How to Use curl_cffi for Web Scraping
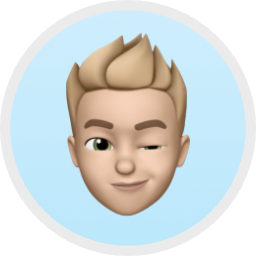
Ethan Collins
Pattern Recognition Specialist
17-Sep-2024
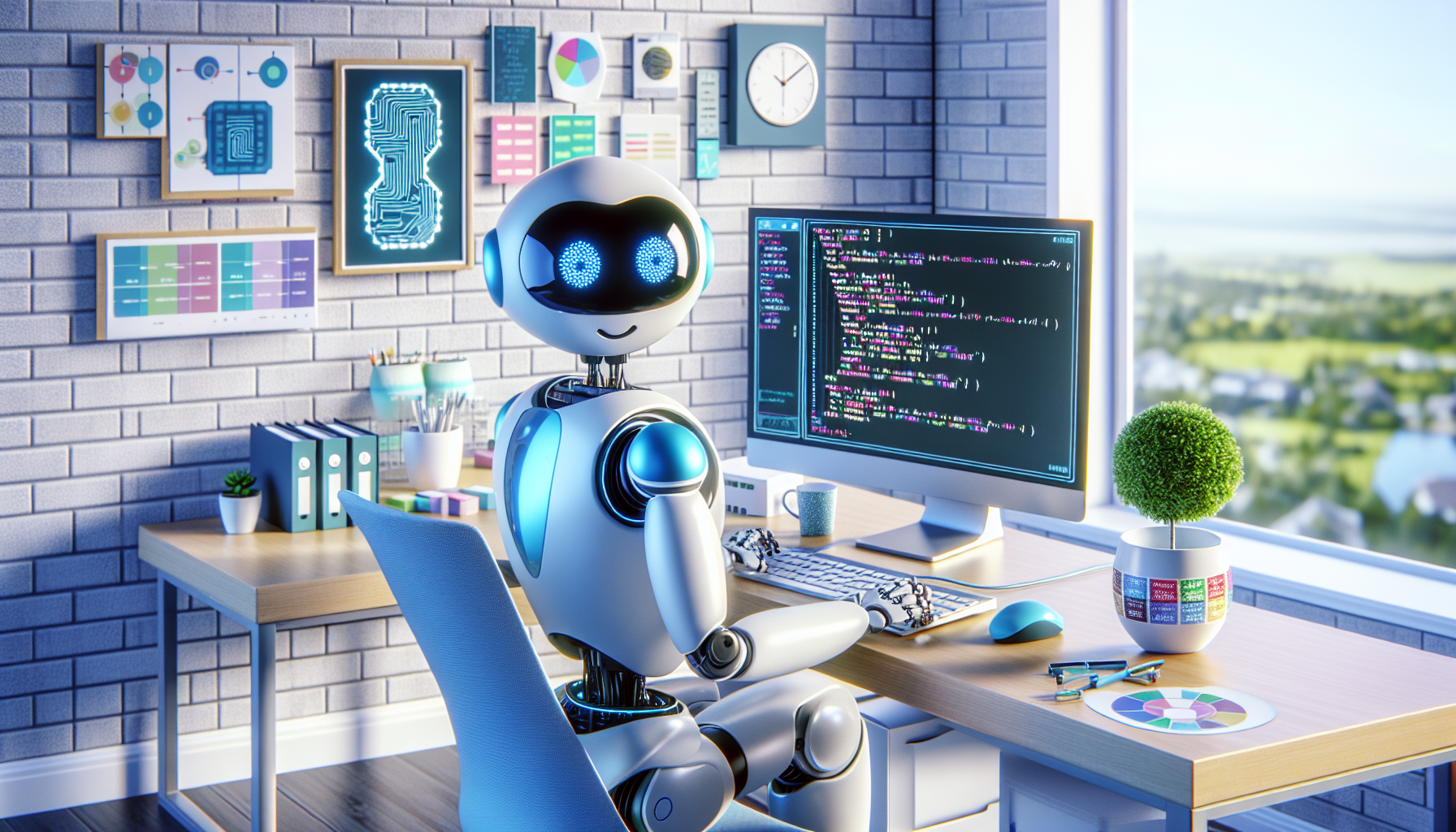
What is curl_cffi?
curl_cffi is a Python library that provides efficient, low-level bindings to the libcurl
library using CFFI (C Foreign Function Interface). This allows you to perform HTTP requests with high performance and fine-grained control, similar to the curl
command-line tool but within Python. It's particularly useful for web scraping tasks that require speed and advanced configurations.
Features:
- High Performance: Leverages the speed of
libcurl
for fast HTTP requests. - Thread Safety: Safe to use in multi-threaded applications.
