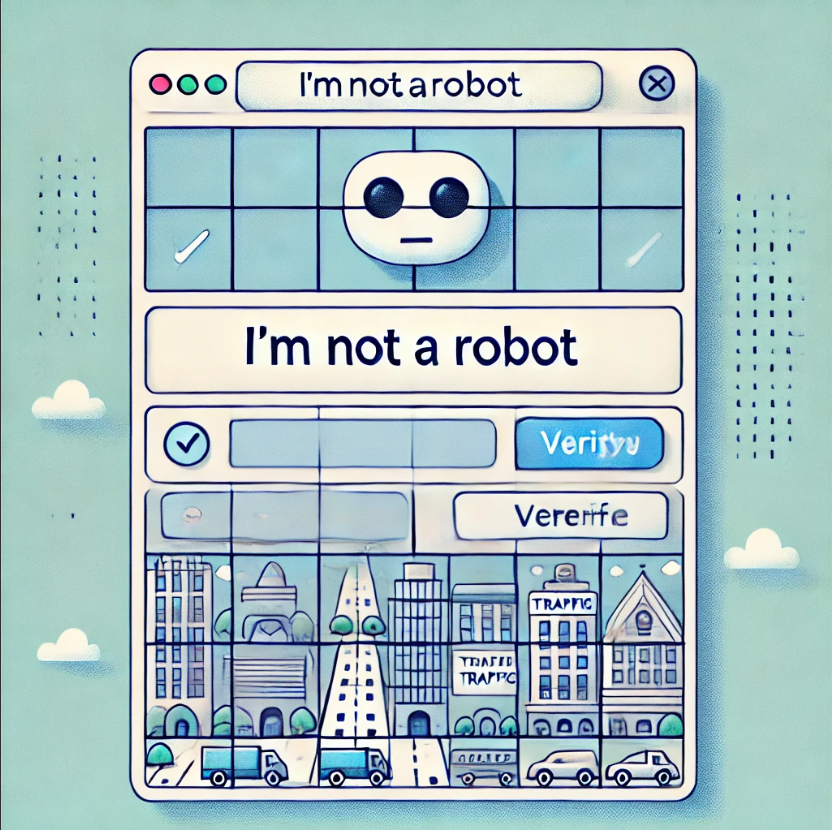
What Is reCAPTCHA Recognition? A Beginner's Guide
Struggling with reCAPTCHA image grids? Discover how Capsolver's AI-powered recognition solves 'Select all ' challenges instantly. Learn API integration, browser extensions, and pro tips to automate CAPTCHA solving with 95%+ accuracy
