How to solve MTCaptcha with Node.JS
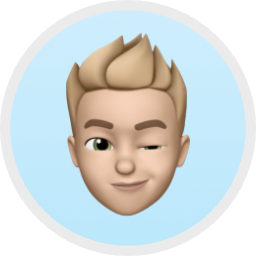
Ethan Collins
Pattern Recognition Specialist
27-Sep-2023
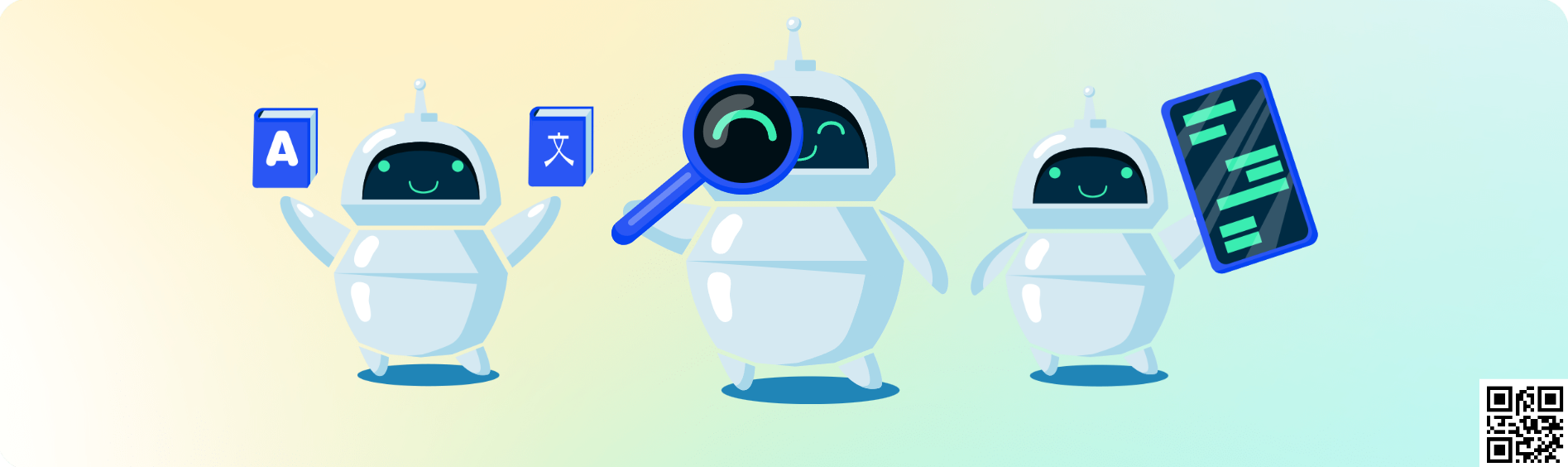
⚙️ Prerequisites
- Proxy (Optional)
- Node.JS installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
python
npm install axios
👨💻 Node.JS Code for solve Mtcaptcha without proxy
Here's a Node.JS sample script to accomplish the task:
js
const axios = require('axios');
const PAGE_URL = ""; // Replace with your Website
const SITE_KEY = ""; // Replace with your Website
const CLIENT_KEY = ""; // Replace with your CAPSOLVER API Key
async function createTask(payload) {
try {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: CLIENT_KEY,
task: payload
});
return res.data;
} catch (error) {
console.error(error);
}
}
async function getTaskResult(taskId) {
try {
success = false;
while(success == false){
await sleep(1000);
console.log("Getting task result for task ID: " + taskId);