- Advanced Features: Supports proxies, SSL/TLS configurations, custom headers, and more.
- Low-Level Control: Provides detailed control over the request and response process.
Prerequisites
Before you dive into using curl_cffi
, ensure you have the following installed:
- Python 3.6 or higher
- libcurl installed on your system
- pip for installing Python packages
On Ubuntu/Debian systems, you might need to install libcurl
development headers:
bash
sudo apt-get install libcurl4-openssl-dev
Getting Started with curl_cffi
Installation
Install curl_cffi
using pip
:
bash
pip install curl_cffi
Basic Example: Making a GET Request
Here's a basic example of how to use curl_cffi
to perform a GET request:
python
from curl_cffi import requests
# Perform a GET request
response = requests.get('https://httpbin.org/get')
# Check the status code
print(f'Status Code: {response.status_code}')
# Print the response content
print('Response Body:', response.text)
Web Scraping Example: Scraping Quotes from a Website
Let's scrape a webpage to extract information. We'll use Quotes to Scrape to get all the quotes along with their authors.
python
from curl_cffi import requests
from bs4 import BeautifulSoup
# URL to scrape
url = 'http://quotes.toscrape.com/'
# Perform a GET request
response = requests.get(url)
# Parse the HTML content using BeautifulSoup
soup = BeautifulSoup(response.content, 'html.parser')
# Find all quote elements
quotes = soup.find_all('div', class_='quote')
# Extract and display the quotes and authors
for quote in quotes:
text = quote.find('span', class_='text').get_text()
author = quote.find('small', class_='author').get_text()
print(f'{text} — {author}')
Output:
“The world as we have created it is a process of our thinking. It cannot be changed without changing our thinking.” — Albert Einstein
“It is our choices, Harry, that show what we truly are, far more than our abilities.” — J.K. Rowling
... (additional quotes)
Handling Captchas with CapSolver and curl_cffi
In this section, we'll explore how to integrate CapSolver with curl_cffi
to bypass captchas. CapSolver is an external service that helps in solving various types of captchas, including ReCaptcha V2, which are commonly used on websites.
We will demonstrate solving ReCaptcha V2 using CapSolver and then scraping the content of a page that requires solving the captcha first.
Example: Solving ReCaptcha V2 with CapSolver and curl_cffi
python
import os
import capsolver
from curl_cffi import requests
# Consider using environment variables for sensitive information
capsolver.api_key = os.getenv("CAPSOLVER_API_KEY", "Your CapSolver API Key")
PAGE_URL = os.getenv("PAGE_URL", "https://example.com") # URL of the page with the captcha
PAGE_KEY = os.getenv("PAGE_SITE_KEY", "SITE_KEY") # Site key for the captcha
def solve_recaptcha_v2(url, site_key):
solution = capsolver.solve({
"type": "ReCaptchaV2TaskProxyless",
"websiteURL": url,
"websiteKey": site_key,
"proxy": PROXY
})
return solution['solution']['gRecaptchaResponse']
def main():
print("Solving reCaptcha V2...")
captcha_solution = solve_recaptcha_v2(PAGE_URL, PAGE_KEY)
print("Captcha Solved!")
if __name__ == "__main__":
main()
Note: Replace PAGE_URL
with the URL of the page containing the captcha and PAGE_SITE_KEY
with the site key of the captcha. You can find the site key in the HTML source of the page, usually within the <div>
containing the captcha widget.
Handling Proxies with curl_cffi
If you need to route your requests through a proxy, curl_cffi
makes it straightforward:
python
from curl_cffi import requests
# Define the proxy settings
proxies = {
'http': 'http://username:password@proxyserver:port',
'https': 'https://username:password@proxyserver:port',
}
# Perform a GET request using the proxy
response = requests.get('https://httpbin.org/ip', proxies=proxies)
# Print the response content
print('Response Body:', response.text)
Handling Cookies with curl_cffi
You can manage cookies using the CookieJar
from Python's http.cookiejar
module:
python
from curl_cffi import requests
from http.cookiejar import CookieJar
# Create a CookieJar instance
cookie_jar = CookieJar()
# Create a session with the cookie jar
session = requests.Session()
session.cookies = cookie_jar
# Perform a GET request
response = session.get('https://httpbin.org/cookies/set?name=value')
# Display the cookies
for cookie in session.cookies:
print(f'{cookie.name}: {cookie.value}')
Advanced Usage: Custom Headers and POST Requests
You can send custom headers and perform POST requests with curl_cffi
:
python
from curl_cffi import requests
# Define custom headers
headers = {
'User-Agent': 'Mozilla/5.0 (compatible)',
'Accept-Language': 'en-US,en;q=0.5',
}
# Data to send in the POST request
data = {
'username': 'testuser',
'password': 'testpass',
}
# Perform a POST request
response = requests.post('https://httpbin.org/post', headers=headers, data=data)
# Print the JSON response
print('Response JSON:', response.json())
Bonus Code
Claim your Bonus Code for top captcha solutions at CapSolver: scrape. After redeeming it, you will get an extra 5% bonus after each recharge, unlimited times.