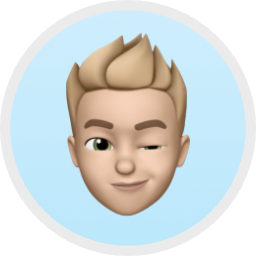
Ethan Collins
23-Jan-2025
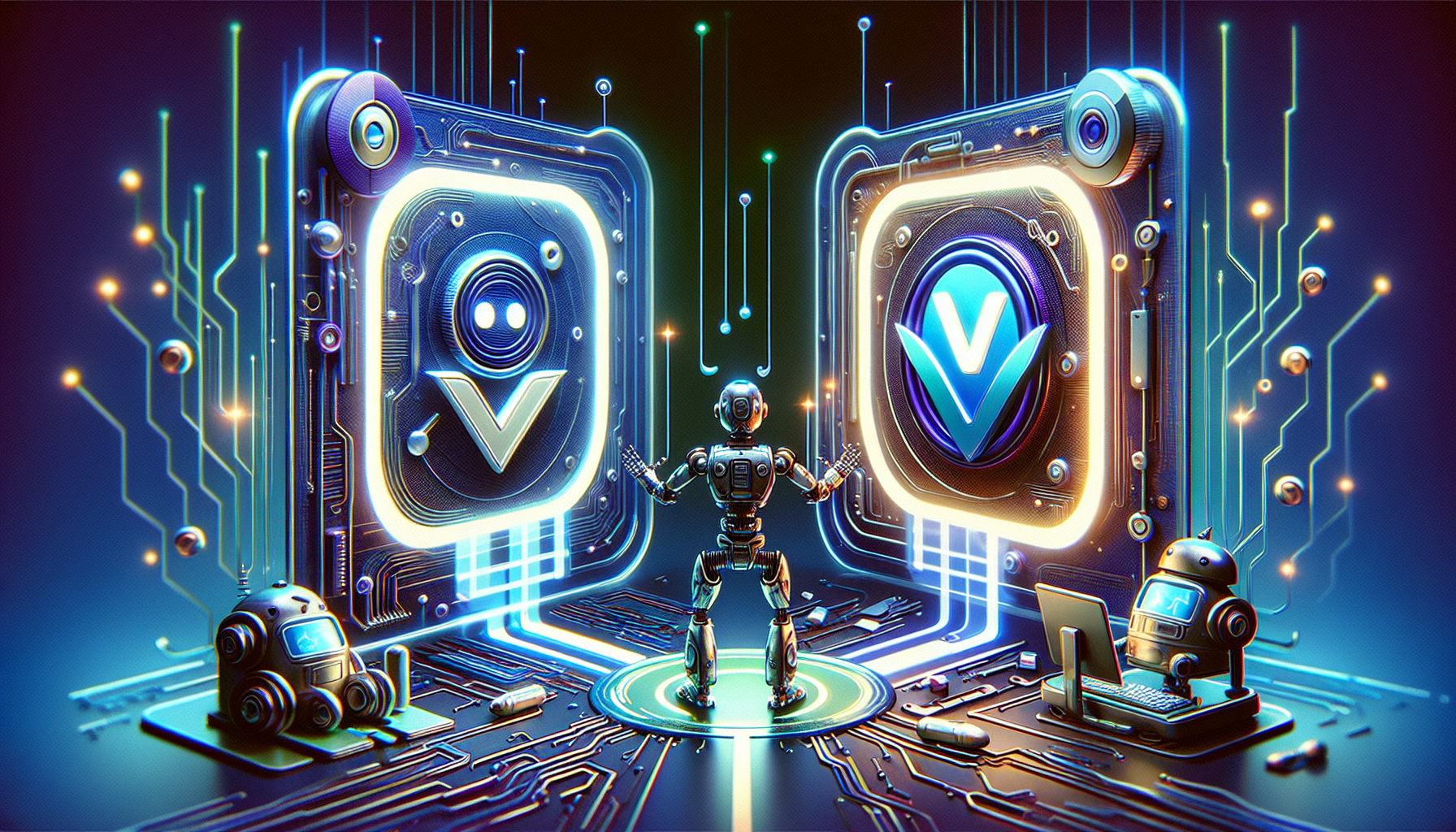
What is the best reCAPTCHA v2 and v3 Solver while web scraping in 2025
In 2025, with the heightened sophistication of anti-bot systems, finding reliable reCAPTCHA solvers has become critical for successful data extraction.
