
CapSolver Blogger
How to use CapSolver
-
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
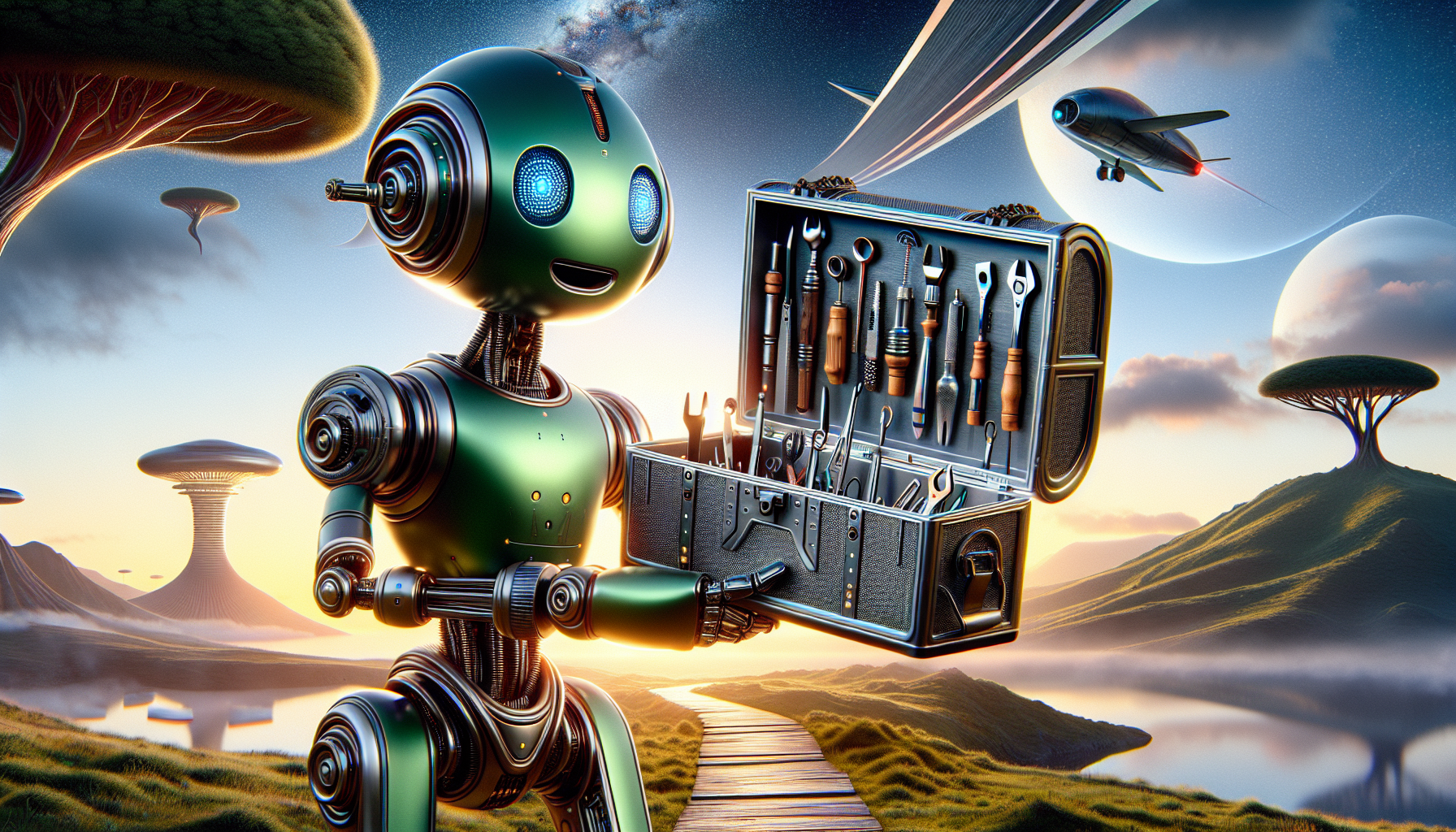
How to Use Libcurl for Web Scraping
Learn how to solve ReCaptcha V3 using CapSolver with libcurl in C++. This comprehensive guide covers HTTP requests, proxies, cookies, and more with practical C++ examples using libcurl.