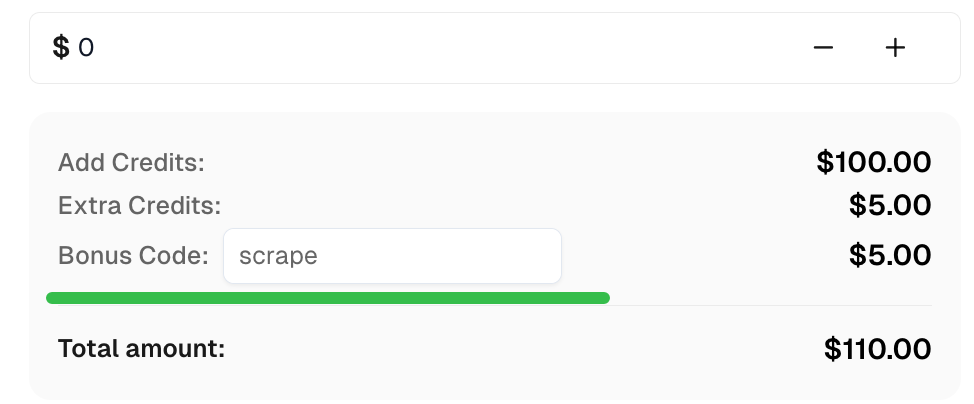
Conclusion
With curl_cffi
, you can efficiently perform web scraping tasks while having detailed control over your HTTP requests. Integrating it with CapSolver allows you to bypass captchas like ReCaptcha V2 , enabling access to content that would otherwise be difficult to scrape.
Feel free to expand upon these examples to suit your specific scraping needs. Always remember to respect the terms of service of the websites you scrape and adhere to legal guidelines.
Happy scraping!
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
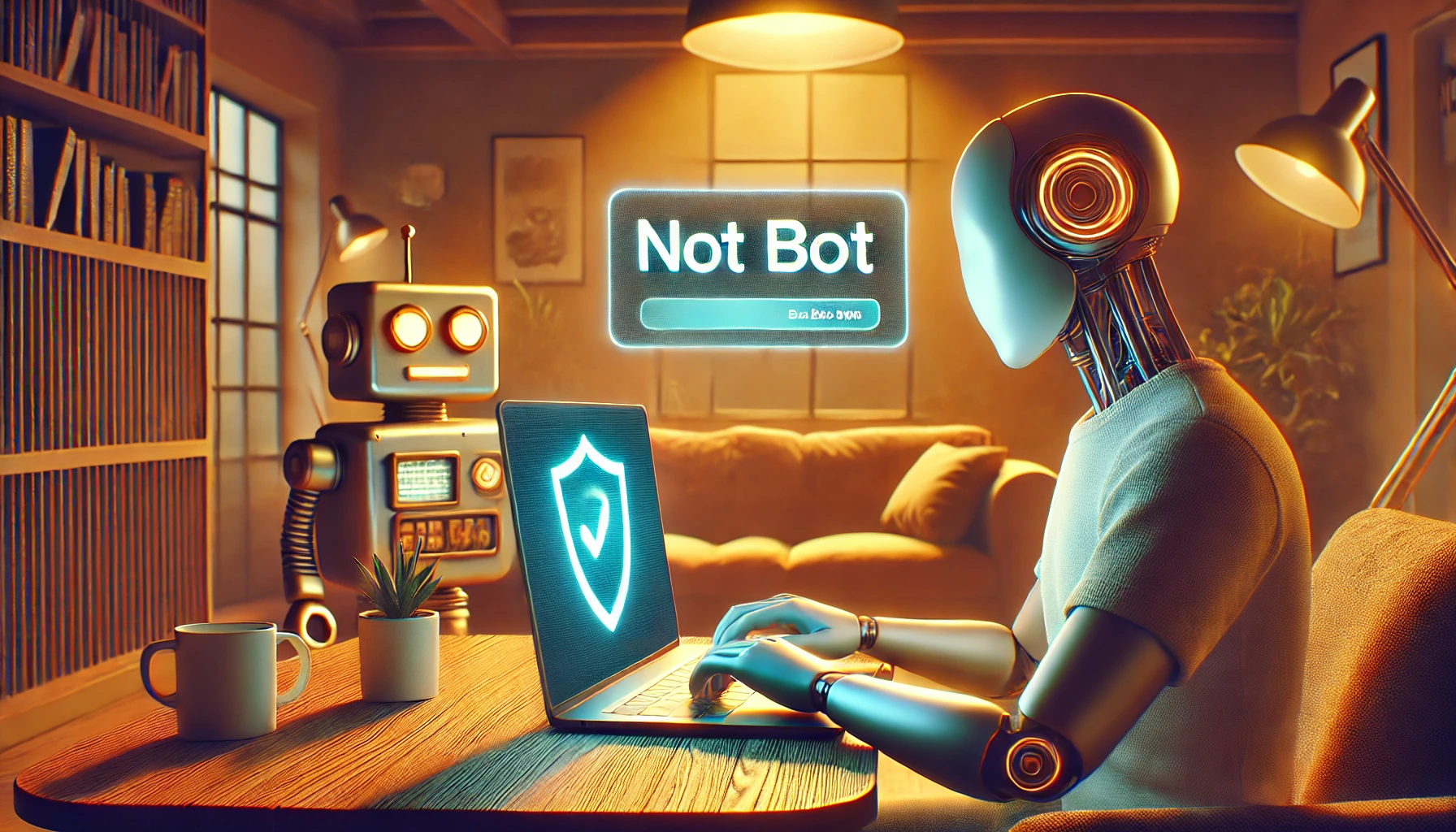
Why do I keep getting asked to verify I'm not a robot?