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: CLIENT_KEY,
taskId: taskId
});
if( res.data.status == "ready") {
success = true;
console.log(res.data)
return res.data;
}
}
} catch (error) {
console.error(error);
return null;
}
}
async function solveMTCaptcha(pageURL, sitekey) {
const taskPayload = {
type: "MTCaptchaTaskProxyless",
websiteURL: pageURL,
websiteKey: sitekey,
};
const taskData = await createTask(taskPayload);
return await getTaskResult(taskData.taskId);
}
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function main() {
try {
const response = await solveMTCaptcha(PAGE_URL, SITE_KEY );
console.log(`Received token: ${response.solution.token}`);
}
catch (error) {
console.error(`Error: ${error}`);
}
}
main();
👨💻 Node.JS Code for solve MTCaptcha with proxy
Here's a Node.JS sample script to accomplish the task:
js
const axios = require('axios');
const PAGE_URL = ""; // Replace with your Website
const SITE_KEY = ""; // Replace with your Website
const CLIENT_KEY = ""; // Replace with your CAPSOLVER API Key
const PROXY = "https://username:password@host:port";
async function createTask(payload) {
try {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: CLIENT_KEY,
task: payload
});
return res.data;
} catch (error) {
console.error(error);
}
}
async function getTaskResult(taskId) {
try {
success = false;
while(success == false){
await sleep(1000);
console.log("Getting task result for task ID: " + taskId);
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: CLIENT_KEY,
taskId: taskId
});
if( res.data.status == "ready") {
success = true;
console.log(res.data)
return res.data;
}
}
} catch (error) {
console.error(error);
return null;
}
}
async function solveMTCaptcha(pageURL, sitekey) {
const taskPayload = {
type: "MTCaptchaTask",
websiteURL: pageURL,
websiteKey: sitekey,
proxy: PROXY
};