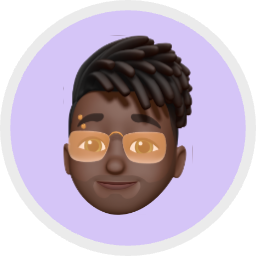
Lucas Mitchell
17-Jan-2025
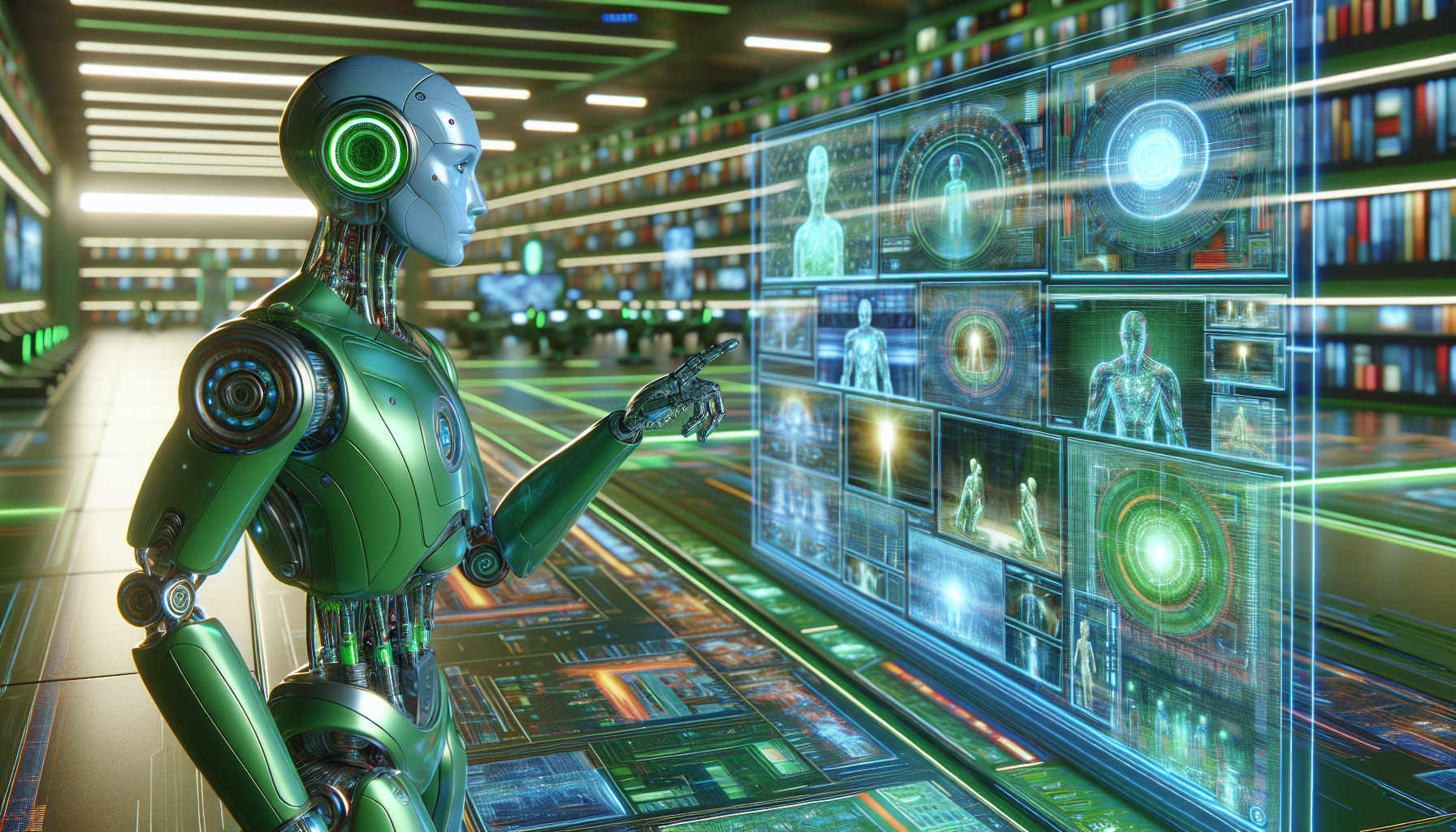
Solving reCAPTCHA with AI Recognition in 2025
Explore how AI is transforming reCAPTCHA-solving, CapSolver's solutions, and the evolving landscape of CAPTCHA security in 2025.