Learn why Google prompts you to verify you're not a robot and explore solutions like using CapSolver’s API to solve CAPTCHA challenges efficiently.
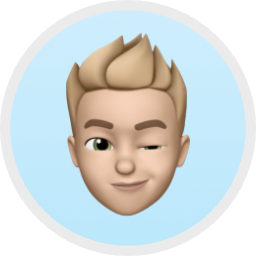
Ethan Collins
27-Feb-2025
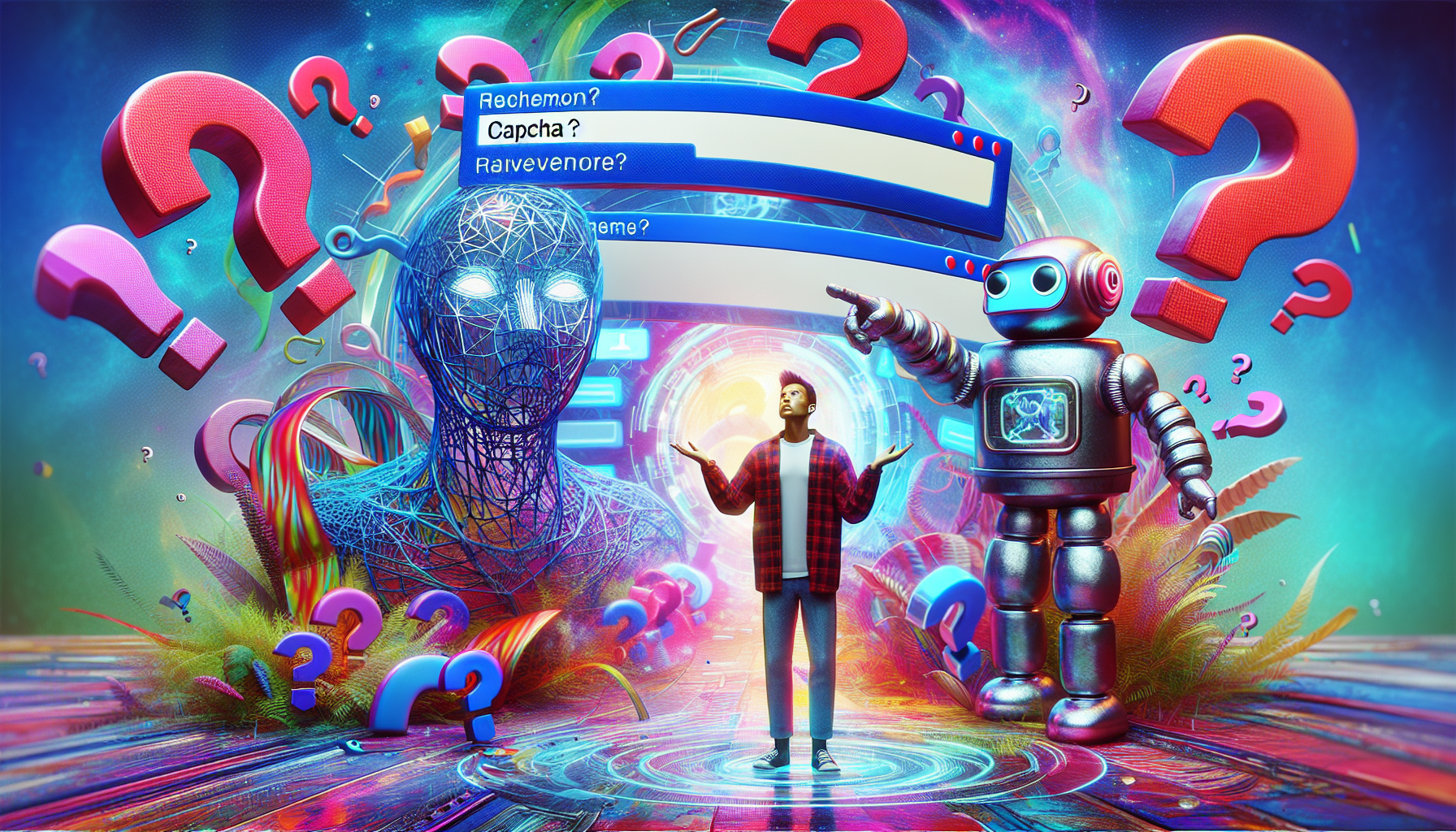
Why Do Websites Think I'm a Bot? And How to Solve Them
Understand why websites flag you as a bot and how to avoid detection. Key triggers include CAPTCHA challenges, suspicious IPs, and unusual browser behavior.
