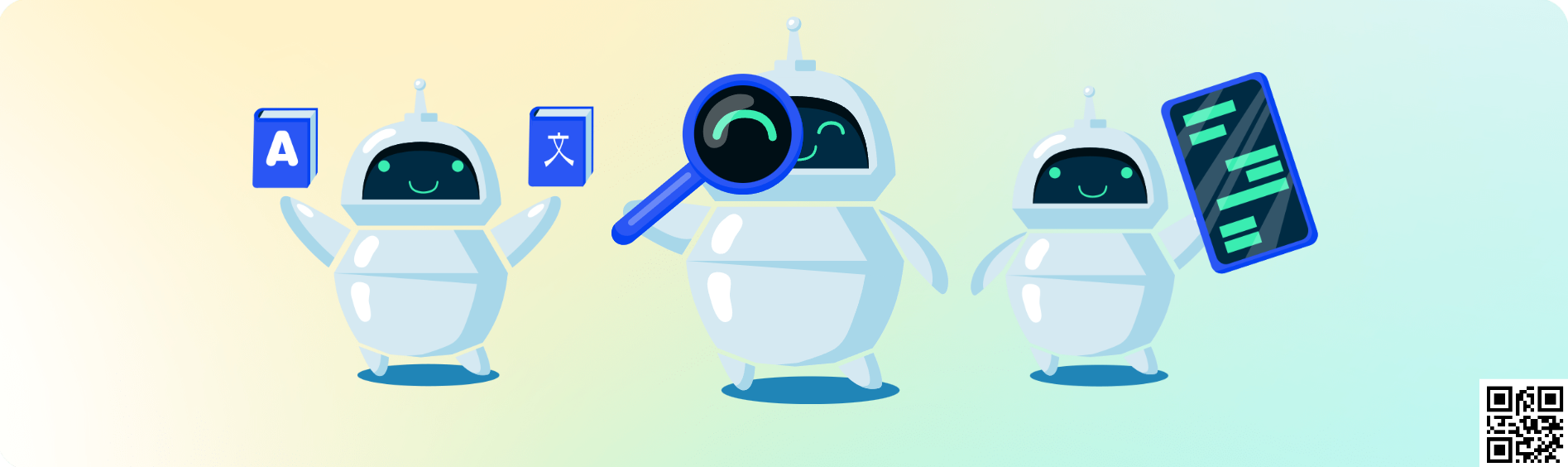
⚙️ Prerequisites
- A working proxy
- Python installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
pip install capsolver
👨💻 Python Code for solve hCaptcha Enterprise with your own proxy
Here's a Python sample script to accomplish the task:
import capsolver
# Change these values
PROXY = "http://username:password@host:port"
capsolver.api_key = "YourPayPerUsage Key"
PAGE_URL = "Site url"
PAGE_KEY = "Your site key"
def solve_hcaptcha_enterprise(url,key):
solution = capsolver.solve({
"type": "HCaptchaTask",
"websiteURL": url,
"websiteKey":key,
"proxy": PROXY
})
return solution
def main():
print("Solving hCaptcha Enterprise...")
solution = solve_hcaptcha_enterprise(PAGE_URL, PAGE_KEY)
print("Solution: ", solution)
if __name__ == "__main__":
main()
⚠️ Change these variables
- PROXY: Update with your proxy details. The format should be http://username:password@ip:port.
- capsolver.api_key: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve hcaptcha enterprise captcha
- PAGE_KEY: Replace with the site key of the website for which you wish to solve hcaptcha enterprise captcha