const taskData = await createTask(taskPayload);
return await getTaskResult(taskData.taskId);
}
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function main() {
try {
const response = await solveMTCaptcha(PAGE_URL, SITE_KEY );
console.log(`Received token: ${response.solution.token}`);
}
catch (error) {
console.error(`Error: ${error}`);
}
}
main();
⚠️ Change these variables
- PROXY: Change to your proxy, only if you use MTCaptchaTask.
- CLIENT_KEY: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve MTCaptcha
- SITE_KEY: Replace with the site key of the page with MTCaptcha
👀 More information
Compliance Disclaimer: The information provided on this blog is for informational purposes only. CapSolver is committed to compliance with all applicable laws and regulations. The use of the CapSolver network for illegal, fraudulent, or abusive activities is strictly prohibited and will be investigated. Our captcha-solving solutions enhance user experience while ensuring 100% compliance in helping solve captcha difficulties during public data crawling. We encourage responsible use of our services. For more information, please visit our Terms of Service and Privacy Policy.
More
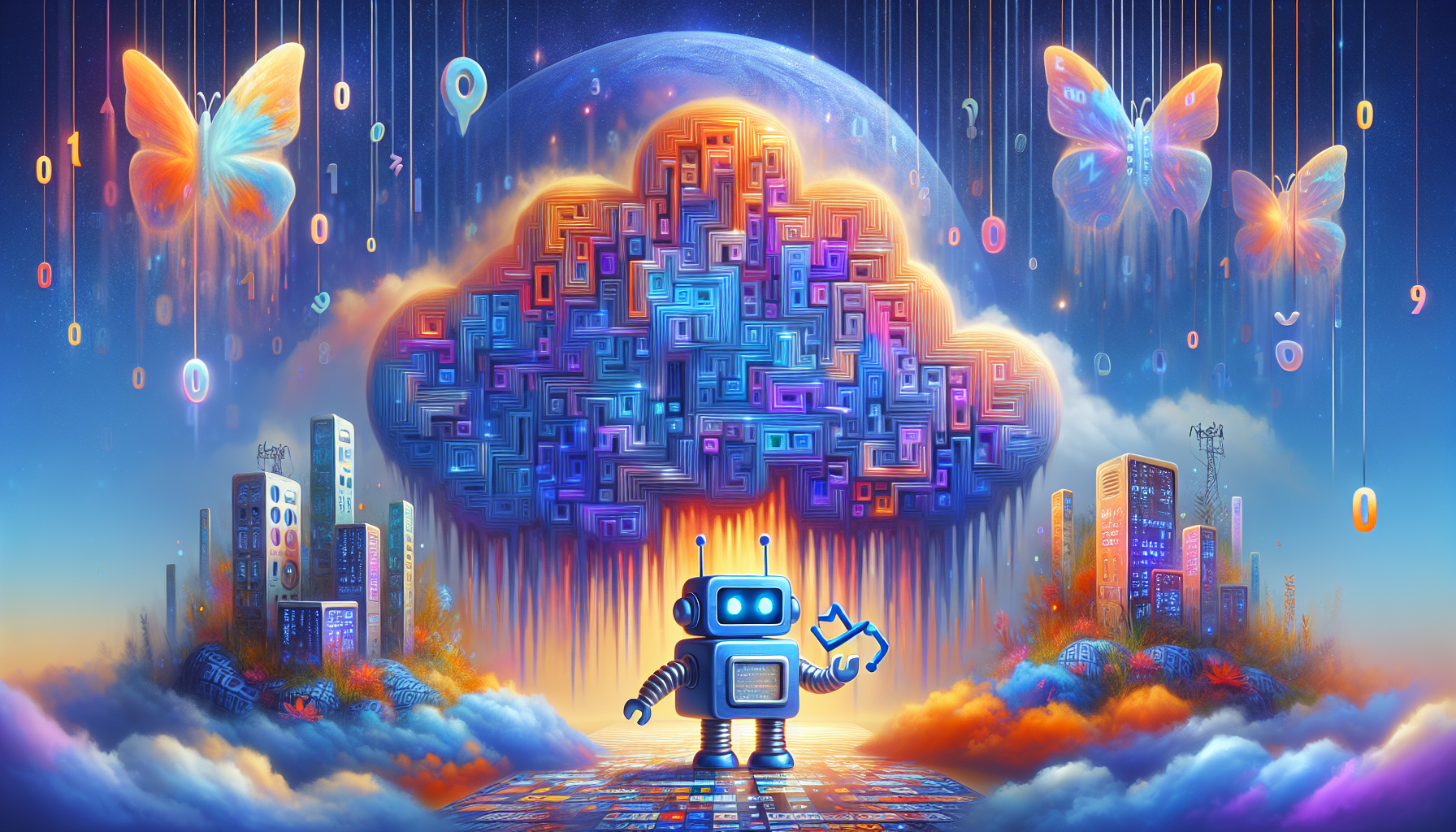
How to Solve CAPTCHA with Selenium and Node.js when Scraping
If you’re facing continuous CAPTCHA issues in your scraping efforts, consider using some tools and their advanced technology to ensure you have a reliable solution
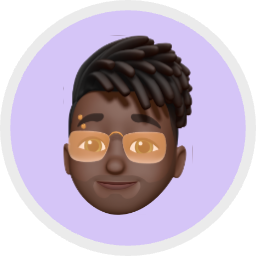
Lucas Mitchell
15-Oct-2024
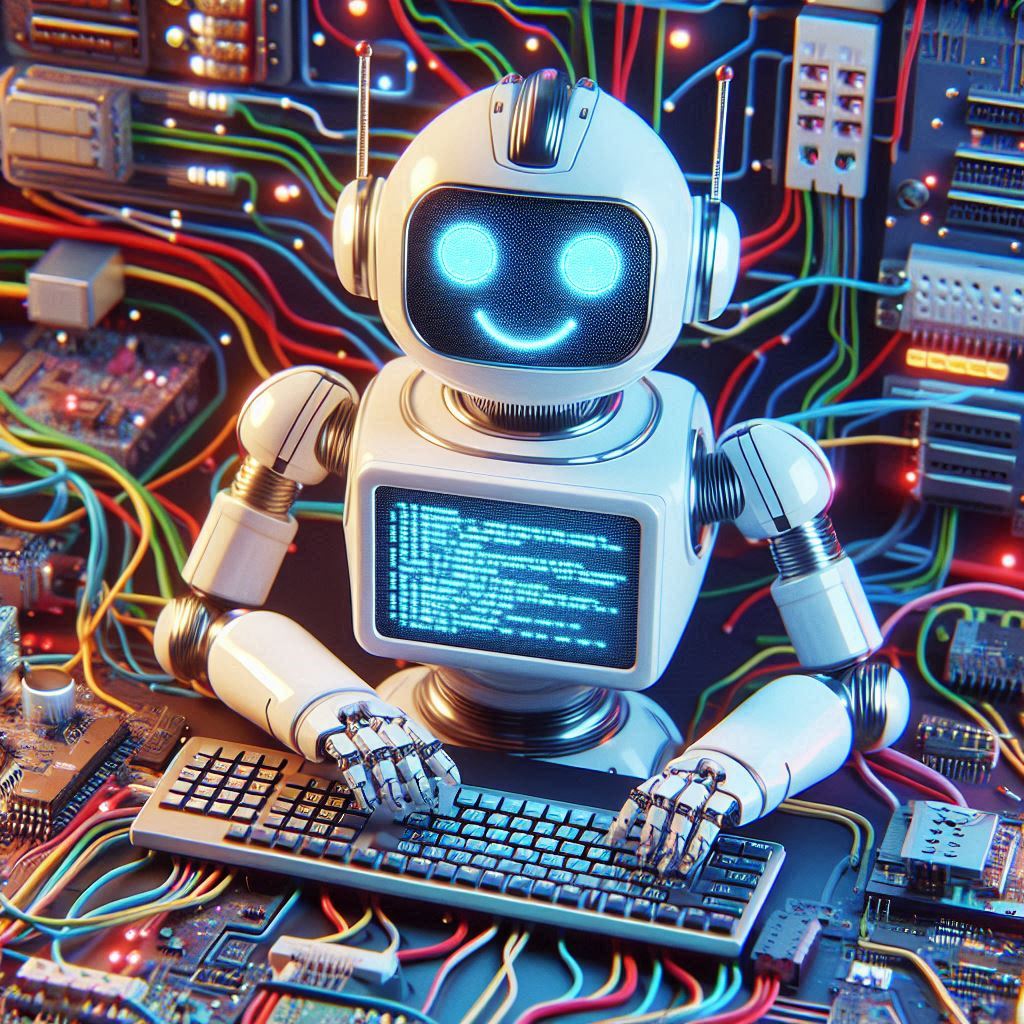
Solving 403 Forbidden Errors When Crawling Websites with Python
Learn how to overcome 403 Forbidden errors when crawling websites with Python. This guide covers IP rotation, user-agent spoofing, request throttling, authentication handling, and using headless browsers to bypass access restrictions and continue web scraping successfully.