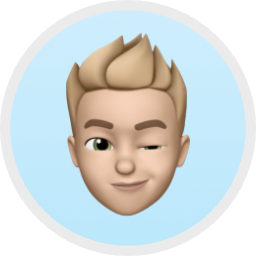
Ethan Collins
22-Oct-2024
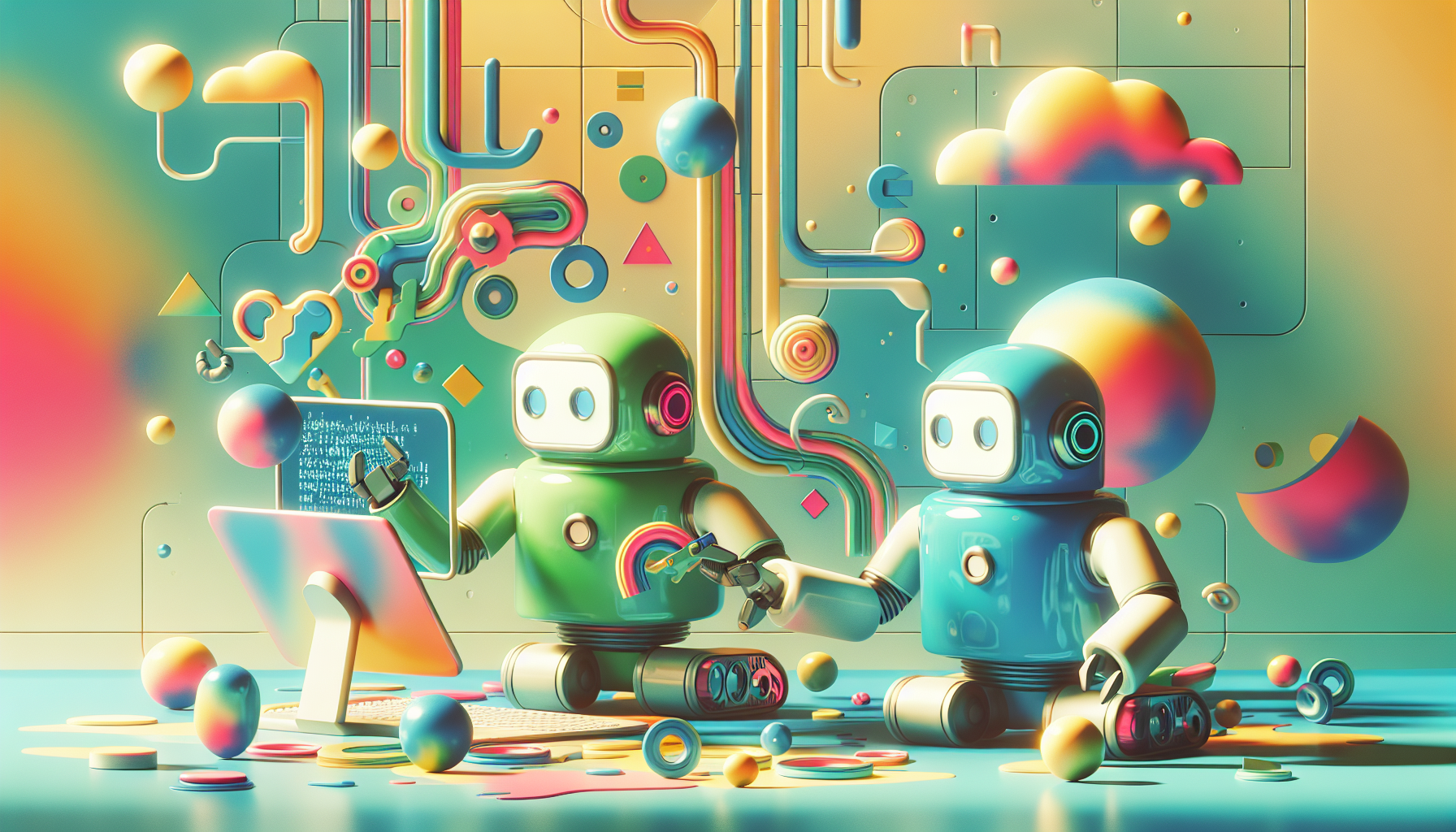
How to Solve reCAPTCHA v2 with Rust
Learn how to solve reCaptcha v2 using Rust and the Capsolver API. This guide covers both proxy and proxyless methods, providing step-by-step instructions and code examples for integrating reCaptcha v2 solving into your Rust applications.