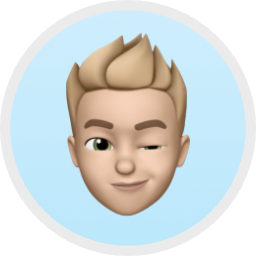
Ethan Collins
11-Nov-2024
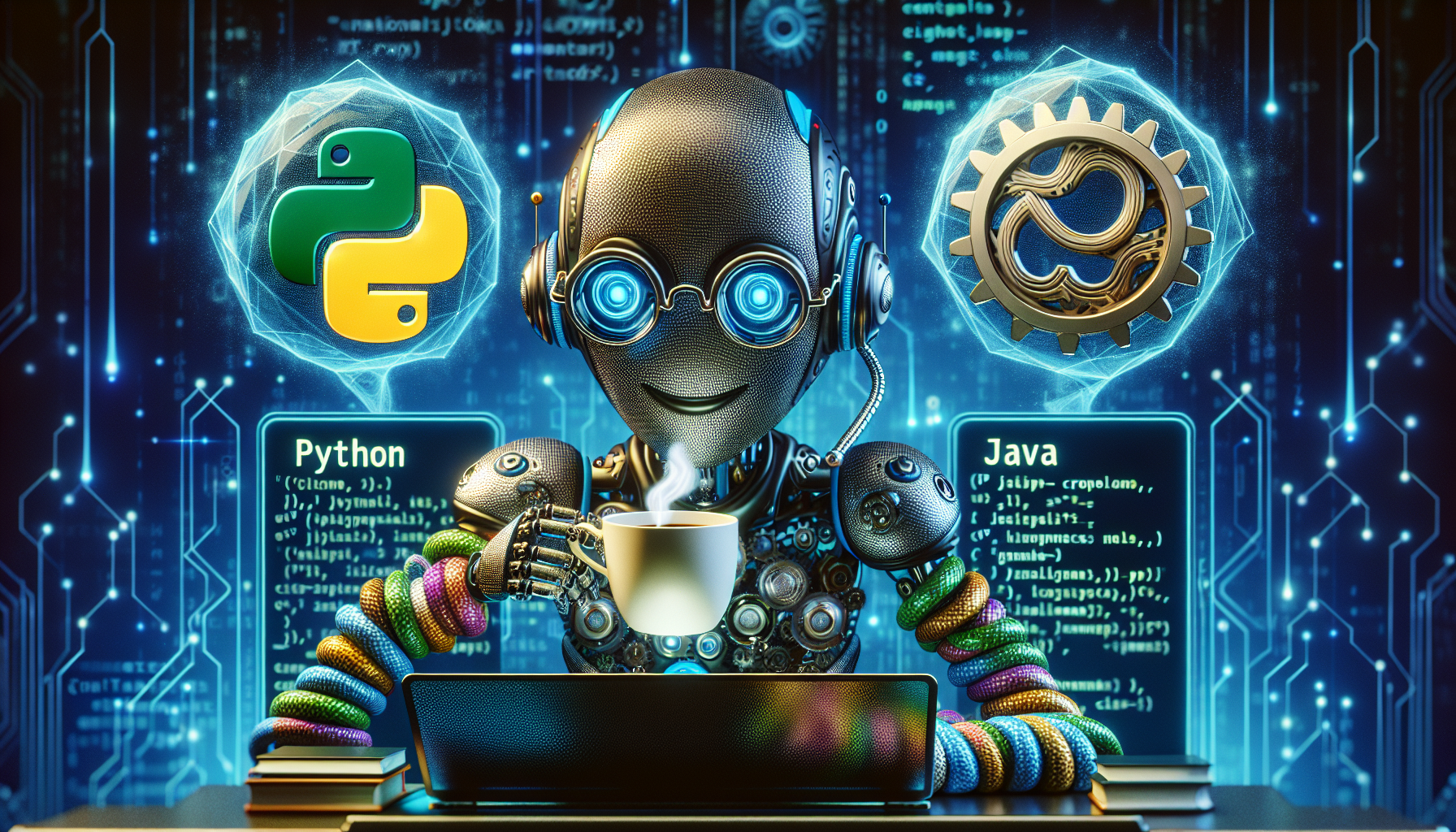
Solving reCAPTCHA Using Python, Java, and C++
What to know how to successfully solve reCAPTCHA using three powerful programming languages: Python, Java, and C++ in one blog? Get in!
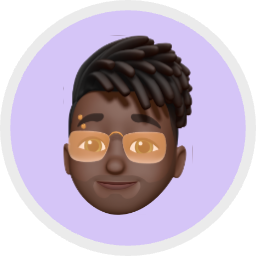
Lucas Mitchell
25-Oct-2024