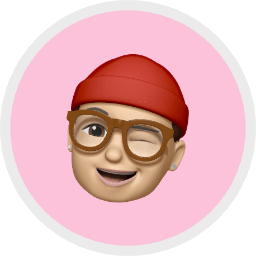
Sora Fujimoto
01-Aug-2024
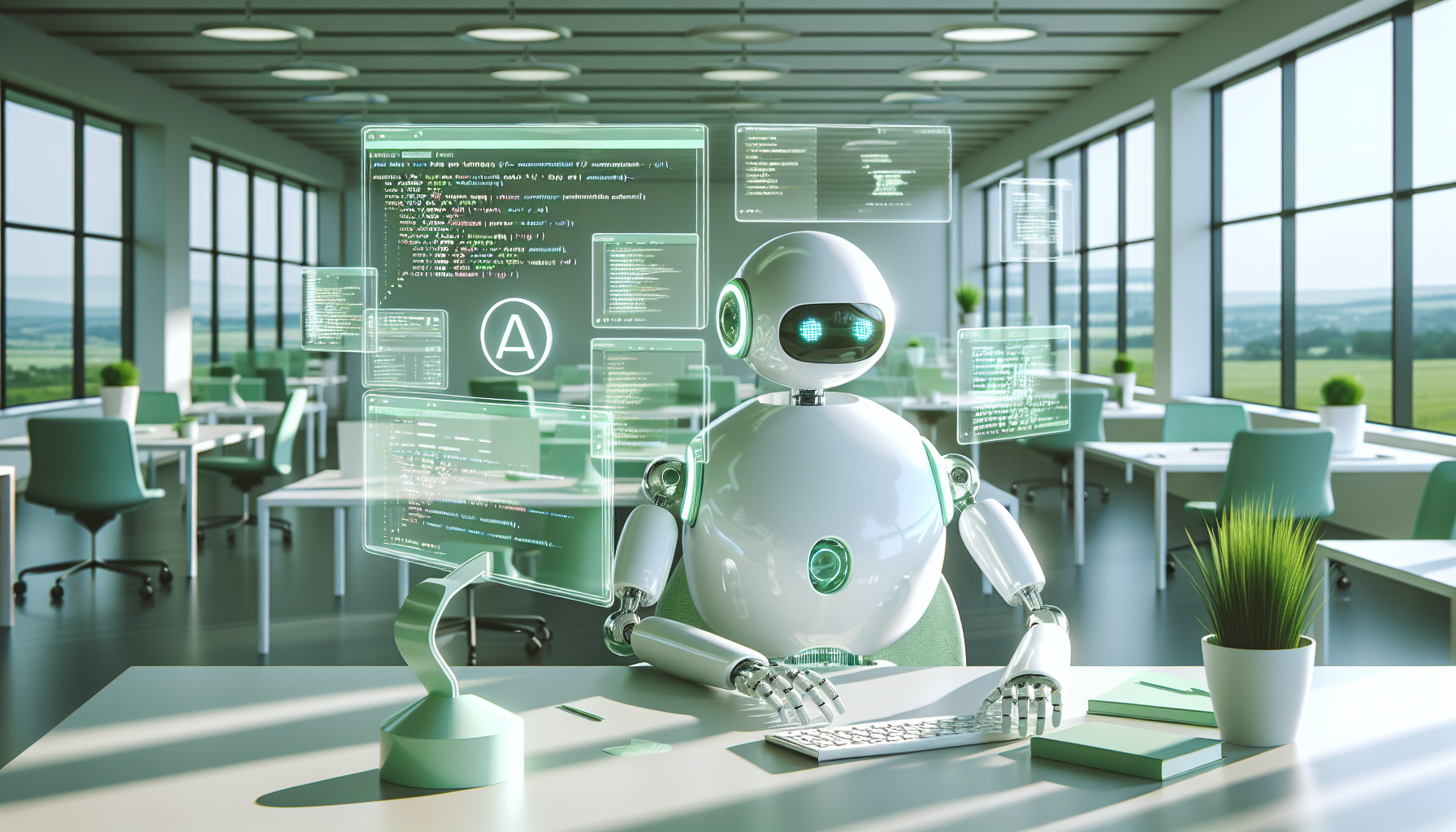
How to Use Selenium Driverless for Efficient Web Scraping
Learn how to use Selenium Driverless for efficient web scraping. This guide provides step-by-step instructions on setting up your environment, writing your first Selenium Driverless script, and handling dynamic content. Streamline your web scraping tasks by avoiding the complexities of traditional WebDriver management, making your data extraction process simpler, faster, and more portable.
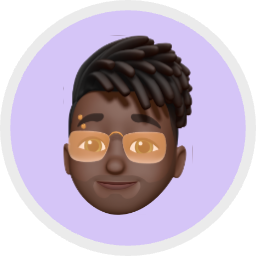
Lucas Mitchell
01-Aug-2024
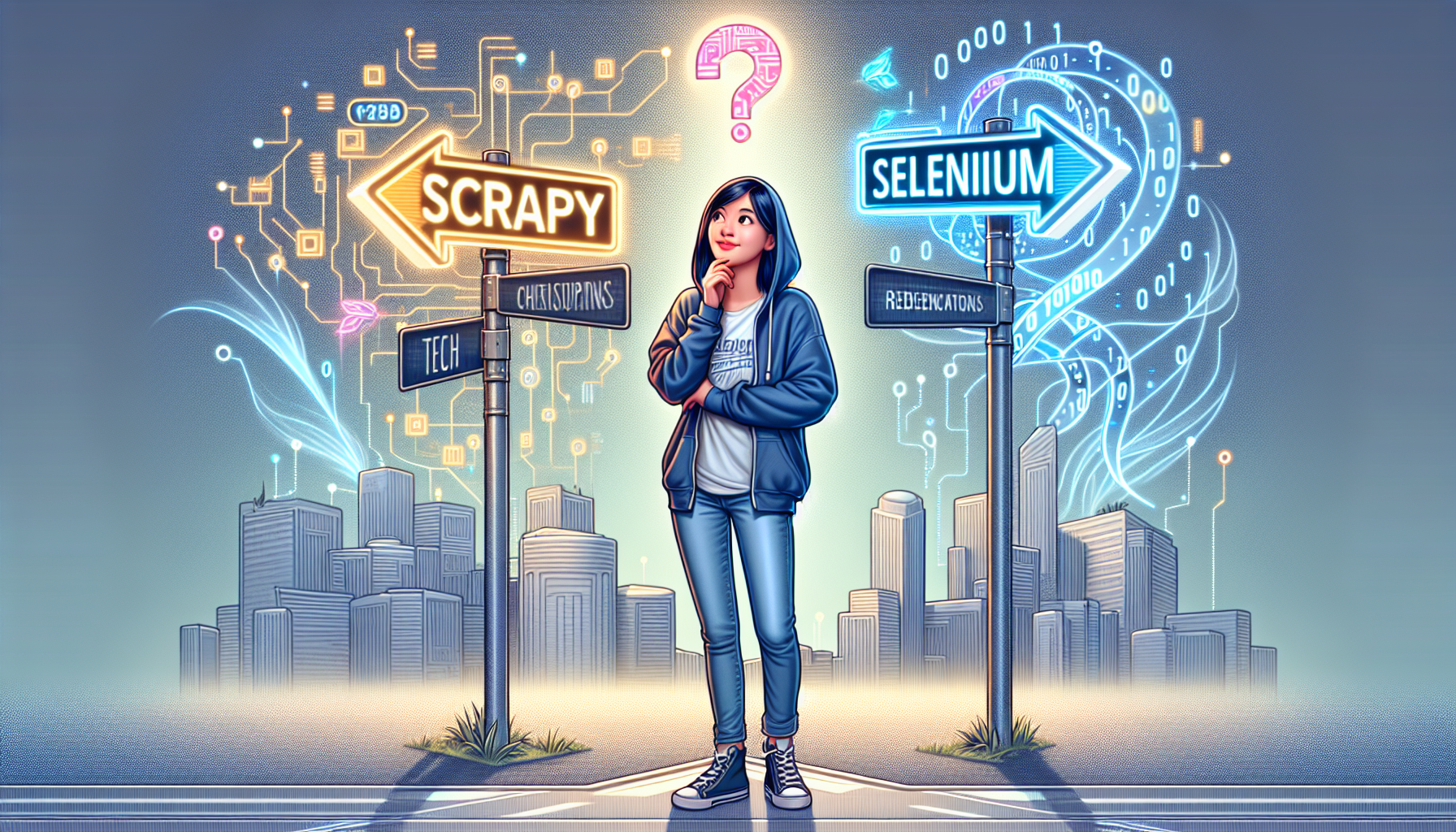
Scrapy vs. Selenium: What's Best for Your Web Scraping Project
Discover the strengths and differences between Scrapy and Selenium for web scraping. Learn which tool suits your project best and how to handle challenges like CAPTCHAs.
