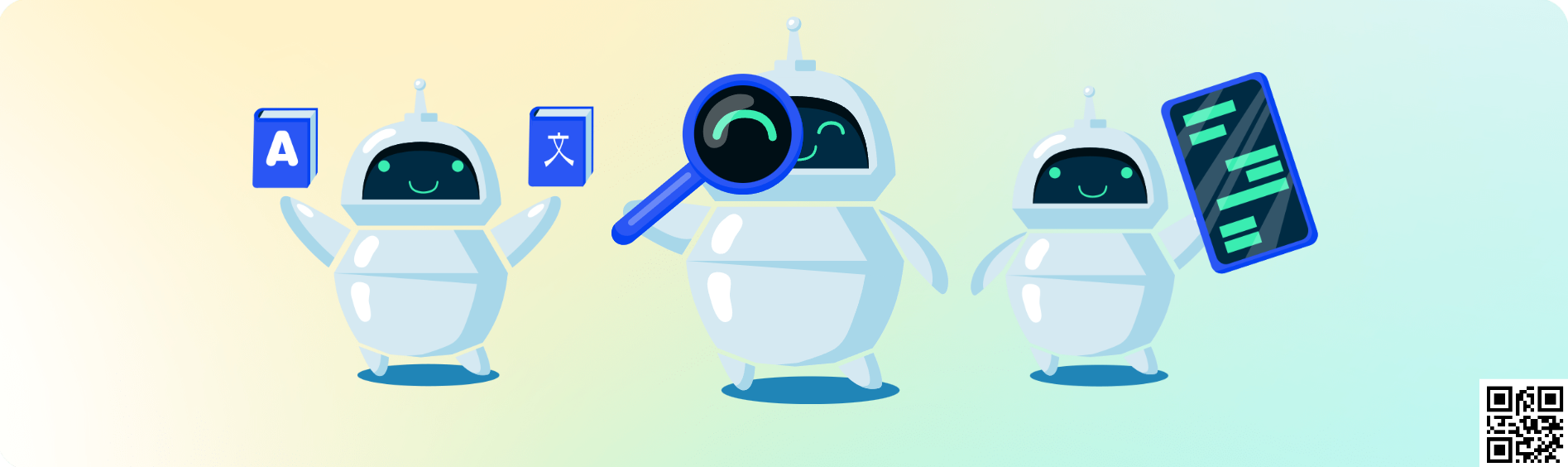
⚙️ Prerequisites
- A working proxy (Optional, read both examples, as one require proxy and other don't require)
- Python installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
pip install capsolver
👨💻 Python Code for solve reCaptcha v2 with your proxy
Here's a Python sample script to accomplish the task:
import capsolver
# Consider using environment variables for sensitive information
PROXY = "http://username:password@host:port"
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL = "PAGE_URL"
PAGE_KEY = "PAGE_SITE_KEY"
def solve_recaptcha_v2(url,key):
solution = capsolver.solve({
"type": "ReCaptchaV2Task",
"websiteURL": url,
"websiteKey":key,
"proxy": PROXY
})
return solution
def main():
print("Solving reCaptcha v2")
solution = solve_recaptcha_v2(PAGE_URL, PAGE_KEY)
print("Solution: ", solution)
if __name__ == "__main__":
main()
👨💻 Python Code for solve reCaptcha v2 without proxy
Here's a Python sample script to accomplish the task:
import capsolver
# Consider using environment variables for sensitive information
capsolver.api_key = "Your Capsolver API Key"
PAGE_URL = "PAGE_URL"
PAGE_KEY = "PAGE_SITE_KEY"
def solve_recaptcha_v2(url,key):
solution = capsolver.solve({
"type": "ReCaptchaV2TaskProxyless",
"websiteURL": url,
"websiteKey":key,
})
return solution
def main():
print("Solving reCaptcha v2")
solution = solve_recaptcha_v2(PAGE_URL, PAGE_KEY)
print("Solution: ", solution)
if __name__ == "__main__":
main()
⚠️ Change these variables
- PROXY: Update with your proxy details. The format should be http://username:password@ip:port. ONLY REQUIRED with ReCaptchaV2Task
- capsolver.api_key: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve reCaptcha v2.
- PAGE_KEY: Replace with the SITEKEY of the website for which you wish to solve reCaptcha v2.