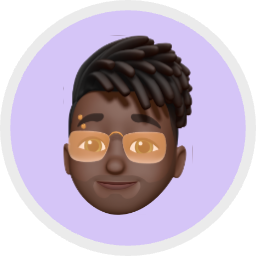
Lucas Mitchell
20-Feb-2025
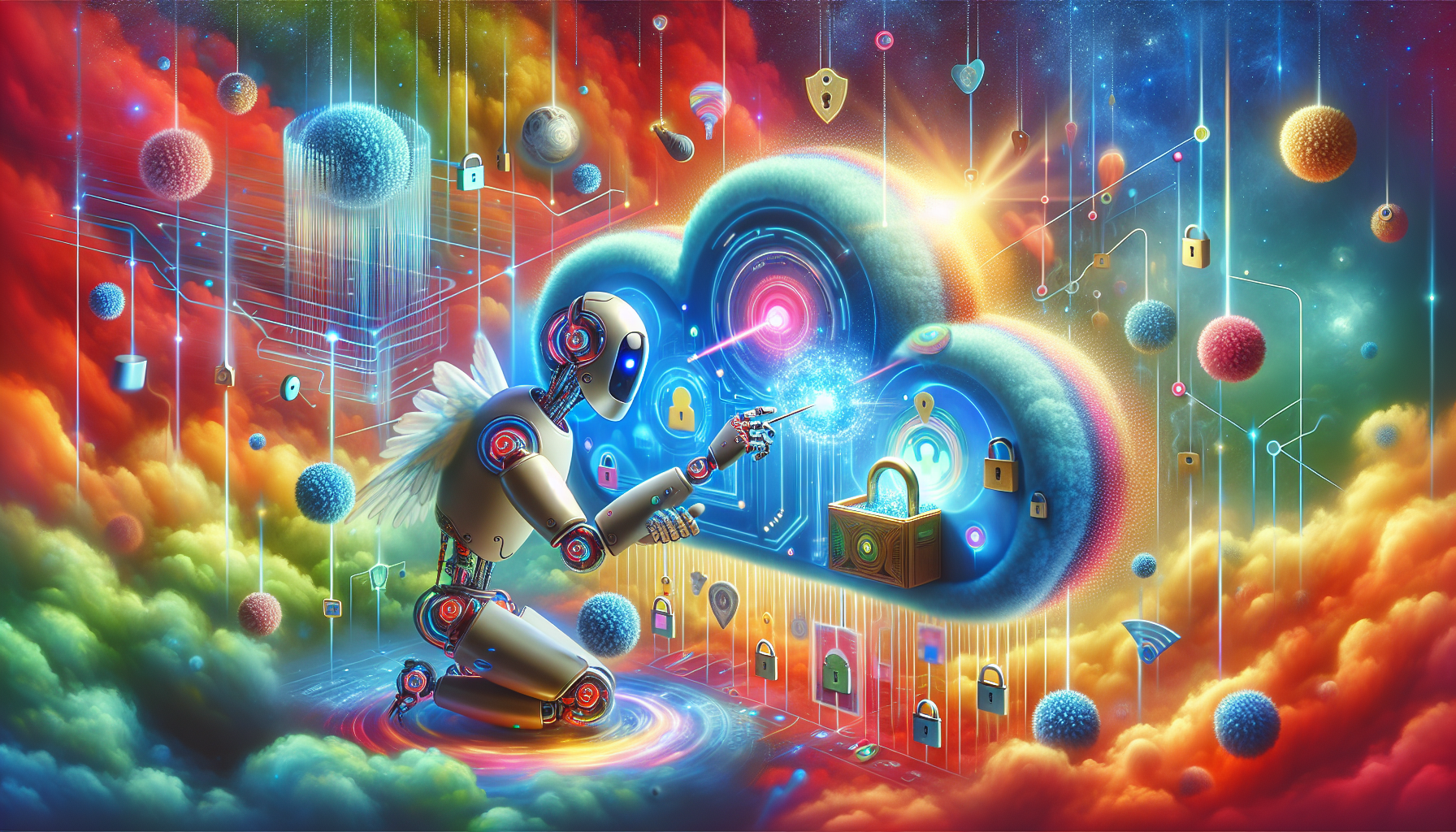
How to Extract Data from a Cloudflare-Protected Website
In this guide, we'll explore ethical and effective techniques to extract data from Cloudflare-protected websites.