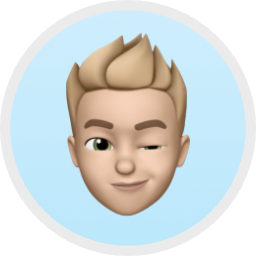
Ethan Collins
24-Jul-2024
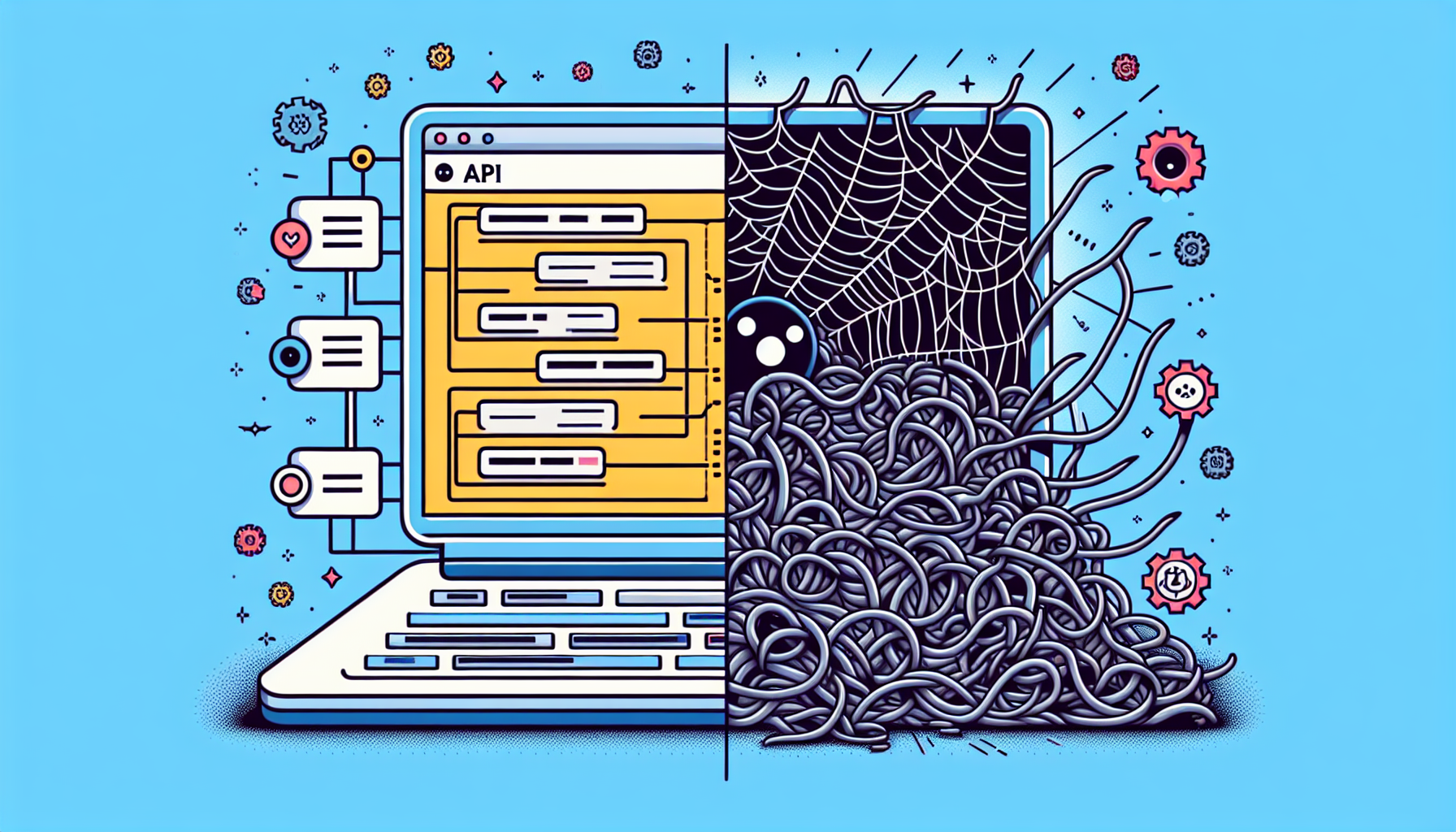
API vs Scraping : the best way to obtain the data
Understand the differences, pros, and cons of Web Scraping and API Scraping to choose the best data collection method. Explore CapSolver for bot challenge solutions.