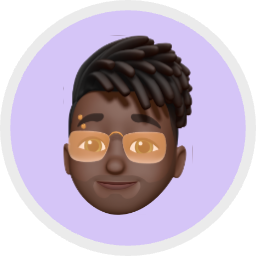
Lucas Mitchell
17-Oct-2024
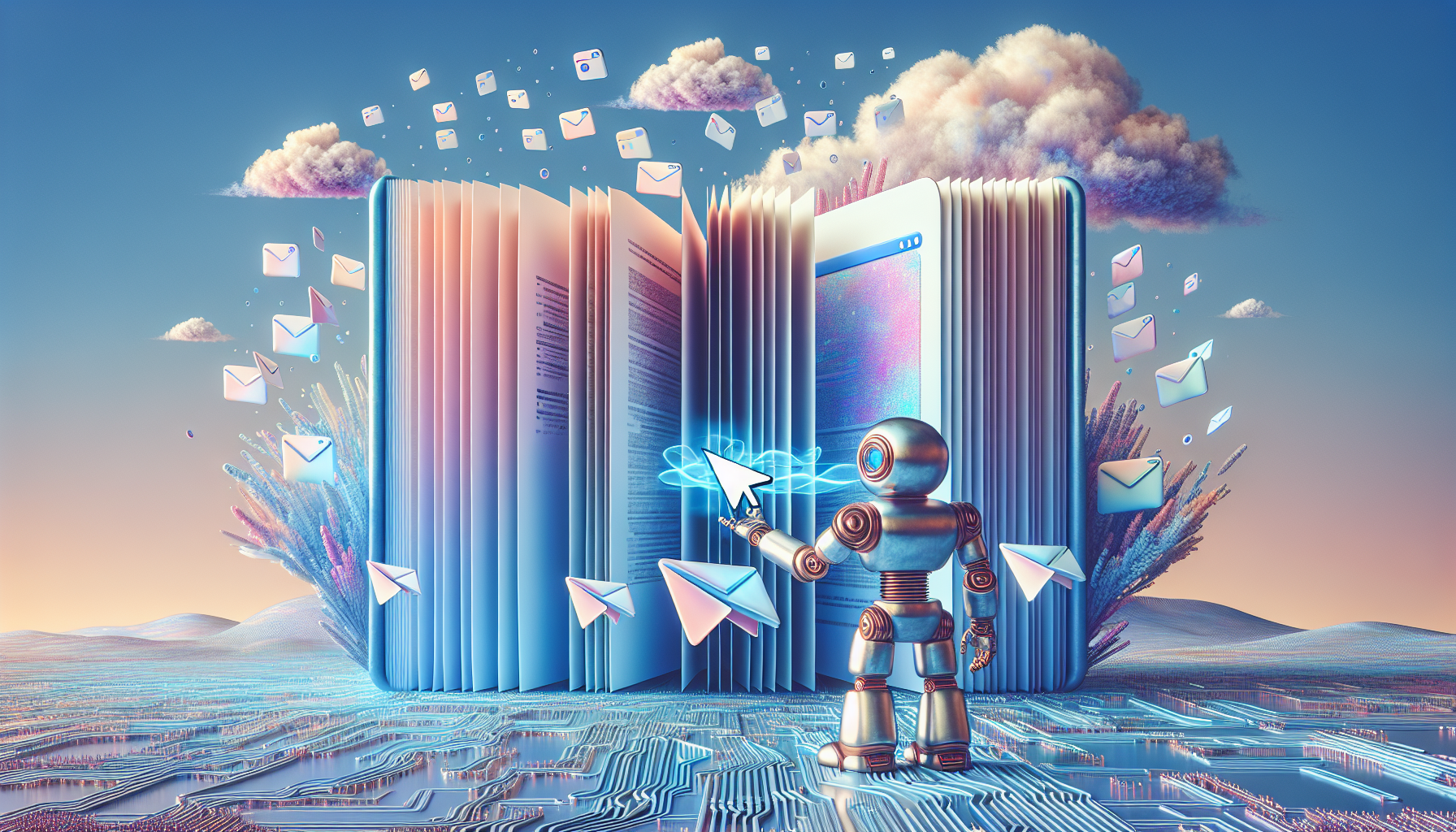
How to Use BrowserForge: A Comprehensive Guide
Learn how to use BrowserForge, a Python library for web scraping and browser automation. This comprehensive guide covers installation, header management, proxy usage, and integrating CapSolver to bypass CAPTCHAs.