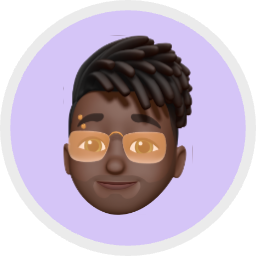
Lucas Mitchell
20-Feb-2025
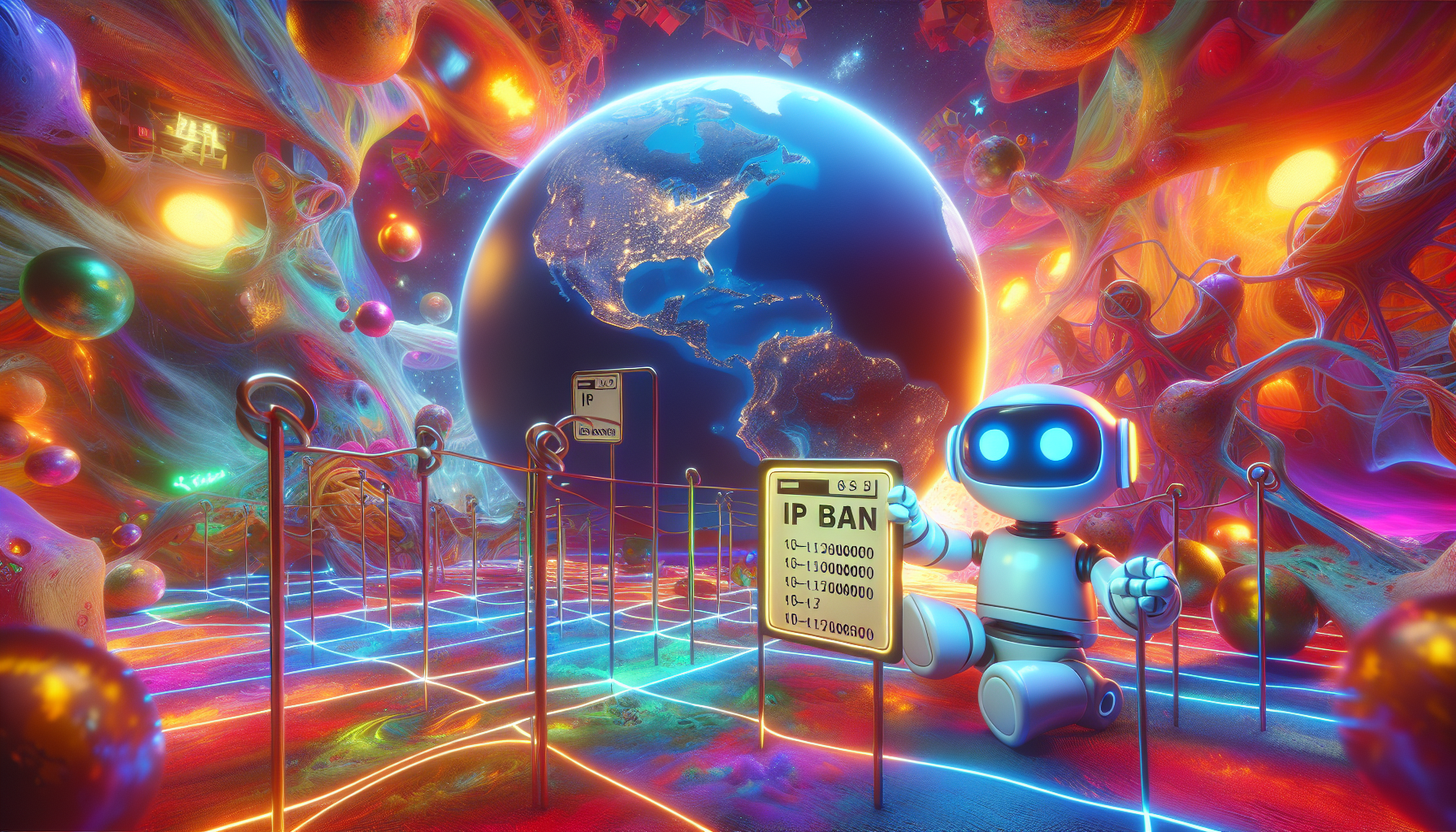
How to Avoid IP Bans when Using Captcha Solver in 2025
Learn effective strategies to prevent IP bans when using captcha solvers in 2025. Explore the best practices, tools, and techniques to safely and efficiently solve CAPTCHA challenges.
