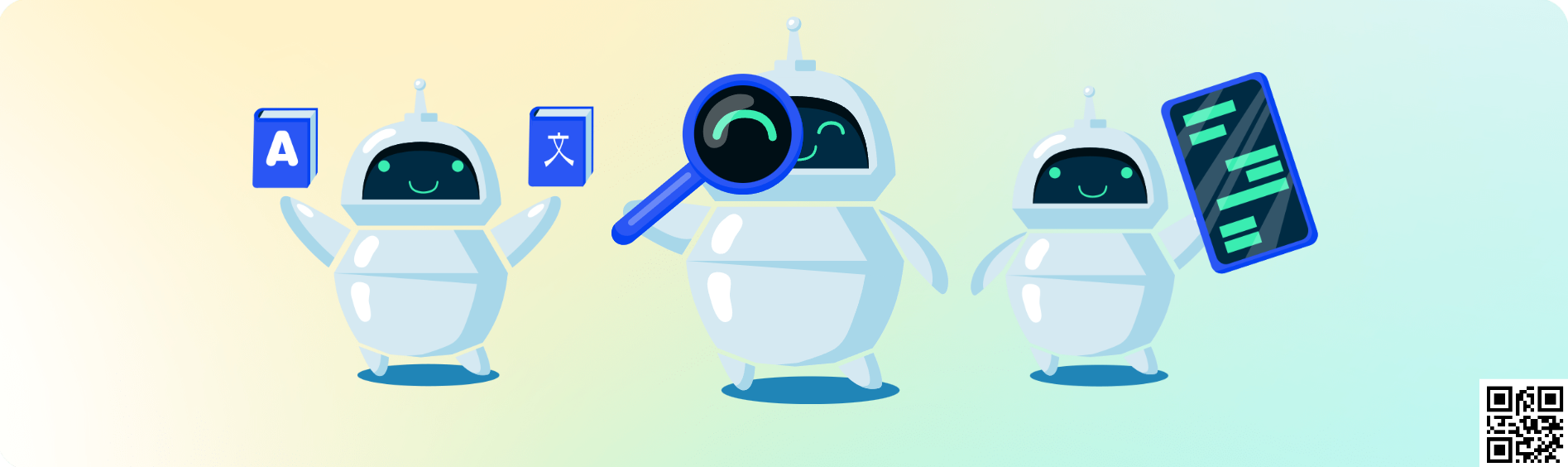
⚙️ Prerequisites
- A working proxy
- Node.JS installed
- Capsolver API key
🤖 Step 1: Install Necessary Packages
Execute the following commands to install the required packages:
npm install axios
npm install url
👨💻 Node.JS Code for solve cloudflare challenge (5s) / cf_clearance
Here's a Node.JS sample script to accomplish the task:
const axios = require('axios');
const url = require('url');
// Change these values
const PARSED_PROXY = 'http://username:password@ip:port';
const capsolverApiKey = 'YourAPIKEY';
const PAGE_URL = 'site';
async function createTask(payload) {
try {
const res = await axios.post('https://api.capsolver.com/createTask', {
clientKey: capsolverApiKey,
task: payload
});
console.log(res.data)
return res.data;
} catch (error) {
console.error(error);
}
}
async function getTaskResult(taskId) {
try {
success = false;
while(success == false){
await sleep(1000);
console.log("Getting task result for task ID: " + taskId);
const res = await axios.post('https://api.capsolver.com/getTaskResult', {
clientKey: capsolverApiKey,
taskId: taskId
});
if( res.data.status == "ready") {
success = true;
console.log(res.data)
return res.data;
}
}
} catch (error) {
console.error(error);
return null;
}
}
async function solveCloudflare(pageURL, proxy) {
const taskPayload = {
type: 'AntiCloudflareTask',
websiteURL: pageURL,
proxy: proxy,
};
const taskData = await createTask(taskPayload);
return await getTaskResult(taskData.taskId);
}
function sleep(ms) {
return new Promise(resolve => setTimeout(resolve, ms));
}
async function main() {
console.log('Solving CF');
const solution = await solveCloudflare(PAGE_URL, PARSED_PROXY );
console.log('Solution:', solution);
const parsedUrl = url.parse(PAGE_URL);
const domain = parsedUrl.hostname;
const formattedDomain = `.${domain}`;
axiosInstance.defaults.headers['Cookie'] = `cf_clearance=${solution.token}`;
// console.log(response.data);
}
main().catch((err) => {
console.error(err);
});
⚠️ Change these variables
- PARSED_PROXY: Update with your proxy details. The format should be http://username:password@ip:port.
- capsolverApiKey: Obtain your API key from the Capsolver Dashboard.
- PAGE_URL: Replace with the URL of the website for which you wish to solve cloudflare challenge