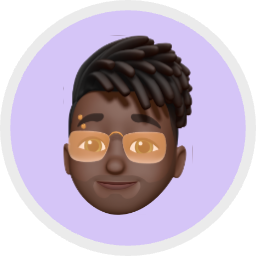
Lucas Mitchell
16-Oct-2024
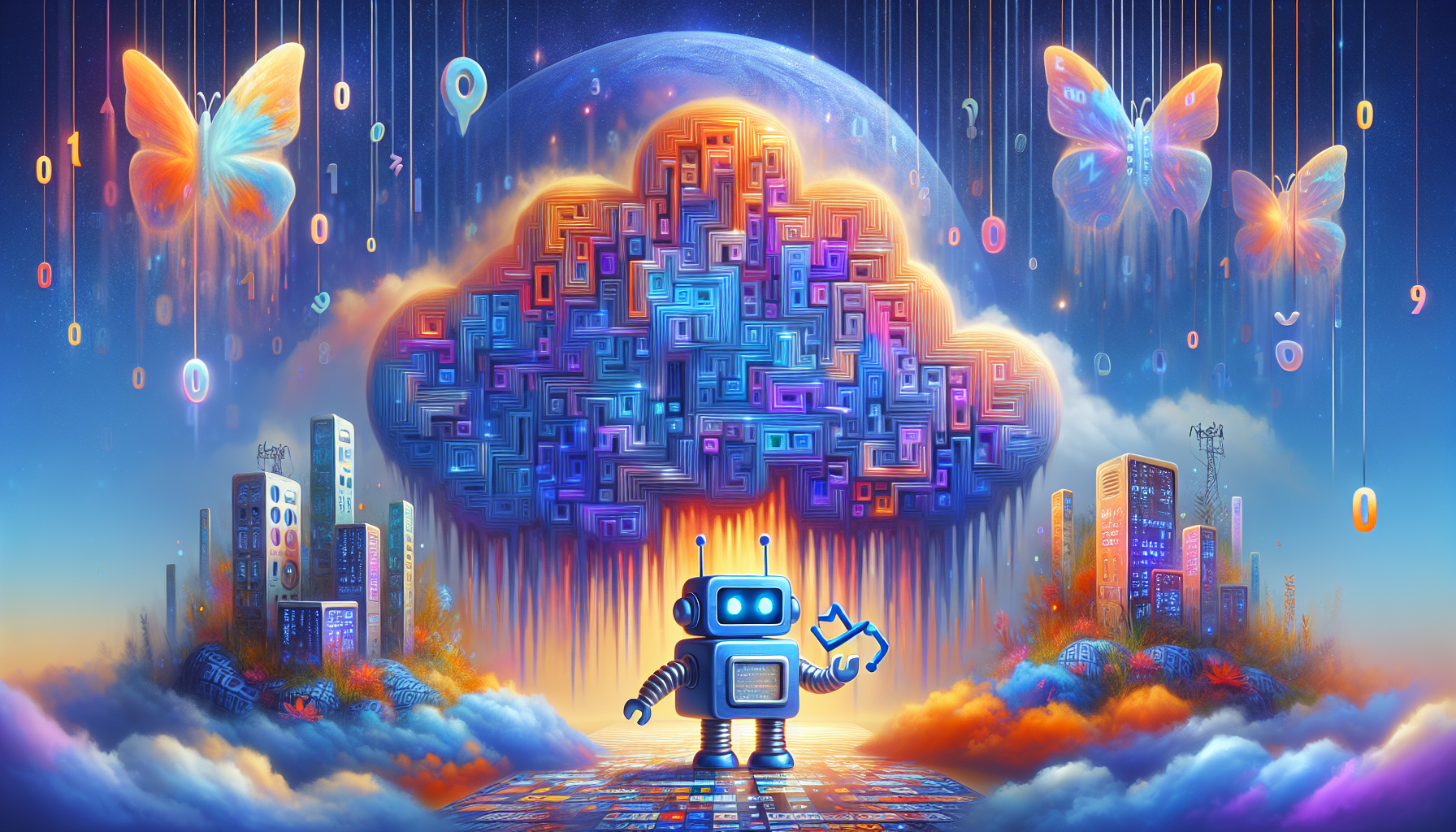
How to Solve CAPTCHA with Selenium and Node.js when Scraping
If youâre facing continuous CAPTCHA issues in your scraping efforts, consider using some tools and their advanced technology to ensure you have a reliable solution