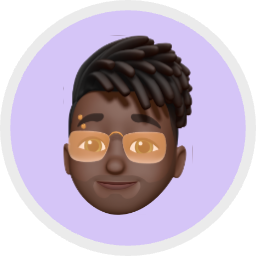
Lucas Mitchell
18-Feb-2025
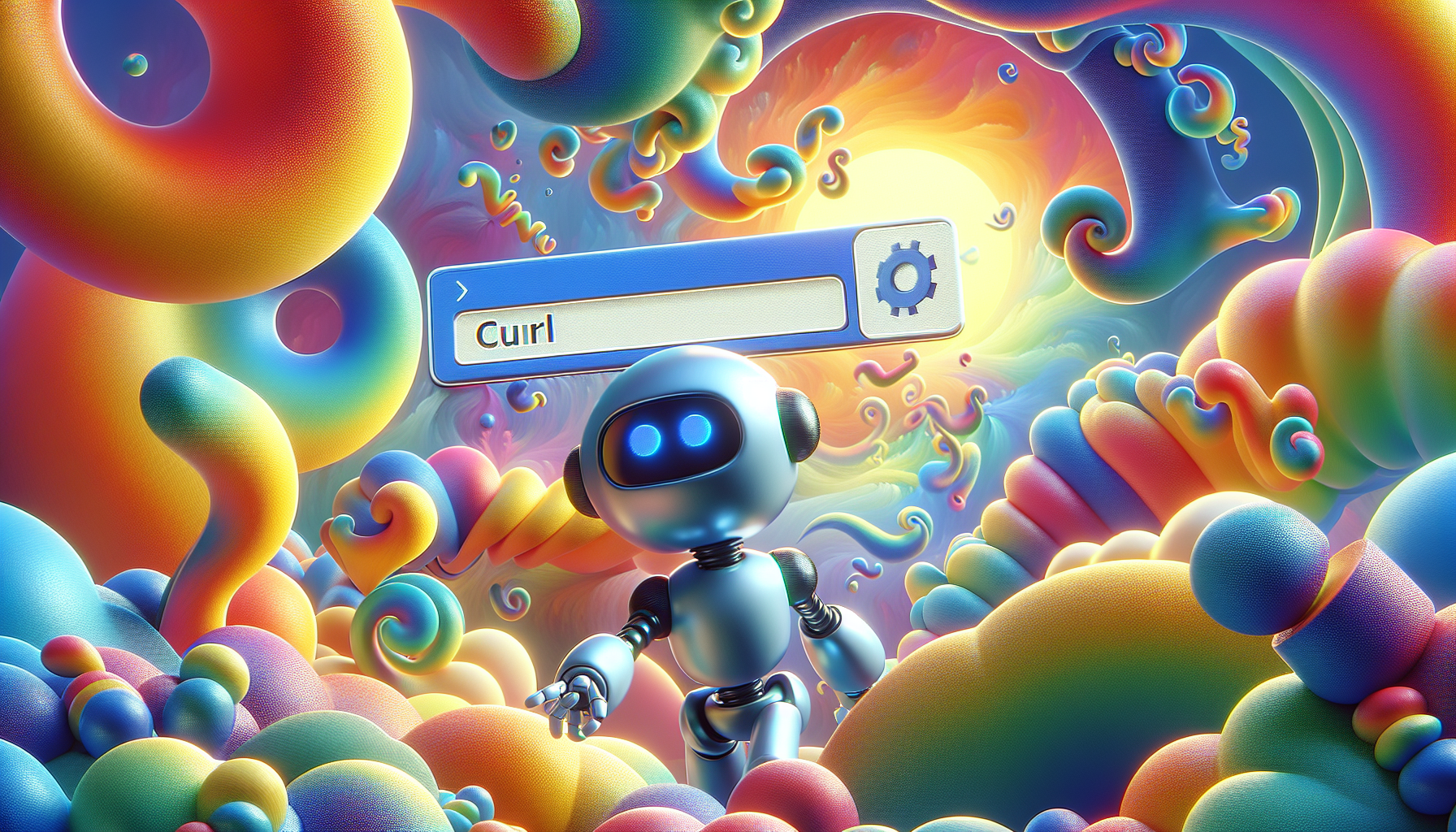
Solving CAPTCHA with cURL: A Step-by-Step Guide
Learn how to solve CAPTCHA using cURL with simple steps and automation techniques.