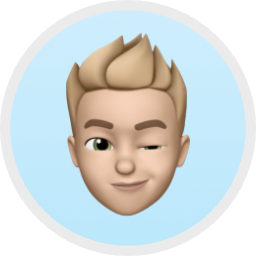
Ethan Collins
15-Jul-2024
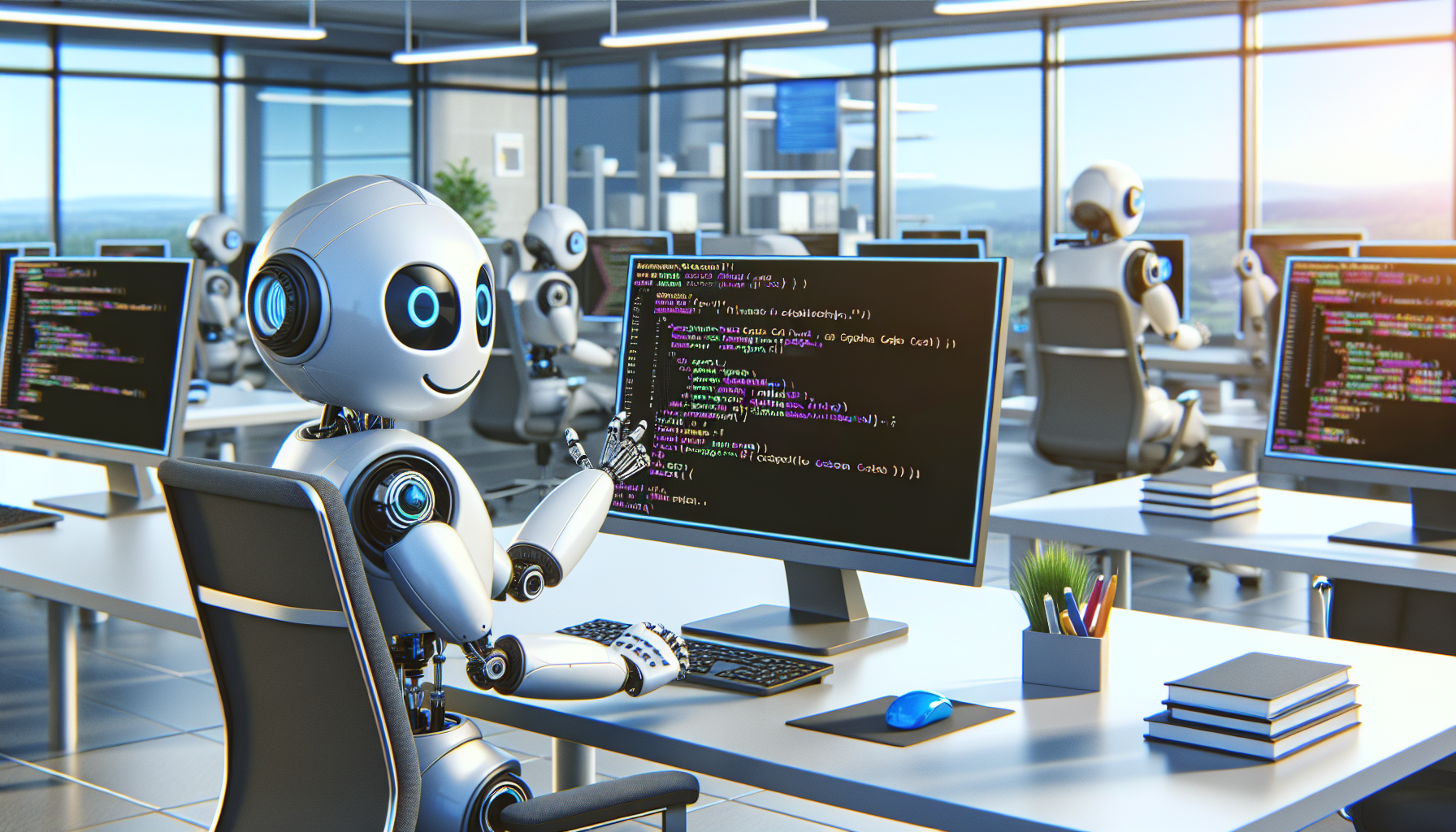
How to solve CAPTCHA With Selenium C#
At the end of this tutorial, you'll have a solid understanding of How to solve CAPTCHA With Selenium C#
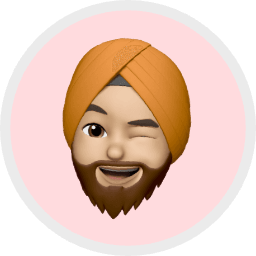
Rajinder Singh
10-Jul-2024