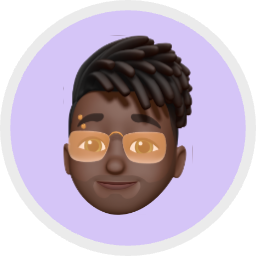
Lucas Mitchell
15-Oct-2024
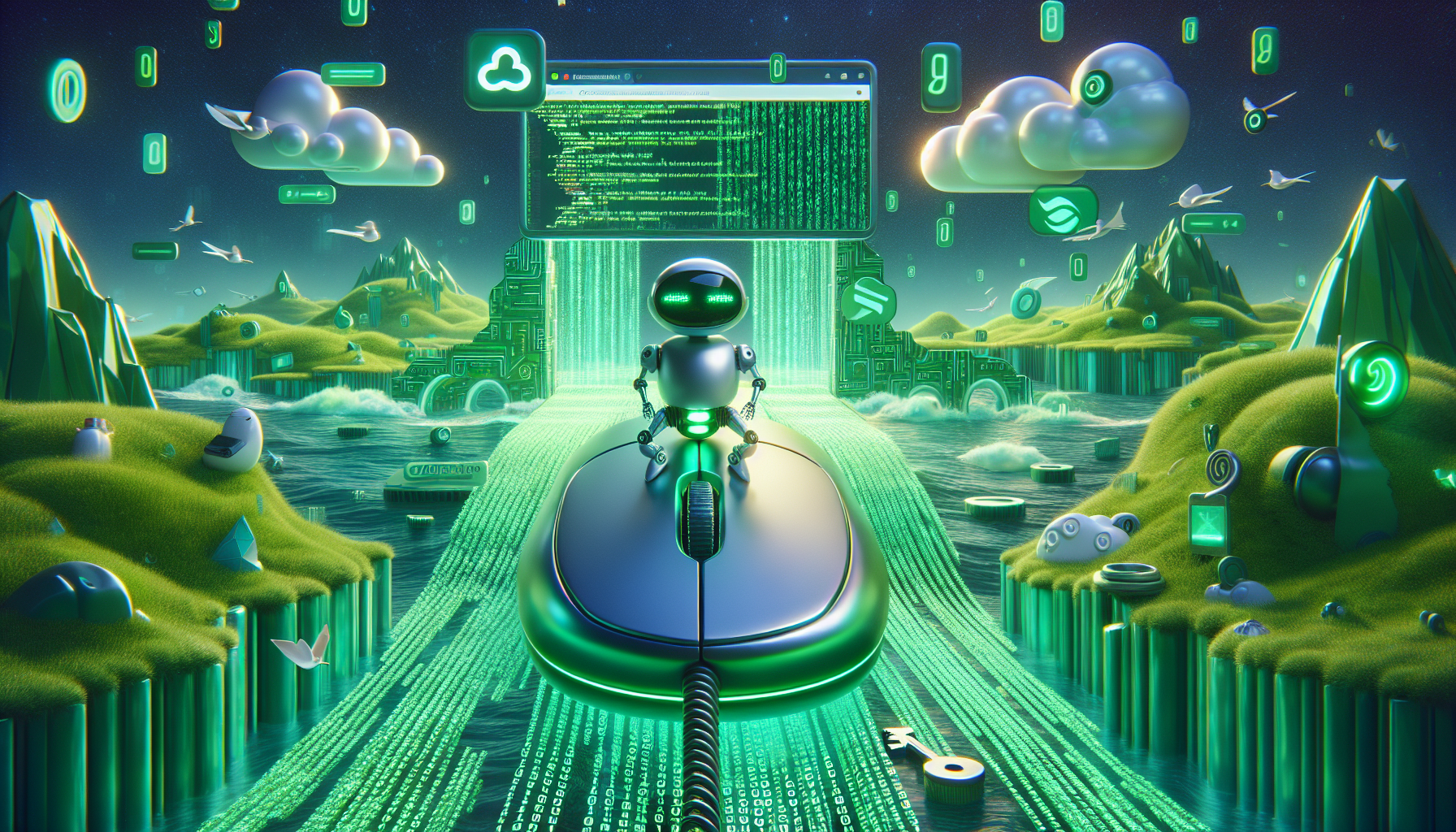
How to Solve Cloudflare Turnstile Captchas With Selenium
In this blog, weâll discuss several effective techniques for overcoming Cloudflare Turnstile Captchas using Selenium
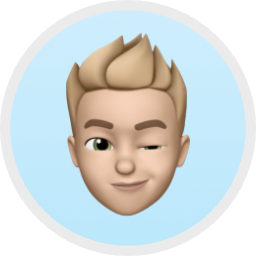
Ethan Collins