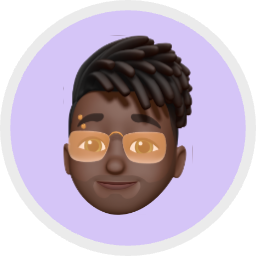
Lucas Mitchell
18-Feb-2025
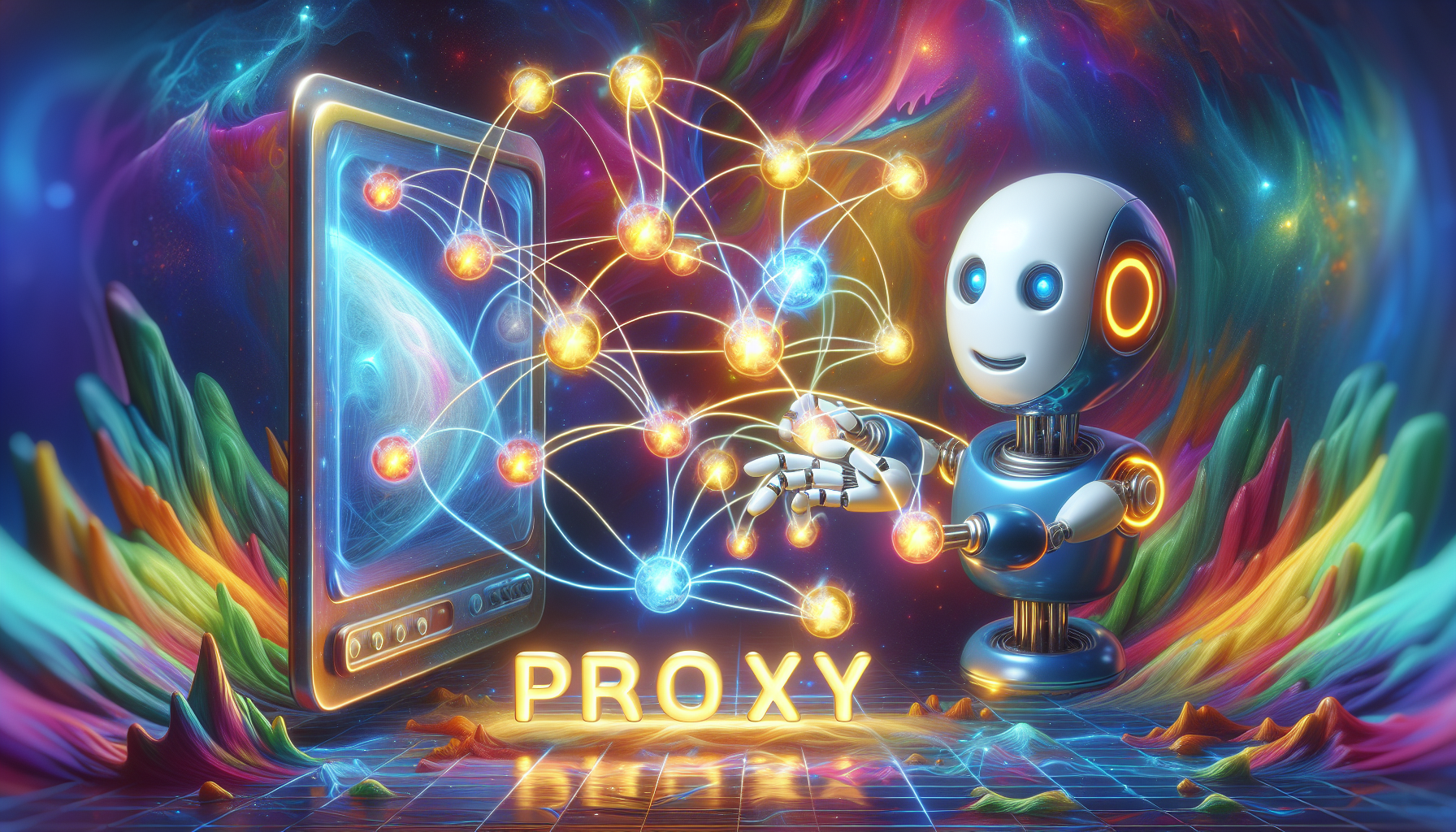
How to Set Up Proxies for CAPTCHA Solving
Discover how to set up proxies for CAPTCHA solving using CapSolver. This comprehensive guide explains proxy configurations, task types, and integration strategies for efficient web scraping and automation while bypassing CAPTCHA challenges.
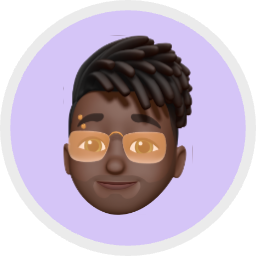
Lucas Mitchell
17-Feb-2025