11-Oct-2024
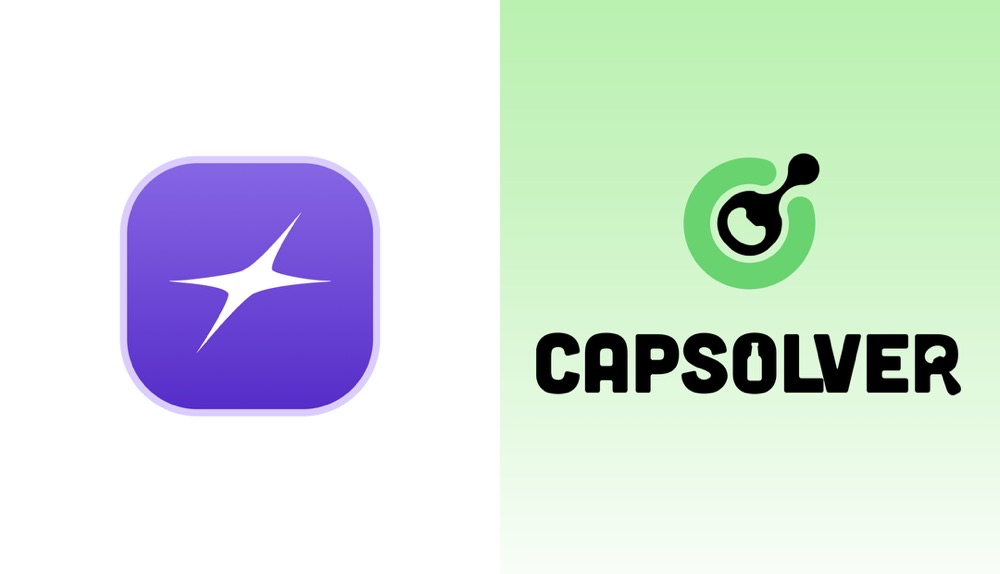
FlashProxy: Powering the Internet with Advanced Proxy Solutions
FlashProxy is one of the well-established proxy service providers, offering a wide suite of proxy solutions to fit the different online requirements.
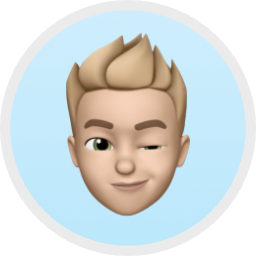
Ethan Collins
11-Oct-2024